Q: x-123.456 a-123 if x//a x//a> (a>0): print (x//1) else: print ("ok")
A: The given code is: x = 123.456a = 123 if x//a > (a>0): print(x//1) else:…
Q: USE PYTHON Source: en.wikipedia.org/wiki/Camel_case In some languages, it’s common to use…
A: error 404
Q: The following program first reads an integer number 'number' (1/2 2/2 3/2+...+ number/2) and stores…
A: SOLUTION
Q: a. Provide a 2-3 paragraph summary of the data retention and privacy policies. Be sure to look for…
A: The above question is solved in step 2 :-
Q: Hello. The goal of the experiment was to find the largest adder that will fit into a GAL22V10.…
A: The largest adder that will fit into a GAL22V10 is a 4-bit ripple carry adder.
Q: Write the following SQL Statements: 1. Write a SELECT statement that returns one row for each…
A: 1.
Q: Think about when Huffman’s algorithm does reduce required bit transmission. Use Huffman’s algorithm…
A: Explanation: Huffman Algorithm A lossless data compression algorithm is Huffman coding. Input…
Q: without truth table. show that ¬(¬p V q) ^ ¬p is a contradiction.
A: These question answer is as follows,
Q: In Quick Sort, the function Partition is used to arranged the values into the S1 set and S2 set…
A: Select a pivot. Split: Splits the task set, moving smaller pieces to the left of the pivot and…
Q: "Include the header file fstream in the program.2.Declare file stream variables.3.Associate the file…
A: For the given problem, below is the C++ code.
Q: The only way to get both higher precision and range is to increase the number of bits. True False
A: According to the information given:- We have to define the mentioned statement is true or false.
Q: A student is running a Python program like this: >python mergefasta.py myfile1.fa myfile2.fa In the…
A: Answer: In python, when we pass command line arguments, the 0th argument is the program name itself.…
Q: those are the wrong values.
A: We need to find the correct hexadecimal codes for the given mips statements.
Q: Many data centers that support cloud computing now pipe chilled water through the server racks to…
A: Which of the following is FALSE? Virginia's "data center alley," is the site of 70 percent of that…
Q: Explain types of Evaluation.
A:
Q: (d) Find Ce (e) Find A UBUC
A: Solution for Question 4 Complement of a set It is a set which include all the elements from the…
Q: Over the past decades, advances in technology for data storage has rapidly evolved from paper tape…
A: Punch cards, floppy disks, tape, hard drives, and flash technology are just a few of the many…
Q: What is hierarchical task analysis? Explain with example.
A: In this question we need to explain the Hierarchical Task Analysis (HTA) with proper example.
Q: The following incomplete code computes the sum of the first 10 numbers (1,..., 10): i=1 sum-sum+i;…
A: Below I have provided a program for the given question.
Q: How can we organize an international regulatory entity that has the power, knowledge, and neutrality…
A: The above question is solved in step 2 :-
Q: 3. The number of ways of arranging r objects chosen from n, where order is important (these are…
A: In this question we need to implement a C program which takes value of r and n as input from user…
Q: Define the following sequence recursively, giving two instructions in mathematical language. {2, 6,…
A: The recursively sequence defined as follows:given the two instructions in mathematical language. =…
Q: a. A main method asks the user to provide the number of rows and columns for a 2-dimensional array…
A: error 404
Q: www.m Greeting!
A:
Q: ou have been asked to work as a usability consultant for a company where the development team has…
A: A Usability authority may be a person that analyses the planning and overall application or product…
Q: rove that every tree with at least two vertices is bipartit
A: Dear Student, A bipartite graph is a graph whose vertices can be divided into two sets X and Y such…
Q: Need to fill the blanks. #include #include #include // this library is needed to use exit(0) //…
A: Fill in the blanks. Note: The solution provided is in bold.
Q: backward. That is,, if the argument is 1234, it outputs the following to the screen: 4321.
A: #include <iostream> using namespace std; void reverse(int n) //function reverse{ if (n…
Q: List the factors affecting human computer interface.
A: Human computer interaction can de defined as the study of how persons deal or interact with the…
Q: In Python, a fu
A: Dear Student, In Python, a function can only return one value.
