Define a class named Complex that will represent complex numbers. A complex number is a number of the form a+b*i, where, for our purposes, a and b are integers and i represents sqrt(-1). In your class, include a constructor that takes two parameters and initializes a and b to the first and second parameter respectively, and include a constructor that takes one parameter and initializes a to the value of the parameter and b to 0. Also include a default constructor that initializes both a and b to 0. Overload all of the followind operators so that they correctly apply to the class Complex: ==, +, -, *, >>, and <<. Using your Complex class, write a program that would let the user input two complex numbers, and then print out their sum, difference, product, and whether they are equal to one another.
Define a class named Complex that will represent complex numbers. A
complex number is a number of the form
a+b*i,
where, for our purposes, a and b are integers and i represents sqrt(-1).
In your class, include a constructor that takes two parameters and
initializes a and b to the first and second parameter respectively, and include
a constructor that takes one parameter and initializes a to the value of the
parameter and b to 0. Also include a default constructor that initializes both a
and b to 0. Overload all of the followind operators so that they correctly apply
to the class Complex: ==, +, -, *, >>, and <<.
Using your Complex class, write a program that would let the user input two
complex numbers, and then print out their sum, difference, product, and whether
they are equal to one another.

#include<bits/stdc++.h>
using namespace std;
class Complex {
public:
int real, imaginary;
Complex()
{
}
Complex(int tempReal, int tempImaginary)
{
real = tempReal;
imaginary = tempImaginary;
}
Complex addComp(Complex C1, Complex C2)
{
Complex temp;
temp.real = C1.real + C2.real;
temp.imaginary = C1.imaginary + C2.imaginary;
return temp;
}
Complex subComp(Complex C1, Complex C2)
{
Complex temp;
temp.real = C1.real - C2.real;
temp.imaginary = C1.imaginary - C2.imaginary;
return temp;
}
Complex multiComp(Complex C1, Complex C2)
{
Complex temp;
temp.real = C1.real * C2.real;
temp.imaginary = C1.imaginary * C2.imaginary;
return temp;
}
Complex compareComp(Complex C1, Complex C2)
{
if(sqrt((C1.real)^2 + (C1.imaginary)^2)-sqrt((C2.real)^2 + (C2.imaginary)^2)>0)
cout<<"first number is larger "<<endl;
else if(sqrt((C1.real)^2 + (C1.imaginary)^2)-sqrt((C2.real)^2 + (C2.imaginary)^2)<0)
cout<<"first number is larger "<<endl;
else
cout<<"both are eual "<<endl;
}
};
int main()
{
int c;
Complex c1,c2,c3;
cout<<"Enter the real part of first number "<<endl;
cin>>c1.real;
cout<<"Enter the imaginary part of first number "<<endl;
cin>>c1.imaginary;
cout<<"Enter the real part of second number "<<endl;
cin>>c2.real;
cout<<"Enter the imaginary part of second number "<<endl;
cin>>c2.imaginary;
do{
cout<<endl<<"1 for add"<<endl<<"2 for subtract"<<endl<<"3 for multipply"<<endl<<"4 for compare"<<endl<<"5 for exit"<<endl;
cin>>c;
switch(c){
case 1:
c3=c3.addComp(c1,c2);
cout<<"Sum of complex number : "
<< c3.real << " + i"
<< c3.imaginary;
break;
case 2:
c3=c3.subComp(c1,c2);
cout<<"Subtract of complex number : "
<< c3.real << " + i("
<< c3.imaginary<<")";
break;
case 3:
c3=c3.multiComp(c1,c2);
cout<<"multiplication of complex number : "
<< c3.real << " + i"
<< c3.imaginary;
break;
case 4:
c3=c3.compareComp(c1,c2);
break;
case 5:
exit(1);
default:
cout<<"Enter valid input"<<endl;
break;
}
}while(1);
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

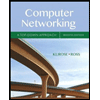
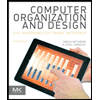
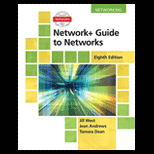
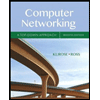
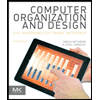
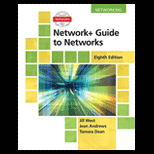
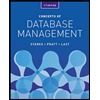
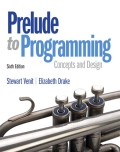
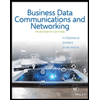