Drawing a POINT Problem on OpenGL Java: (…..imports as before) public class Code extends JFrame implements GLEventListener { private int renderingProgram; private int vao[ ] = new int[1]; public Code() { (…..constructor as before) } public void display(GLAutoDrawable drawable) { GL4 gl = (GL4) GLContext.getCurrentGL(); gl.glUseProgram(renderingProgram); gl.glDrawArrays(GL_POINTS, 0, 1); } public void init(GLAutoDrawable drawable) { GL4 gl = (GL4) GLContext.getCurrentGL(); renderingProgram = createShaderProgram(); gl.glGenVertexArrays(vao.length, vao, 0); gl.glBindVertexArray(vao[0]); } private int createShaderProgram() { GL4 gl = (GL4) GLContext.getCurrentGL(); String vshaderSource[ ] = { "#version 430 \n", "void main(void) \n", "{ gl_Position = vec4(0.0, 0.0, 0.0, 1.0); } \n", }; String fshaderSource[ ] = { "#version 430 \n", "out vec4 color; \n", "void main(void) \n", "{ color = vec4(0.0, 0.0, 1.0, 1.0); } \n", }; int vShader = gl.glCreateShader(GL_VERTEX_SHADER); gl.glShaderSource(vShader, 3, vshaderSource, null, 0); // 3 is the count of lines of source code gl.glCompileShader(vShader); int fShader=gl.glCreateShader(GL_FRAGMENT_SHADER); gl.glShaderSource(fShader, 4, fshaderSource, null, 0); // 4 is the count of lines of source code gl.glCompileShader(fShader); int vfProgram = gl.glCreateProgram(); gl.glAttachShader(vfProgram, vShader); gl.glAttachShader(vfProgram, fShader); gl.glLinkProgram(vfProgram); gl.glDeleteShader(vShader); gl.glDeleteShader(fShader); return vfProgram; } . . . main(), reshape(), and dispose() as before /* fragshader coding */ #version 430 out vec4 color; void main(void) { if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0); else if(gl_FragCoord.x > 295 && gl_FragCoord.x < 310) color = vec4(1.0, 0.0, 1.0, 1.0); else color = vec4(0.0, 0.0, 1.0, 1.0); } /* vertshader coding */ #version 430 uniform float offset; void main(void) { if (gl_VertexID == 0) gl_Position = vec4( 0.25 + offset, -0.25, 0.0, 1.0); else if (gl_VertexID == 1) gl_Position = vec4(-0.25 + offset, -0.25, 0.0, 1.0); else gl_Position = vec4( 0.25 + offset, 0.25, 0.0, 1.0); } How answer below question with explanations & picture: 1. Modify above Java OpenGL program to add animation that causes the drawn point to grow and shrink, in a cycle. Hint: use the glPointSize() function, with a variable as the parameter? 2. Modify above Java OpenGL program so that the drawn rectangle (not point) will grow and shrink in a cycle (try to move the rectangle in x-axis)?
Drawing a POINT Problem on OpenGL Java:
(…..imports as before)
public class Code extends JFrame implements GLEventListener
{ private int renderingProgram;
private int vao[ ] = new int[1];
public Code() { (…..constructor as before) }
public void display(GLAutoDrawable drawable)
{ GL4 gl = (GL4) GLContext.getCurrentGL();
gl.glUseProgram(renderingProgram);
gl.glDrawArrays(GL_POINTS, 0, 1);
}
public void init(GLAutoDrawable drawable)
{ GL4 gl = (GL4) GLContext.getCurrentGL();
renderingProgram = createShaderProgram();
gl.glGenVertexArrays(vao.length, vao, 0);
gl.glBindVertexArray(vao[0]);
}
private int createShaderProgram()
{ GL4 gl = (GL4) GLContext.getCurrentGL();
String vshaderSource[ ] =
{ "#version 430 \n",
"void main(void) \n",
"{ gl_Position = vec4(0.0, 0.0, 0.0, 1.0); } \n",
};
String fshaderSource[ ] =
{ "#version 430 \n",
"out vec4 color; \n",
"void main(void) \n",
"{ color = vec4(0.0, 0.0, 1.0, 1.0); } \n",
};
int vShader = gl.glCreateShader(GL_VERTEX_SHADER);
gl.glShaderSource(vShader, 3, vshaderSource, null, 0); // 3 is the count of lines of source code
gl.glCompileShader(vShader);
int fShader=gl.glCreateShader(GL_FRAGMENT_SHADER);
gl.glShaderSource(fShader, 4, fshaderSource, null, 0); // 4 is the count of lines of source code
gl.glCompileShader(fShader);
int vfProgram = gl.glCreateProgram();
gl.glAttachShader(vfProgram, vShader);
gl.glAttachShader(vfProgram, fShader);
gl.glLinkProgram(vfProgram);
gl.glDeleteShader(vShader);
gl.glDeleteShader(fShader);
return vfProgram;
}
. . . main(), reshape(), and dispose() as before
/* fragshader coding */
#version 430
out vec4 color;
void main(void)
{
if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0);
else if(gl_FragCoord.x > 295 && gl_FragCoord.x < 310) color = vec4(1.0, 0.0, 1.0, 1.0);
else color = vec4(0.0, 0.0, 1.0, 1.0);
}
/* vertshader coding */
#version 430
uniform float offset;
void main(void)
{
if (gl_VertexID == 0) gl_Position = vec4( 0.25 + offset, -0.25, 0.0, 1.0);
else if (gl_VertexID == 1) gl_Position = vec4(-0.25 + offset, -0.25, 0.0, 1.0);
else gl_Position = vec4( 0.25 + offset, 0.25, 0.0, 1.0);
}
How answer below question with explanations & picture:
1. Modify above Java OpenGL
2. Modify above Java OpenGL program so that the drawn rectangle (not point) will grow and shrink in a cycle (try to move the rectangle in x-axis)?

Step by step
Solved in 4 steps

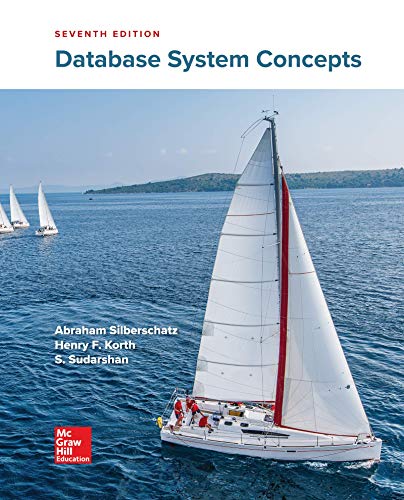
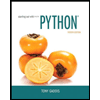
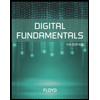
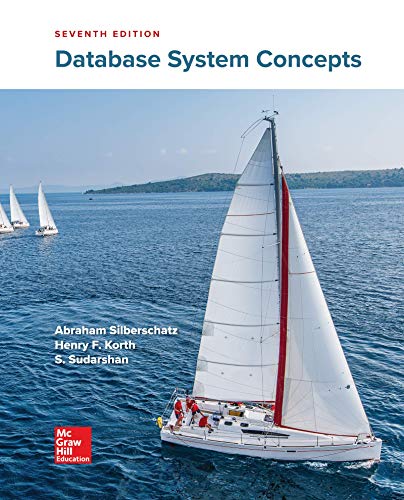
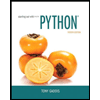
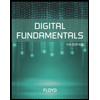
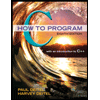
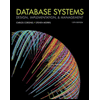
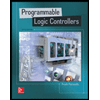