Element 1 Element 2 Element 3 Element 4 end function 2 1 1 Top 2 Task 4: Complete the following function: function SEARCHSTACK(stack, item) 4 The coordinates of the element in yellow are (3,2), for example. So to select the value stored there we use the syntax puzzle[3][2], since we are looking at the second element in puzzle[3]. Using this coordinate system to re- fer to the 2-by-2 sub-grids, for example, the top-left sub-grid will consist of the elements (1,1), (1,2), (2,1) and (2,2). You will check the Pseudoku conditions using a stack. In particular, you will start with a stack containing all numbers from 1 to 4, and then inspect each element in each column (or sub-grid) to see if the number in that element is stored in the stack: if it is stored in the stack, pop that value from the stack and return the stack; otherwise, return false. This process will be repeated for every element in a column or sub-grid. If, at the end of checking every element, the stack is empty then all numbers appear in the the column or sub-grid; if the stack is not empty, then not all numbers from 1 to 4 appear. We will complete a function called SEARCHSTACK(stack, item) that will search a stack for the value item: if item is in one of the elements of the stack, we remove that element storing item. Otherwise, the function should return FALSE Let's demonstrate this with diagram. We have the following stack: 2 1 1 Top 1 3 2 3 3 4 We want to look for the value 3 in the stack and remove it if it is there, otherwise if it is not there, return FALSE. The value 3 is stored in the stack so we then need to remove it so the stack could look like this: 3 The next task will be to complete a function that does this process of searching a stack and possibly removing an element. This function will take a stack and a value (called item) as input parameters, and return FALSE if item is not stored in the stack, otherwise return the stack without the element storing item.
Element 1 Element 2 Element 3 Element 4 end function 2 1 1 Top 2 Task 4: Complete the following function: function SEARCHSTACK(stack, item) 4 The coordinates of the element in yellow are (3,2), for example. So to select the value stored there we use the syntax puzzle[3][2], since we are looking at the second element in puzzle[3]. Using this coordinate system to re- fer to the 2-by-2 sub-grids, for example, the top-left sub-grid will consist of the elements (1,1), (1,2), (2,1) and (2,2). You will check the Pseudoku conditions using a stack. In particular, you will start with a stack containing all numbers from 1 to 4, and then inspect each element in each column (or sub-grid) to see if the number in that element is stored in the stack: if it is stored in the stack, pop that value from the stack and return the stack; otherwise, return false. This process will be repeated for every element in a column or sub-grid. If, at the end of checking every element, the stack is empty then all numbers appear in the the column or sub-grid; if the stack is not empty, then not all numbers from 1 to 4 appear. We will complete a function called SEARCHSTACK(stack, item) that will search a stack for the value item: if item is in one of the elements of the stack, we remove that element storing item. Otherwise, the function should return FALSE Let's demonstrate this with diagram. We have the following stack: 2 1 1 Top 1 3 2 3 3 4 We want to look for the value 3 in the stack and remove it if it is there, otherwise if it is not there, return FALSE. The value 3 is stored in the stack so we then need to remove it so the stack could look like this: 3 The next task will be to complete a function that does this process of searching a stack and possibly removing an element. This function will take a stack and a value (called item) as input parameters, and return FALSE if item is not stored in the stack, otherwise return the stack without the element storing item.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18PE
Related questions
Question
![Element 1
Element 2
Element 3
Element 4
end function
2
1
†
Top
2
2
Task 4: Complete the following function:
function SEARCHSTACK(stack, item)
2
4
4
2
1
1
Top
4
The coordinates of the element in yellow are (3,2), for example. So to select the value stored there we use the
syntax puzzle[3][2], since we are looking at the second element in puzzle [3]. Using this coordinate system to re-
fer to the 2-by-2 sub-grids, for example, the top-left sub-grid will consist of the elements (1,1), (1,2), (2,1) and (2,2).
You will check the Pseudoku conditions using a stack. In particular, you will start with a stack containing all
numbers from 1 to 4, and then inspect each element in each column (or sub-grid) to see if the number in that
element is stored in the stack: if it is stored in the stack, pop that value from the stack and return the stack;
otherwise, return false. This process will be repeated for every element in a column or sub-grid. If, at the end of
checking every element, the stack is empty then all numbers appear in the the column or sub-grid; if the stack is
not empty, then not all numbers from 1 to 4 appear.
We will complete a function called SEARCHSTACK(stack, item) that will search a stack for the value item: if
item is in one of the elements of the stack, we remove that element storing item. Otherwise, the function should
return FALSE Let's demonstrate this with diagram. We have the following stack:
1
1
3
1
We want to look for the value 3 in the stack and remove it if it is there, otherwise if it is not there, return FALSE.
The value 3 is stored in the stack so we then need to remove it so the stack could look like this:
3
4
4
The next task will be to complete a function that does this process of searching a stack and possibly removing an
element.
