Interpreter.java is missing these methods in the code so make sure to add them: -print, printf: Exist, marked as variadic, call Java functions -getline and next: Exist and call SplitAndAssign -gsub, match, sub, index, length, split, substr, tolower, toupper: Exist, call Java functions, correct return Below is interpreter.java import java.util.ArrayList; import java.util.HashMap; import java.util.List; public class Interpreter { private HashMap globalVariables; private HashMap functions; private class LineManager { private List lines; private int currentLineIndex; public LineManager(List inputLines) { this.lines = inputLines; this.currentLineIndex = 0; } public boolean splitAndAssign() { if (currentLineIndex < lines.size()) { String currentLine = lines.get(currentLineIndex); currentLineIndex++; // Move to the next line return true; } return false; } } public Interpreter(ProgramNode node, String path) { globalVariables = new HashMap<>(); functions = new HashMap<>(); if (path != null) { List inputLines = readAndParseFile(path); LineManager lineManager = new LineManager(inputLines); } } private List readAndParseFile(String path) { return new ArrayList<>(); } }
Interpreter.java is missing these methods in the code so make sure to add them: -print, printf: Exist, marked as variadic, call Java functions -getline and next: Exist and call SplitAndAssign -gsub, match, sub, index, length, split, substr, tolower, toupper: Exist, call Java functions, correct return Below is interpreter.java import java.util.ArrayList; import java.util.HashMap; import java.util.List; public class Interpreter { private HashMap globalVariables; private HashMap functions; private class LineManager { private List lines; private int currentLineIndex; public LineManager(List inputLines) { this.lines = inputLines; this.currentLineIndex = 0; } public boolean splitAndAssign() { if (currentLineIndex < lines.size()) { String currentLine = lines.get(currentLineIndex); currentLineIndex++; // Move to the next line return true; } return false; } } public Interpreter(ProgramNode node, String path) { globalVariables = new HashMap<>(); functions = new HashMap<>(); if (path != null) { List inputLines = readAndParseFile(path); LineManager lineManager = new LineManager(inputLines); } } private List readAndParseFile(String path) { return new ArrayList<>(); } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Interpreter.java is missing these methods in the code so make sure to add them:
-print, printf: Exist, marked as variadic, call Java functions
-getline and next: Exist and call SplitAndAssign
-gsub, match, sub, index, length, split, substr, tolower, toupper: Exist, call Java functions, correct return
Below is interpreter.java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class Interpreter {
private HashMap<String, InterpreterDataType> globalVariables;
private HashMap<String, FunctionDefinitionNode> functions;
private class LineManager {
private List<String> lines;
private int currentLineIndex;
public LineManager(List<String> inputLines) {
this.lines = inputLines;
this.currentLineIndex = 0;
}
public boolean splitAndAssign() {
if (currentLineIndex < lines.size()) {
String currentLine = lines.get(currentLineIndex);
currentLineIndex++; // Move to the next line
return true;
}
return false;
}
}
public Interpreter(ProgramNode node, String path) {
globalVariables = new HashMap<>();
functions = new HashMap<>();
if (path != null) {
List<String> inputLines = readAndParseFile(path);
LineManager lineManager = new LineManager(inputLines);
}
}
private List<String> readAndParseFile(String path) {
return new ArrayList<>();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
There are errors in lines 56 and 57. Please fix those errors and show the fixed code.
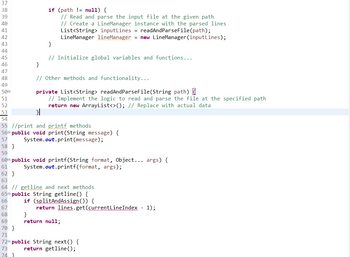
Transcribed Image Text:789FHQB4 45 46 47 48
37
38
39
40
41
42
43
44
49
500
51
52
53
}
if (path != null) {
// Read and parse the input file at the given path
// Create a LineManager instance with the parsed lines
List<String> inputLines = readAndParseFile(path);
LineManager lineManager = new LineManager (inputLines);
69
70 }
71
}
// Initialize global variables and functions...
// Other methods and functionality...
private List<String> readAndParseFile(String path) {
// Implement the logic to read and parse the file at the specified path
return new ArrayList<>(); // Replace with actual data
}
54
55 //print and printf methods
56 public void print (String message) {
57
System.out.print (message);
58 }
59
60 public void printf(String format, Object... args) {
System.out.printf(format, args);
61
62 }
63
64 //getline and next methods
65 public String getline() {
66
67
68
if (splitAndAssign()) {
return lines.get(currentLineIndex - 1);
}
return null;
72 public String next() {
73
return getline();
74 }
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
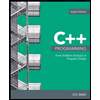
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
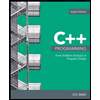
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning