esolve the errors from the guuven code and made this programe run able: Programe is for to solve the algebric expression using stack in java: Attach the output's screentshots and explain every line with comments: import java.util.Arrays; import java.util.Scanner; import java.util.HashSet; import java.util.StringTokenizer; public class ArithmeticExpressio
Course: Data Structure and
Language: Java
Resolve the errors from the guuven code and made this programe run able:
Programe is for to solve the algebric expression using stack in java:
Attach the output's screentshots and explain every line with comments:
import java.util.Arrays;
import java.util.Scanner;
import java.util.HashSet;
import java.util.StringTokenizer;
public class ArithmeticExpressionInfix {
public static String evaluate(String inputAlgebraicExpression){
inputAlgebraicExpression = inputAlgebraicExpression.replaceAll("\\s+", "");
GenericStack<String> stack = new GenericStack<String>(inputAlgebraicExpression.length());
StringTokenizer tokens = new StringTokenizer(inputAlgebraicExpression, "{}()*/+-", true);
while(tokens.hasMoreTokens()){
String tkn = tokens.nextToken();
if(tkn.equals("(")
|| tkn.equals("{")
|| tkn.matches("[0-9]+")
|| tkn.equals("*")
|| tkn.equals("/")
|| tkn.equals("+")
|| tkn.equals("-")){
stack.push(tkn);
} else if(tkn.equals("}") || tkn.equals(")")){
try {
int op2 = Integer.parseInt(stack.pop());
String oprnd = stack.pop();
int op1 = Integer.parseInt(stack.pop());
if(!stack.isEmpty()){
stack.pop();
}
int result = 0;
if(oprnd.equals("*")){
result = op1*op2;
} else if(oprnd.equals("/")){
result = op1/op2;
} else if(oprnd.equals("+")){
result = op1+op2;
} else if(oprnd.equals("-")){
result = op1-op2;
}
stack.push(result+"");
} catch (Exception e) {
e.printStackTrace();
break;
}
}
}
String finalResult = "";
try {
finalResult = stack.pop();
} catch (Exception e) {
e.printStackTrace();
}
return finalResult;
}
public static boolean isValidExpression(String expression) throws Exception {
//Check whether the start and the end entry are valid elements
if ((!Character.isDigit(expression.charAt(0)) && !(expression.charAt(0) == '('))|| (!Character.isDigit(expression.charAt(expression.length() - 1)) && !(expression.charAt(expression.length() - 1) == ')'))) {
return false;
}
HashSet<Character> validCharactersSet = new HashSet<>();
validCharactersSet.add('*');
validCharactersSet.add('+');
validCharactersSet.add('-');
validCharactersSet.add('/');
validCharactersSet.add('%');
validCharactersSet.add('(');
validCharactersSet.add(')');
GenericStack<String> validParenthesisCheck = new GenericStack<String>(expression.length());for (int i = 0; i < expression.length(); i++) {
if (!Character.isDigit(expression.charAt(i)) &&!validCharactersSet.contains(expression.charAt(i))) {
return false;
}
if (expression.charAt(i) == '(') {
validParenthesisCheck.push(""+expression.charAt(i));
}
if (expression.charAt(i) == ')') {
if (validParenthesisCheck.isEmpty()) {
return false;
}
validParenthesisCheck.pop();
}
}
if (validParenthesisCheck.isEmpty()) {
return true;
} else {
return false;
}
}
public static void main(String args[]) throws Exception{
if(args.length<1)
throw new Exception("Usage: java ArithmeticExpressionInfix <algebraic_expression>");
String inputAlgebraicExpression = args[0];
System.out.println("Expression: "+inputAlgebraicExpression);
boolean flag = isValidExpression(inputAlgebraicExpression);
if(!flag) {
System.out.println("Invalid Input");
System.exit(1);
}
System.out.println("Final Result:"+evaluate(inputAlgebraicExpression));
}
}
class GenericStack<T extends Object> {
private int stackSize;
private T[] stackArr;
private int top;
@SuppressWarnings("unchecked")
public GenericStack(int size) {
this.stackSize = size;
this.stackArr = (T[]) new Object[stackSize];
this.top = -1;
}
public void push(T entry){
if(this.isFull()){
System.out.println(("Stack is full. Increasing the capacity."));
this.increaseCapacity(); }
System.out.println("Adding: "+entry);
this.stackArr[++top] = entry;
}
public T pop() throws Exception {
if(this.isEmpty()){
throw new Exception("Stack is empty. Can not remove element.");
}
T entry = this.stackArr[top--];
System.out.println("Removed entry: "+entry);
return entry;
}
public T peek() {
return stackArr[top];
}
private void increaseCapacity(){
@SuppressWarnings("unchecked")
T[] newStack = (T[]) new Object[this.stackSize*2];
for(int i=0;i<stackSize;i++){
newStack[i] = this.stackArr[i];
}
this.stackArr = newStack;
this.stackSize = this.stackSize*2;
}
public boolean isEmpty() {
return (top == -1);
}
public boolean isFull() {
return (top == stackSize - 1);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

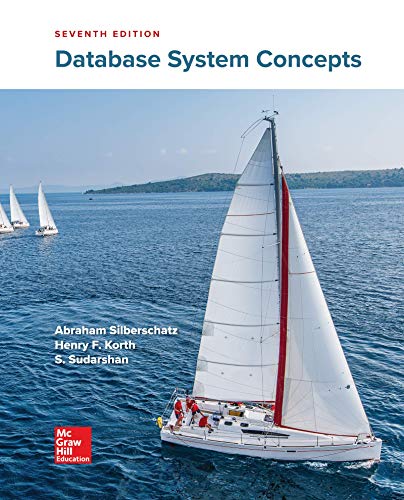
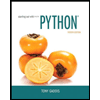
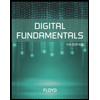
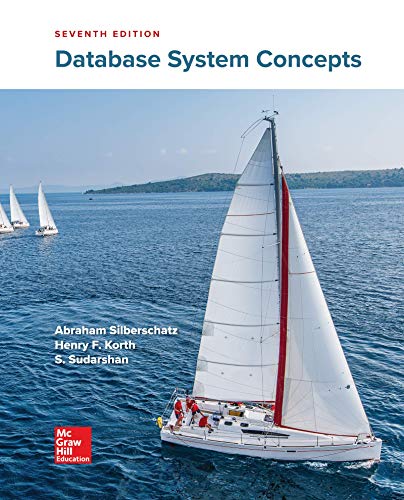
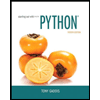
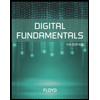
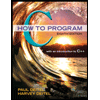
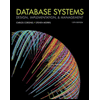
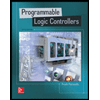