Design a class named Triangle that extends GeometricObject: import java.util.Scanner; abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } @Override public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); } The Triangle class contains: Three double data fields named side1, side2, and side3 A default constructor that creates a triangle with three sides of length 1.0 A constructor that creates a triangle with specified values for side1, side2, and side3 Accessor methods for all three data fields A method called getArea() that returns the area of a triangle A method named getPerimeter() that returns the perimeter of the triangle A method named toString() that returns the string description of the triangle in the following format: "Triangle: side1 = " + side1 + " side2 = " + side2 + " side3 = " + side3;
Design a class named Triangle that extends GeometricObject:
import java.util.Scanner;
abstract class GeometricObject {
private String color = "white";
private boolean filled;
private java.util.Date dateCreated;
/** Construct a default geometric object */
protected GeometricObject() {
}
/** Construct a geometric object with color and filled value */
protected GeometricObject(String color, boolean filled) {
dateCreated = new java.util.Date();
this.color = color;
this.filled = filled;
}
/** Return color */
public String getColor() {
return color;
}
/** Set a new color */
public void setColor(String color) {
this.color = color;
}
/** Return filled. Since filled is boolean,
* the get method is named isFilled */
public boolean isFilled() {
return filled;
}
/** Set a new filled */
public void setFilled(boolean filled) {
this.filled = filled;
}
/** Get dateCreated */
public java.util.Date getDateCreated() {
return dateCreated;
}
@Override
public String toString() {
return "created on " + dateCreated + "\ncolor: " + color +
" and filled: " + filled;
}
/** Abstract method getArea */
public abstract double getArea();
/** Abstract method getPerimeter */
public abstract double getPerimeter();
}
The Triangle class contains:
- Three double data fields named side1, side2, and side3
- A default constructor that creates a triangle with three sides of length 1.0
- A constructor that creates a triangle with specified values for side1, side2, and side3
- Accessor methods for all three data fields
- A method called getArea() that returns the area of a triangle
- A method named getPerimeter() that returns the perimeter of the triangle
- A method named toString() that returns the string description of the triangle in the following format: "Triangle: side1 = " + side1 + " side2 = " + side2 + " side3 = " + side3;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

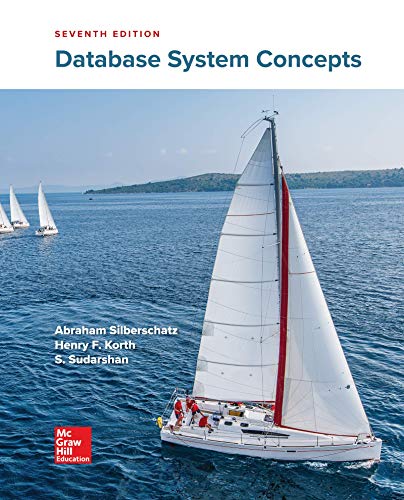
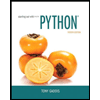
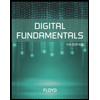
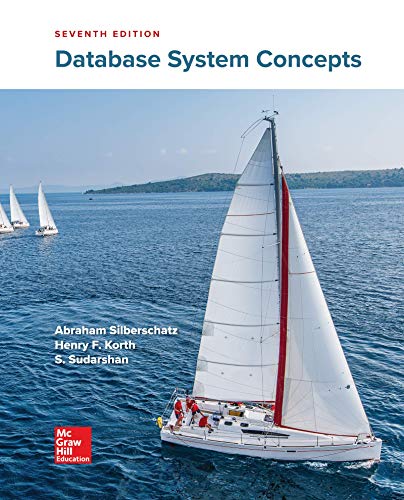
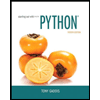
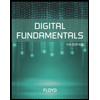
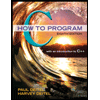
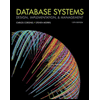
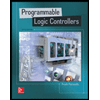