Q: Can someone pretty please explain what nchar and nvarchar datatypes are compared to regular char and…
A: char and nchar are fixed-length which will reserve storage space for number of characters you…
Q: eight is good na physical developme of a baby s growth. The below chart shows weights in the 50th…
A: error 404
Q: WorksOn Database: Employee (EmployeeNumber, EmployeeName, BirthDate, Title, Salary,…
A: Dear Student, SQL query for each question is given below and the queries are self explanatory.
Q: 22 23 24 25 26 P 1 KB 2 KB A KR VPN bits Number of VPO bits PPN bits PPO bit
A: The answer is
Q: 4. Alia wrote the code shown for a character in her animation. After running the code once, how many…
A: The solution is given below with explanation Happy to help you ?
Q: lease assist me with this lab: 2.17 LAB: Network Automation (modules) In network administration,…
A: The complete answer is below:
Q: Exercise 3: Multi-way Trees A way to reduce the height of tree and ensure balance is to allow…
A: Solution- In the given solution , the B - tree insert one by one elements with maximum keys of 4 and…
Q: (c) WITH RESPECT TO THE VECTOR SPACE MODEL (VSM): (i) Specify a data structure that might be…
A: Vector space model or term vector model is an algebraic model for representing text documents as…
Q: B: Artwork label (modules) Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921…
A: error 404
Q: What are the specific pros and cons of single sourcing cloud services on a single cloud provider?…
A: I have given answer below.
Q: 0-12. Multiple Exceptions: Add to the following code such that no unhandled exceptions occur. Look…
A: To handle all the exception, provided python code with proper exception handing and output below.
Q: LOAD reg4, [reg3] ;fetch current list element
A: The answer is
Q: In python. Please include docstring, and follow the code style requirements below. Thanks. Write a…
A: Write a recursive function named list_max that takes as its parameter a list of numbers and returns…
Q: universal set subset empty set set element
A: I have given definition of all the terms with example in details below.
Q: What is task migratability?
A: Please find the answer in the following steps.
Q: using c++, Write a function method that determines the mean of all the values in an array of…
A: To find the mean of the all values in array of integers, function with proper code in c++ and output…
Q: I need help updating function makeMove to do the following in C Programming - Return type void -…
A: We need to modify the given code such that if fulfills given requirements. Given code: void…
Q: 35. What are the causes of overheating of microprocessor?
A:
Q: What is the idea behind distributed query processing using the semijoin operation? Write your answer…
A: Answer:
Q: w do you feel about file sharing? While it is illegal to copy or download music without paying for…
A: Description: 1) The regulation of copyright covers that tune, restricting human beings from…
A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter subclass of Critter whose move method behaves as described.
What do I need to change to make it work?
import java.util.ArrayList;
/**
A simulated critter.
*/
public class Critter //superclass
{
private int position;
private ArrayList<String> history;
/**
Constructs a critter at position 0 with blank history.
*/
public Critter()
{
position = 0;
history = new ArrayList<String>();
}
/**
Gets the history of this critter.
@return the history
*/
public ArrayList<String> getHistory()
{
return history;
}
/**
Adds to the history of this critter.
@param newValue the desired state
*/
public void addHistory(String event)
{
history.add(event);
}
/**
Gets the position of this critter.
@return the position
*/
public int getPosition()
{
return position;
}
/**
Moves this critter.
@param steps the number of steps by which to move.