This function will take a stack and a value (called item) as input parameters, and return FALSE if item is not stored
in the stack, otherwise return the stack without the element storing item.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb5c8e275-2683-4d09-b1c0-4142df9a1282%2F02900203-0d94-4175-a10e-e7958839ae09%2Fgz5fg4_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Element 1
Element 2
Element 3
Element 4
end function
2
1
†
Top
2
2
Task 4: Complete the following function:
function SEARCHSTACK(stack, item)
2
4
4
2
1
1
Top
4
The coordinates of the element in yellow are (3,2), for example. So to select the value stored there we use the
syntax puzzle[3][2], since we are looking at the second element in puzzle [3]. Using this coordinate system to re-
fer to the 2-by-2 sub-grids, for example, the top-left sub-grid will consist of the elements (1,1), (1,2), (2,1) and (2,2).
You will check the Pseudoku conditions using a stack. In particular, you will start with a stack containing all
numbers from 1 to 4, and then inspect each element in each column (or sub-grid) to see if the number in that
element is stored in the stack: if it is stored in the stack, pop that value from the stack and return the stack;
otherwise, return false. This process will be repeated for every element in a column or sub-grid. If, at the end of
checking every element, the stack is empty then all numbers appear in the the column or sub-grid; if the stack is
not empty, then not all numbers from 1 to 4 appear.
We will complete a function called SEARCHSTACK(stack, item) that will search a stack for the value item: if
item is in one of the elements of the stack, we remove that element storing item. Otherwise, the function should
return FALSE Let's demonstrate this with diagram. We have the following stack:
1
1
3
1
We want to look for the value 3 in the stack and remove it if it is there, otherwise if it is not there, return FALSE.
The value 3 is stored in the stack so we then need to remove it so the stack could look like this:
3
4
4
The next task will be to complete a function that does this process of searching a stack and possibly removing an
element.
This function will take a stack and a value (called item) as input parameters, and return FALSE if item is not stored
in the stack, otherwise return the stack without the element storing item.
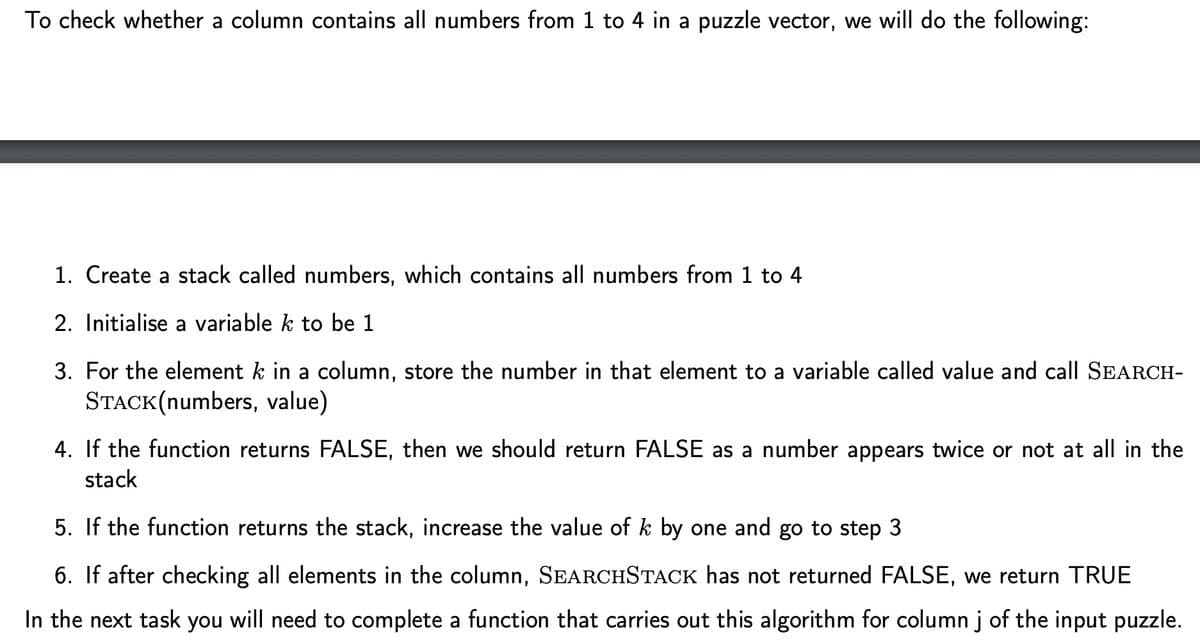
Transcribed Image Text:To check whether a column contains all numbers from 1 to 4 in a puzzle vector, we will do the following:
1. Create a stack called numbers, which contains all numbers from 1 to 4
2. Initialise a variable k to be 1
3. For the element k in a column, store the number in that element to a variable called value and call SEARCH-
STACK(numbers, value)
4. If the function returns FALSE, then we should return FALSE as a number appears twice or not at all in the
stack
5. If the function returns the stack, increase the value of k by one and go to step 3
6. If after checking all elements in the column, SEARCHSTACK has not returned FALSE, we return TRUE
In the next task you will need to complete a function that carries out this algorithm for column j of the input puzzle.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
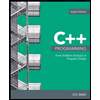
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
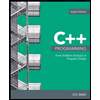
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning