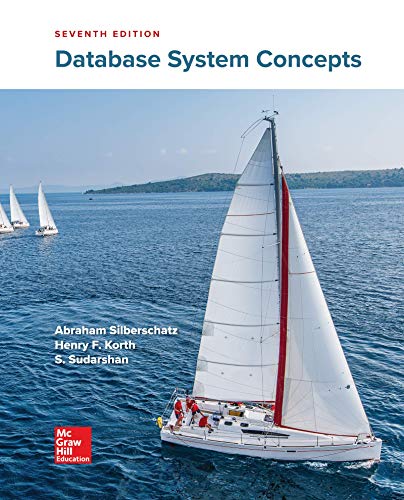
In java, implement a simple email messaging system. a message has a recipient, a sender, and a message text. a mailbox can store messages. supply a number of mailboxes for different users and a user interface for the user to login, send messages to other users, read their own messages, and log out.
Given the code I have so far, what needs to be added?
class Message {
private String sender;
private String recipient;
private String messageText;
public Message(String sender, String recipient) {
this.sender = sender;
this.recipient = recipient;
this.messageText = " ";
}
public void append(String text) {
this.messageText += text;
}
public String toString() {
return "From: " + sender + " To: " + recipient + "\n" + this.messageText;
}
}
class Mailbox {
private ArrayList<Message> email;
public Mailbox() {
this.email = new ArrayList<Message>();
}
public Message getMessage(int i) {
return this.email.get(i);
}
public void addMessage(Message m) {
this.email.add(m);
}
public void removeMessage(int i) {
this.email.remove(i);
}
public int mailBoxSize() {
return this.email.size();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- In Java import java.util.Scanner;public class Image {int numberOfPhotos; // photos on rolldouble fStop; // light let it 1.4,2.0,2.8 ... 16.0int iso; // sensativity to light 100,200, 600int filterNumber; // 1-6String subjectMatter;String color; // black and white or colorString location;boolean isblurry;public String looksBlurry(boolean key){if ( key == true){return "Photo is Blurry";}else{return "Photo is Clear";}}public void printPhotoDetails (String s1){Scanner br= new Scanner(System.in);String subjectMatter=s1;System.out.println("Data of Nature photos:");System.out.println("Enter number of photos:");numberOfPhotos= br.nextInt();int i=1;while(true){System.out.println("Enter Filter number of photos"+i+":");filterNumber= br.nextInt();System.out.println("Enter colour of photo"+i+ ":");String color= br.next();System.out.println("Enter focal length of photo"+i+":");fStop= br.nextInt();System.out.println("Enter location of photo"+i+":");String location= br.next();System.out.println("Enter…arrow_forwardWrite a java class method named capitalizeWords that takes one parameter of type String. The method should print its parameter, but with the first letter of each word capitalized. For example, the call capitalizeWords ("abstract window toolkit"); will produce the following output: Abstract Window Toolkitarrow_forwardjava Write an interface for Animal. We will say that every Animal will make a sound and will also be able to give its type.arrow_forward
- Java. Please use the template in the picture.arrow_forwardImplement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forwardPlease solve this using java and attach output screenshot and implementation screenshot. Using this clock class please: public class Clock { private int hr; private int min; private int sec; public Clock() { setTime(0, 0, 0); } public Clock(int hours, int minutes, int seconds) { setTime(hours, minutes, seconds); } public void setTime(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; if(0 <= seconds && seconds < 60) sec = seconds; else sec = 0; } public int getHours() { return hr; } public int getMinutes() { return min; } public int getSeconds() { return sec; } public void printTime() { if (hr < 10) System.out.print("0"); System.out.print(hr + ":"); if (min < 10) System.out.print("0"); System.out.print(min + ":"); if (sec < 10) System.out.print("0"); System.out.print(sec); } public void incrementSeconds() { sec++; if (sec >…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
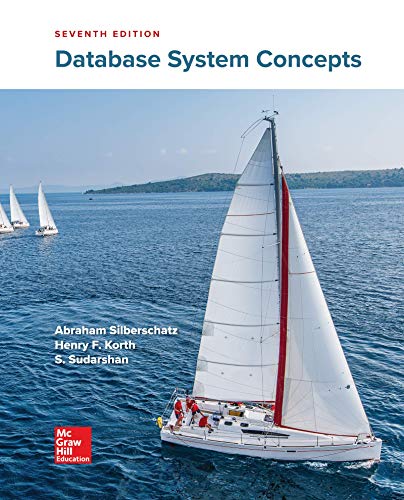
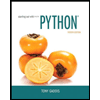
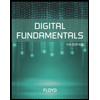
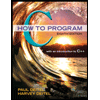
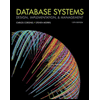
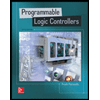