*/
public void move(int steps)
{
position = position + steps;
addHistory("move to " + position);
}
}
public class FastCritter extends Critter
{
public void act(){
super.move();
n = steps;
move() = 2*n;
}
}
public class FastCritter extends Critter //subclass I am using super to access move() from super class and multiply 2* n (steps)
{
public void act(){
super.move();
n = steps;
move() = 2*n;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

- Consider class IntArrayStack that has two instance variables: int[] data and int top. Implement an instance method inside the IntArrayStack, called popBottom(),that extracts and returns as output the element at the bottom of the stack. All other elements in the stack must remain unchanged and in the same order.Provide a different implementation of ChoiceQuestion. Instead of storing the choices in an array list, the addChoice method should add the choice to the question text. For this purpose, an addLine method has been added to the Question class. /** A question with multiple choices.*/public class ChoiceQuestion extends Question{ // Add any needed instance variables, but don't store the choices // The choices should be added to the text of the superclass /* code goes here */ /** Constructs a choice question with a given text and no choices. @param questionText the text of this question */ public ChoiceQuestion(String questionText) { /code goes here */ } /** Adds an answer choice to this question. @param choice the choice to add @param correct true if this is the correct choice, false otherwise */ public void addChoice(String choice, boolean correct) { /* code goes here */ } }Implement a superclass Appointment and subclasses Onetime, Daily, and Monthly. An appointment has a description (for example, “see the dentist”) and a date (use int's to store the date). Write a method occursOn(int year, int month, int day) that checks whether the appointment occurs on that date. For example, for a monthly appointment, you must check whether the day of the month matches. Additionally, you will need to implement a class called Calendar. This will contain an ArrayList of Appointment objects (The list is an instance variable of the Calendar class). You will need to provide the following methods for your Calendar class. A no argument constructor public Calendar(){...} This constructor will initialize your ArrayList instance variable to an empty list. /** * A method to add an appointment to the calendar * @param apt – the appointment object to add to the calendar. */ public void add(Appointment apt) {…} /** * A method to remove an appointment from the…
- In this problem, we are going to use ArrayLists and classes to design a road trip. You have three classes: GeoLocation.java which represents a geo location. A RoadTrip.java class, which represents a road trip (or an ordered list of places), and a RoadTripTester.java class which brings them all together. In GeoLocation.java: Add a private instance variable called name which is a String. This represents the name of the location. Modify the Geolocation class constructor so that it is now of the format public GeoLocation(String name, double theLatitude, double theLongitude) Add a getter method for name called getName(). Update the toString so that it returns a String of the format San Francisco (37.7833, -122.4167) Now, you’ll also need to create a RoadTrip class. RoadTrip stores an ordered list of locations, so you’ll need to have an ArrayList as an instance variable. The RoadTrip class constructor should initialize an ArrayList that stores a list of GeoLocations. You’ll also need to…In java A supermarket wants to reward its best customer of each day, showing the customer’s name on a screen in the supermarket. For that purpose, the store keeps an ArrayList.Create a class customer having id, name, gender, and bill provide appropriate getters/setters and constructors also provide toString method.In the Store class, make an ArrayList of customers, store name, and address implement methods public void addSale(Customer c) that will add customers to the arraylist.public void RemoveCustomer(int id);public void UpdateCustomerRecord(int Id);public displayAll();public String nameOfBestCustomer() to record the sale and return the name of the customer with the largest sale. public ArrayList nameOfBestCustomers(int topN) so that it displays the top customers, that is, the topN customers with the largest sales, where topN is a value that the user of the program supplies. Write a program that prompts following menu and ============================================…From Big Java 6th edition P9.7: "In this problem, you will model a circuit consisting of an arbitrary configuration of resistors. Provide a superclass Circuit with a instance method getResistance. Provide a subclass Resistor representing a single resistor. Provide subclasses Serial and Parallel, each of which contains an ArrayList<Circuit>. A Serial circuit models a series of circuits, each of which can be a single resistor or another circuit. Similarly, a Parallel circuit models a set of circuits in parallel; using Ohm's Law to compute the combined ressistance." I managed to create appropriate subclasses off of the Circuit superclass. But from here, I'm stuck! How do I compute the combined resistance? and how would I get the method to read the values off of the ArrayList<Circuit>? And does that Circuit refer to the Circuit Class I created, or would that require input for resister amounts?
- Consider the following class PrimeNumbers, which has three methods. The first, computePrimes() takes one integer input and calculates that many prime numbers. Iterator() returns an Iterator object that will iterate through the primes, and toString() returns a string representation. public class PrimeNumbers implements Iterable<Integer> { private List<Integer> primes = new ArrayList<Integer>(); public void computePrimes (int n) { int count = 1; // count of primes int number = 2; // number tested for primeness boolean isPrime; // is this number a prime while (count <= n) { isPrime = true; for (int divisor = 2; divisor <= number / 2; divisor++) { if (number % divisor == 0) { isPrime = false; break; // for loop } } if (isPrime && (number % 10 != 9)) // FAULT { primes.add (number); count++; } number++; } } @Override public Iterator<Integer> iterator() { return primes.iterator(); } 2 @Override public String toString() { return primes.toString();…Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. impliment the solver…Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. import…
- Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. import…Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. import java.util.Arrays;…Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. impliment the solver…
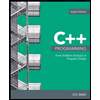
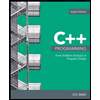