Do a program that displays a simulated writing check.Use supplied Check.java and CheckDemo.java. It should then display a simulated check with the dollar amount spelled out,as shown. Check.Demo package checks; import java.time.LocalDate; public class Check { private double amount; private String payee; private LocalDate date; /* * Constructor */ public Check(double amount, String payee, LocalDate date) { // Your code here } public Check(Check original) { // Your code here } /* * Returns a String representing the check */ public String toString() { String result = "\t\t\t\tDate:\t"; result += date.getMonthValue() + "/"; result += date.getDayOfMonth() + "/"; result += date.getYear() + "\n\n"; result += "Pay to the Order of: " + payee + "\t\t"; result += "$" + amount + "\n\n"; result += amountText() + "\n"; return result; // return amountText(); } private String amountText() { String result = ""; // Your code here return result; } /* * Mutators */ public void setAmount(double amount) { // Your code here } public void setPayee(String payee) { // Your code hree } public void setDate(LocalDate date) { // Your code here } /* * Accessors */ public double getAmount() { // Your code here } public String getPayee() { // Your code here } public LocalDate getDate() { // Your code here } } CheckDemo.java package check; import java.time.LocalDate; public class CheckDemo { public static void main(String[] args) { Check check = new Check(1915.85, "Walmart", LocalDate.of(2021, 11, 24)); System.out.println(check); check = new Check(15.25, "McDonald", LocalDate.of(2021, 11, 24)); System.out.println(check); check = new Check(999.00, "Rent", LocalDate.of(2021, 11, 24)); System.out.println(check); check = new Check(6000.01, "Trip", LocalDate.of(2021, 11, 24)); System.out.println(check); check = new Check(16518.01, "Car", LocalDate.of(2021, 11, 24)); System.out.println(check); check = new Check(25000.99, "Tuition", LocalDate.of(2021, 11, 24)); System.out.println(check); // for (int dollar = 0; dollar < 10000; dollar++) { // check.setAmount(dollar + (Math.random() * 100) / 100.0); // System.out.println(check); // } } }
Do a program that displays a simulated writing check.Use supplied Check.java and CheckDemo.java.
It should then display a simulated check with the dollar amount spelled out,as shown.
Check.Demo
package checks;
import java.time.LocalDate;
public class Check {
private double amount;
private String payee;
private LocalDate date;
/*
* Constructor
*/
public Check(double amount, String payee, LocalDate date) {
// Your code here
}
public Check(Check original) {
// Your code here
}
/*
* Returns a String representing the check
*/
public String toString() {
String result = "\t\t\t\tDate:\t";
result += date.getMonthValue() + "/";
result += date.getDayOfMonth() + "/";
result += date.getYear() + "\n\n";
result += "Pay to the Order of: " + payee + "\t\t";
result += "$" + amount + "\n\n";
result += amountText() + "\n";
return result;
// return amountText();
}
