For this assignment, you will be creating two functions, alongside a main function: 1) toRadians: takes in degrees as input, and returns radians. Given a specified number of degrees, the radians is equal to degrees * PI/180. Important: Be sure to declare PI to be 3.14159. This is so that mimir always comes up with the same answers no matter which server is used. 2) Drawline: takes in two parameters: a character and numRepetitions and prints the character numRepetitions times, followed by a newline. In your main function, for every 5 degrees between 0 and 360, calculate and print out the value of sin(toRadians(degree)) like so: GIVEN 45 DEGREES: sin(45) = 0.70711 To use the sin function, make sure to include cmath in your program. Be sure to set decimal precision to 5 places! Every 90 degrees (including 0 and 360), print out a line of 30 dashes ('-') using drawline after your print out the value of sin. Inside main() For values between 0 and 360, in 5 degree increments: calculate and print the value of sin (degrees), After every 90 degrees, print out a line of 30 dashes (minus signs '-') (Extra information : #include // for sin function In order to have the precise format we want: Put this at the top of main: cout.setf(ios::fixed); cout.setf(ios::showpoint); // show decimals even if not needed cout.precision(5); // show 5 digits to right of decimal ) WHAT THE OUTPUT SHOULD BE EXACTLY sin(0) = 0.00000 ------------------------------ sin(5) = 0.08716 sin(10) = 0.17365 sin(15) = 0.25882 sin(20) = 0.34202 sin(25) = 0.42262 sin(30) = 0.50000 sin(35) = 0.57358 sin(40) = 0.64279 sin(45) = 0.70711 sin(50) = 0.76604 sin(55) = 0.81915 sin(60) = 0.86602 sin(65) = 0.90631 sin(70) = 0.93969 sin(75) = 0.96593 sin(80) = 0.98481 sin(85) = 0.99619 sin(90) = 1.00000 ------------------------------ sin(95) = 0.99619 sin(100) = 0.98481 sin(105) = 0.96593 sin(110) = 0.93969 sin(115) = 0.90631 sin(120) = 0.86603 sin(125) = 0.81915 sin(130) = 0.76605 sin(135) = 0.70711 sin(140) = 0.64279 sin(145) = 0.57358 sin(150) = 0.50000 sin(155) = 0.42262 sin(160) = 0.34202 sin(165) = 0.25882 sin(170) = 0.17365 sin(175) = 0.08716 sin(180) = 0.00000 ------------------------------ sin(185) = -0.08715 sin(190) = -0.17365 sin(195) = -0.25882 sin(200) = -0.34202 sin(205) = -0.42262 sin(210) = -0.50000 sin(215) = -0.57357 sin(220) = -0.64279 sin(225) = -0.70710 sin(230) = -0.76604 sin(235) = -0.81915 sin(240) = -0.86602 sin(245) = -0.90631 sin(250) = -0.93969 sin(255) = -0.96592 sin(260) = -0.98481 sin(265) = -0.99619 sin(270) = -1.00000 ------------------------------ sin(275) = -0.99620 sin(280) = -0.98481 sin(285) = -0.96593 sin(290) = -0.93969 sin(295) = -0.90631 sin(300) = -0.86603 sin(305) = -0.81915 sin(310) = -0.76605 sin(315) = -0.70711 sin(320) = -0.64279 sin(325) = -0.57358 sin(330) = -0.50000 sin(335) = -0.42262 sin(340) = -0.34202 sin(345) = -0.25882 sin(350) = -0.17365 sin(355) = -0.08716 sin(360) = -0.00001 ------------------------------
Call the file: sinDrawline.cpp
For this assignment, you will be creating two functions, alongside a main function:
1) toRadians: takes in degrees as input, and returns radians. Given a specified number of degrees, the radians is equal to degrees * PI/180.
Important: Be sure to declare PI to be 3.14159. This is so that mimir always comes up with the same answers no matter which server is used.
2) Drawline: takes in two parameters: a character and numRepetitions and prints the character numRepetitions times, followed by a newline.
In your main function, for every 5 degrees between 0 and 360, calculate and print out the value of sin(toRadians(degree)) like so:
GIVEN 45 DEGREES:
sin(45) = 0.70711
To use the sin function, make sure to include cmath in your program. Be sure to set decimal precision to 5 places!
Every 90 degrees (including 0 and 360), print out a line of 30 dashes ('-') using drawline after your print out the value of sin.
Inside main()
For values between 0 and 360, in 5 degree increments:
calculate and print the value of sin (degrees),
After every 90 degrees, print out a line of 30 dashes (minus signs '-')
(Extra information :
#include <cmath> // for sin function
In order to have the precise format we want:
Put this at the top of main:
cout.setf(ios::fixed);
cout.setf(ios::showpoint); // show decimals even if not needed
cout.precision(5); // show 5 digits to right of decimal )
WHAT THE OUTPUT SHOULD BE EXACTLY
sin(0) = 0.00000
------------------------------
sin(5) = 0.08716
sin(10) = 0.17365
sin(15) = 0.25882
sin(20) = 0.34202
sin(25) = 0.42262
sin(30) = 0.50000
sin(35) = 0.57358
sin(40) = 0.64279
sin(45) = 0.70711
sin(50) = 0.76604
sin(55) = 0.81915
sin(60) = 0.86602
sin(65) = 0.90631
sin(70) = 0.93969
sin(75) = 0.96593
sin(80) = 0.98481
sin(85) = 0.99619
sin(90) = 1.00000
------------------------------
sin(95) = 0.99619
sin(100) = 0.98481
sin(105) = 0.96593
sin(110) = 0.93969
sin(115) = 0.90631
sin(120) = 0.86603
sin(125) = 0.81915
sin(130) = 0.76605
sin(135) = 0.70711
sin(140) = 0.64279
sin(145) = 0.57358
sin(150) = 0.50000
sin(155) = 0.42262
sin(160) = 0.34202
sin(165) = 0.25882
sin(170) = 0.17365
sin(175) = 0.08716
sin(180) = 0.00000
------------------------------
sin(185) = -0.08715
sin(190) = -0.17365
sin(195) = -0.25882
sin(200) = -0.34202
sin(205) = -0.42262
sin(210) = -0.50000
sin(215) = -0.57357
sin(220) = -0.64279
sin(225) = -0.70710
sin(230) = -0.76604
sin(235) = -0.81915
sin(240) = -0.86602
sin(245) = -0.90631
sin(250) = -0.93969
sin(255) = -0.96592
sin(260) = -0.98481
sin(265) = -0.99619
sin(270) = -1.00000
------------------------------
sin(275) = -0.99620
sin(280) = -0.98481
sin(285) = -0.96593
sin(290) = -0.93969
sin(295) = -0.90631
sin(300) = -0.86603
sin(305) = -0.81915
sin(310) = -0.76605
sin(315) = -0.70711
sin(320) = -0.64279
sin(325) = -0.57358
sin(330) = -0.50000
sin(335) = -0.42262
sin(340) = -0.34202
sin(345) = -0.25882
sin(350) = -0.17365
sin(355) = -0.08716
sin(360) = -0.00001
------------------------------

Step by step
Solved in 2 steps with 1 images

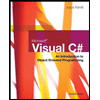
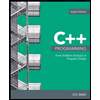
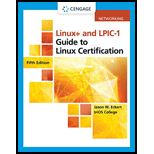
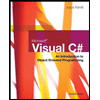
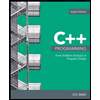
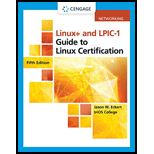