Goal 1: Update the Fractions Class Reduce fractions (i.e. 15/18 SHOULD be reduced to 5/6), Add the "const" keyword to our member functions. Update Member Functions: The parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement assert(denominatorParameter != 0); assert() is not the best way to handle this, but it will have to do until we study exception handling. Add the const keyword to your methods wherever appropriate and also make sure to pass objects by reference. Your class may still work correctly even if you don't do this correctly, so this will require extra care! Add a private "simplify()" function to your class, which will reduce your fraction (for example, 15/18 becomes 5/6). Following is an algorithm that you may use for your simplify() function. algorithm: finds the greatest common factor of numerator and denominator and then divides both numerator and denominator by the result. The greatest common factor is found by starting at the numerator or denominator, whichever is least, and decrementing until a number is found that goes evenly into both numerator and denominator.Fractions should not be simply reduced upon output, they should be storedin reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. Call simplify() from the appropriate member functions (For most of you there will be 5 places where you need to call it. This, however, depends on how you write the class, so don't assume you are wrong if you don't have exactly 5). New Member functions: You will add four member functions to your Fraction class: Copy Constructor, Overloaded Assignment Operator (=), Overloaded equality operator (==). This function has the same functionality as the IsEqual method before. Overloaded output operator (<<). This function has the same functionality as the print method before but with one change: if the numerator is 0 or the denominator is 1, it only displays the numerator; otherwise, it displays both the numerator and the denominator. Output: The product of 9/8 and 2/3 is 3/4 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions (9/8 and 2/3) are not equal. The two Fractions (3/4 and 3/4) are equal. The product of 0 and 3/4 is 0 The quotient of 3/4 and 3/4 is 1 The sum of 4/5 and 6/5 is 2 Goal 2: Creating a new "Recipe" class Member functions: A default constructor that creates an empty recipe (how will each of the member variables be initialized if there is no recipe?). A parametrized constructor that takes three arguments, a string containing the name of the recipe, a vector of strings containing the names of the ingredients, and a vector of Fractions containing the quantity of each ingredient, and assigns the attributes accordingly: Recipe(string& recipeName, vector& name, vector& quantity); 3. A getter function called getitems that returns the number of ingredients in the recipe. Note: it also needs to be defined as const. 4. An overloaded output operator (<<) that displays the recipe name, followed by each ingredient along with their fractional quantity. See the sample output below for exact specifications. Output: The product of 9/8 and 2/3 is 3/4 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions (3/4 and 3/3) are eat equal. The two Fractions (3/4 and 3/4) are equal The product of 0 and 3/4 is 0 The quotient of 3/4 and 3/4 is 1 The sum of 4/5 and 6/5 is 2 Following Recipe has 4 ingredients ---Peanut Sauce Recipe---- Sweet Chilli Sauce (3/4) Peanut Butter (1/3) Hoisin Sauce (1/2) Following Recipe has 6 ingredients ---Vegetable Lasagna Recipe --- Bowtie Pasta (4) Swiss Cheese (2/3) Marinara (7/2) Spinach (3/4) Crushed Red Pepper (1/4) Salt (1)
Goal 1: Update the Fractions Class
- Reduce fractions (i.e. 15/18 SHOULD be reduced to 5/6),
- Add the "const" keyword to our member functions.
Update Member Functions:
-
The parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement
assert(denominatorParameter != 0);assert() is not the best way to handle this, but it will have to do until we study exception handling.
-
Add the const keyword to your methods wherever appropriate and also make sure to pass objects by reference. Your class may still work correctly even if you don't do this correctly, so this will require extra care!
-
Add a private "simplify()" function to your class, which will reduce your fraction (for example, 15/18 becomes 5/6). Following is an
algorithm: finds the greatest common factor of numerator and denominator andalgorithm that you may use for your simplify() function.
then divides both numerator and denominator by the result. The greatest
common factor is found by starting at the numerator or denominator, whichever
is least, and decrementing until a number is found that goes evenly into both
numerator and denominator.Fractions should not be simply reduced upon output, they should be storedin reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. Call simplify() from the appropriate member functions (For most of you there will be 5 places where you need to call it. This, however, depends on how you write the class, so don't assume you are wrong if you don't have exactly 5).
New Member functions:
You will add four member functions to your Fraction class:
- Copy Constructor,
- Overloaded Assignment Operator (=),
- Overloaded equality operator (==). This function has the same functionality as the IsEqual method before.
- Overloaded output operator (<<). This function has the same functionality as the print method before but with one change: if the numerator is 0 or the denominator is 1, it only displays the numerator; otherwise, it displays both the numerator and the denominator.
Output:
The product of 9/8 and 2/3 is 3/4
The quotient of 9/8 and 2/3 is 27/16
The sum of 9/8 and 2/3 is 43/24
The difference of 9/8 and 2/3 is 11/24
The two Fractions (9/8 and 2/3) are not equal.
The two Fractions (3/4 and 3/4) are equal.
The product of 0 and 3/4 is 0
The quotient of 3/4 and 3/4 is 1
The sum of 4/5 and 6/5 is 2
Goal 2: Creating a new "Recipe" class
Member functions:
- A default constructor that creates an empty recipe (how will each of the member variables be initialized if there is no recipe?).
- A parametrized constructor that takes three arguments, a string containing the name of the recipe, a
vector of strings containing the names of the ingredients, and a vector of Fractions containing the quantity of each ingredient, and assigns the attributes accordingly:
Recipe(string& recipeName, vector<string>& name, vector<Fraction>& quantity);
3. A getter function called getitems that returns the number of ingredients in the recipe. Note: it also needs to be defined as const.
4. An overloaded output operator (<<) that displays the recipe name, followed by each ingredient along with their fractional quantity. See the sample output below for exact specifications.
Output:
The product of 9/8 and 2/3 is 3/4
The quotient of 9/8 and 2/3 is 27/16
The sum of 9/8 and 2/3 is 43/24
The difference of 9/8 and 2/3 is 11/24
The two Fractions (3/4 and 3/3) are eat equal.
The two Fractions (3/4 and 3/4) are equal
The product of 0 and 3/4 is 0
The quotient of 3/4 and 3/4 is 1
The sum of 4/5 and 6/5 is 2
Following Recipe has 4 ingredients
---Peanut Sauce Recipe----
Sweet Chilli Sauce (3/4)
Peanut Butter (1/3)
Hoisin Sauce (1/2)
Following Recipe has 6 ingredients
---Vegetable Lasagna Recipe ---
Bowtie Pasta (4)
Swiss Cheese (2/3)
Marinara (7/2)
Spinach (3/4)
Crushed Red Pepper (1/4)
Salt (1)

Step by step
Solved in 5 steps with 6 images

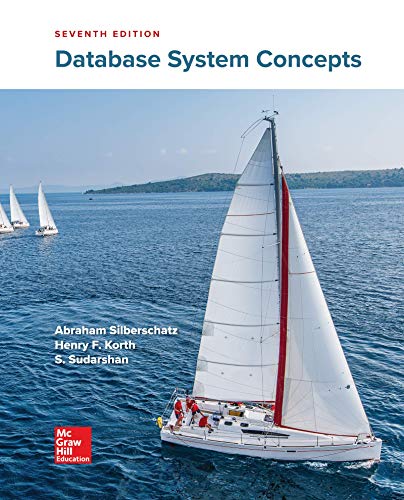
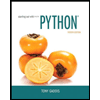
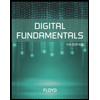
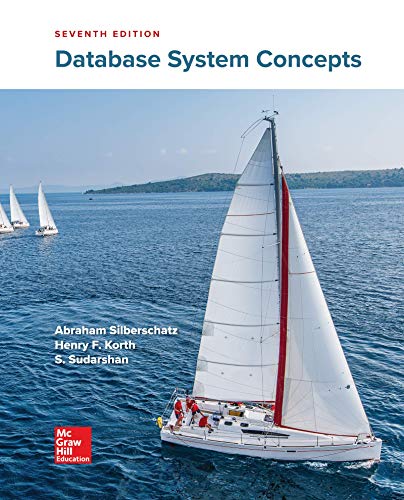
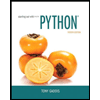
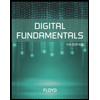
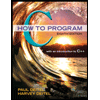
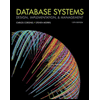
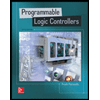