private String amountText() {
String result = "";
// Your code here
return result;
}
/*
* Mutators
*/
public void setAmount(double amount) {
// Your code here
}
public void setPayee(String payee) {
// Your code hree
}
public void setDate(LocalDate date) {
// Your code here
}
/*
* Accessors
*/
public double getAmount() {
// Your code here
}
public String getPayee() {
// Your code here
}
public LocalDate getDate() {
// Your code here
}
}
CheckDemo.java
package check;
import java.time.LocalDate;
public class CheckDemo {
public static void main(String[] args) {
Check check = new Check(1915.85, "Walmart", LocalDate.of(2021, 11, 24));
System.out.println(check);
check = new Check(15.25, "McDonald", LocalDate.of(2021, 11, 24));
System.out.println(check);
check = new Check(999.00, "Rent", LocalDate.of(2021, 11, 24));
System.out.println(check);
check = new Check(6000.01, "Trip", LocalDate.of(2021, 11, 24));
System.out.println(check);
check = new Check(16518.01, "Car", LocalDate.of(2021, 11, 24));
System.out.println(check);
check = new Check(25000.99, "Tuition", LocalDate.of(2021, 11, 24));
System.out.println(check);
// for (int dollar = 0; dollar < 10000; dollar++) {
// check.setAmount(dollar + (Math.random() * 100) / 100.0);
// System.out.println(check);
// }
}
}
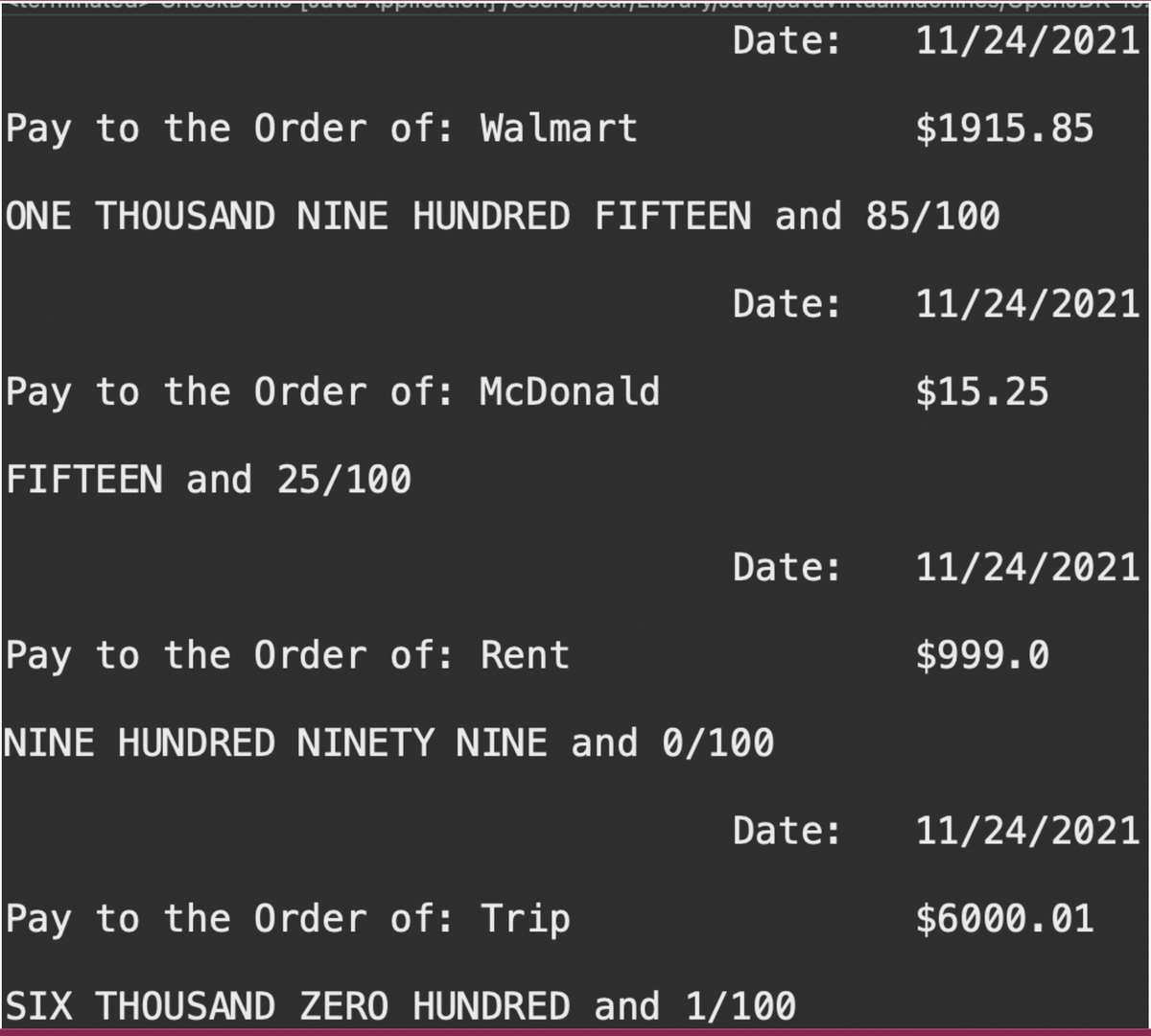
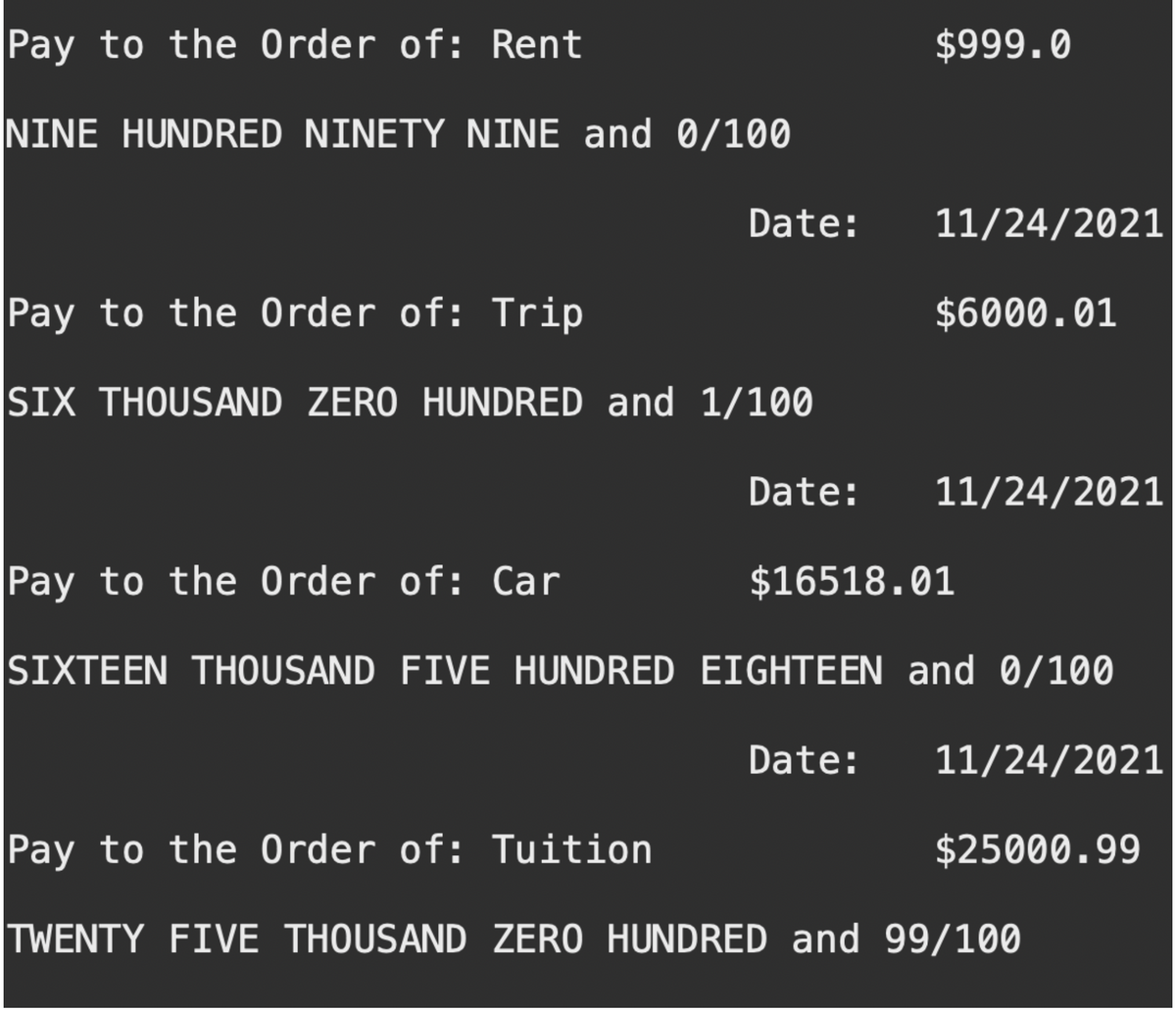

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

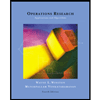
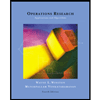