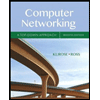

Source code for DrawGraphics.java:
import java.awt.Color;
import java.awt.Graphics;
import java.util.ArrayList;
public class DrawGraphics
{
ArrayList<BouncingBox> boxes;
/** Initializes this class for drawing. */
public DrawGraphics()
{
boxes = new ArrayList<BouncingBox>();
boxes.add(new BouncingBox(200, 50, Color.GREEN));
boxes.get(0).setMovementVector(1,3);
boxes.add(new BouncingBox(100, 100, Color.BLUE));
boxes.get(1).setMovementVector(3,5);
boxes.add(new BouncingBox(50,50, Color.CYAN));
boxes.get(2).setMovementVector(6, 6);
}
/** Draw the contents of the window on surface. Called 20 times per second. */
public void draw(Graphics surface)
{
surface.drawLine(50, 50, 250, 250);
surface.drawOval(20, 20, 50, 50);
surface.drawRect(20, 100, 15, 60);
surface.drawArc(100, 30, 40, 40, 20, 30);
for(int i = 0; i < boxes.size(); i = i + 1)
{
boxes.get(i).draw(surface);
}
}
}
Source code for SimpleDraw.java:
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
/** Displays a window and delegates drawing to DrawGraphics. */
public class SimpleDraw extends JPanel implements Runnable {
private static final long serialVersionUID = -7469734580960165754L;
private boolean animate = true;
private final int FRAME_DELAY = 50; // 50 ms = 20 FPS
public static final int WIDTH = 300;
public static final int HEIGHT = 300;
private DrawGraphics draw;
public SimpleDraw(DrawGraphics drawer) {
this.draw = drawer;
}
/** Paint callback from Swing. Draw graphics using g. */
public void paintComponent(Graphics g) {
super.paintComponent(g);
// Enable anti-aliasing for better looking graphics
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
draw.draw(g2);
}
/** Enables periodic repaint calls. */
public synchronized void start() {
animate = true;
}
/** Pauses animation. */
public synchronized void stop() {
animate = false;
}
private synchronized boolean animationEnabled() {
return animate;
}
public void run() {
while (true) {
if (animationEnabled()) {
repaint();
}
try {
Thread.sleep(FRAME_DELAY);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
public static void main(String args[]) {
final SimpleDraw content = new SimpleDraw(new DrawGraphics());
JFrame frame = new JFrame("Graphics!");
Color bgColor = Color.white;
frame.setBackground(bgColor);
content.setBackground(bgColor);
// content.setSize(WIDTH, HEIGHT);
// content.setMinimumSize(new Dimension(WIDTH, HEIGHT));
content.setPreferredSize(new Dimension(WIDTH, HEIGHT));
// frame.setSize(WIDTH, HEIGHT);
frame.setContentPane(content);
frame.setResizable(false);
frame.pack();
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) { System.exit(0); }
public void windowDeiconified(WindowEvent e) { content.start(); }
public void windowIconified(WindowEvent e) { content.stop(); }
});
new Thread(content).start();
frame.setVisible(true);
}
}
Source code for BouncingBox.java:
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
public class BouncingBox {
int x;
int y;
Color color;
int xDirection = 0;
int yDirection = 0;
final int SIZE = 20;
/**
* Initialize a new box with its center located at (startX, startY), filled
* with startColor.
*/
public BouncingBox(int startX, int startY, Color startColor) {
x = startX;
y = startY;
color = startColor;
}
/** Draws the box at its current position on to surface. */
public void draw(Graphics surface) {
// Draw the object
surface.setColor(color);
surface.fillRect(x - SIZE/2, y - SIZE/2, SIZE, SIZE);
surface.setColor(Color.BLACK);
((Graphics2D) surface).setStroke(new BasicStroke(3.0f));
surface.drawRect(x - SIZE/2, y - SIZE/2, SIZE, SIZE);
// Move the center of the object each time we draw it
x += xDirection;
y += yDirection;
// If we have hit the edge and are moving in the wrong direction, reverse direction
// We check the direction because if a box is placed near the wall, we would get "stuck"
// rather than moving in the right direction
if ((x - SIZE/2 <= 0 && xDirection < 0) ||
(x + SIZE/2 >= SimpleDraw.WIDTH && xDirection > 0)) {
xDirection = -xDirection;
}
if ((y - SIZE/2 <= 0 && yDirection < 0) ||
(y + SIZE/2 >= SimpleDraw.HEIGHT && yDirection > 0)) {
yDirection = -yDirection;
}
}
public void setMovementVector(int xIncrement, int yIncrement) {
xDirection = xIncrement;
yDirection = yIncrement;
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Create the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forwardimport java.util.Scanner; public class AverageWithSentinel{ public static final int END_OF_INPUT = -500; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Declare an int variable with an initial value // as the count of input integers // Step 3: Declare a double variable with an initial value // as the total of all input integers // Step 4: Display an input prompt // "Enter an integer, -500 to stop: " // Step 5: Read an integer and store it in an int variable // Step 6: Use a while loop to update count and total as long as // the input value is not -500. // Then display the same prompt and read the next integer // Step 7: If count is zero // Display the following message // "No integers were…arrow_forwardWrite a java program to complete the following parts: Part-1: Create a class Car and perform the following: - Declare public instance variables (name, model, color) as (String, int, and String) respectively. - Define set() method to set a car's name, model, and color. Define show() method to print out the car's name, model, and color. Part-2: In the main method: Declare an array of the defined class Car with length 5. Create three objects from Car and add them into the array. Set the objects' values (name, model, color) using set method. Print out the object's values (name, model, color) using show method.arrow_forward
- import java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forwardGiven string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forward
- The first one is answered as follows. Help with the second one. package test;import javax.swing.*;import java.awt.*;import java.awt.event.*; public class JavaApplication1 { public static void main(String[] arguments) { JFrame.setDefaultLookAndFeelDecorated(true);JFrame frame = new JFrame("print X and Y Coordinates");frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout());frame.setSize(500,400); final JTextField output = new JTextField();;frame.add(output,BorderLayout.SOUTH); frame.addMouseListener(new MouseListener() {public void mousePressed(MouseEvent me) { }public void mouseReleased(MouseEvent me) { }public void mouseEntered(MouseEvent me) { }public void mouseExited(MouseEvent me) { }public void mouseClicked(MouseEvent me) {int x = me.getX();int y = me.getY();output.setText("Coordinate X:" + x + "|| Coordinate Y:" + y);}}); frame.setVisible(true);}}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardJavaarrow_forward
- Java. Starter code: import java.util.*;public class PoD {public static boolean validateBoard(String[][] gameBoard) {boolean validBoard;//PLEASE START YOUR WORK HERE//PLEASE END YOUR WORK HEREreturn (validBoard);}public static void main(String[] args) {//Instantiate new scanner to read from the console.Scanner in = new Scanner( System.in );int gamePiece;String[][] ticTacToeBoard = new String[3][3];for (int i=0; i<3; i++){for (int j=0; j<3; j++){//Read in boardticTacToeBoard[i][j] = in.next();//Output boardif (j<2){System.out.print(ticTacToeBoard[i][j] + " ");}else{System.out.println(ticTacToeBoard[i][j]);}}}if (validateBoard(ticTacToeBoard)) {System.out.println("Valid board");}else {System.out.println("Invalid board");}}}arrow_forwardUse the Java program in below, implement encapsulation on the property name, and use getters and setters to unhide the name: class Animal1{String name; public void eat(){System.out.println("I can eat");}} class Dog extends Animal1{public void display(){System.out.println("My name is " + name);}} public class Lab06_A{public static void main(String[] args){ Dog labrador = new Dog(); labrador.name = "Rohu";labrador.display(); labrador.eat();} }arrow_forwardPRACTICE CODE import java.util.TimerTask;import org.firmata4j.ssd1306.MonochromeCanvas;import org.firmata4j.ssd1306.SSD1306;public class CountTask extends TimerTask {private int countValue = 10;private final SSD1306 theOledObject;public CountTask(SSD1306 aDisplayObject) {theOledObject = aDisplayObject;}@Overridepublic void run() {for (int j = 0; j <= 3; j++) {theOledObject.getCanvas().clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "Hello");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}theOledObject.clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "My name is ");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}while (true) {for (int i = 10; i >= 0; i--)…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
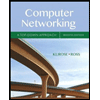
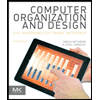
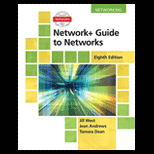
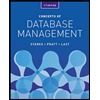
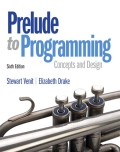
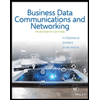