he function should determine the mode of the array or modes. That is, it should determine which value or values in the array occurs most often. The mode is the value or values the function should return. If the array has no mode (none of the values occur more than once), the function should return an empty vector. (Assume the array will always contain nonnegative values). Write functions that fill the array with random numbers. Test your function thoroughly. Outcome Criteria 1) program compiles 2) program solves problem according to specification 3) program declares, creates, or initializes static or dynamic array correctly 4) if required program defines function or functions with array parameters or array returns 5) program uses arrays to solve problem 6) program destroys any dynamic arrays
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Hello, can you please help me. Can you check the code below with the instructions I may have missed something and please check line 69 because I get an error there? If possible, once complete please run the
---------------------------------------------------------------------------
In statistics, the mode of a set of values is the value that occurs most often or with the greatest frequency. Write a function that accepts as arguments the following:
- an array of integers.
- B) An integer that indicates the number of elements in the array and returns a
vector of integers. - You may or may not sort the array.
The function should determine the mode of the array or modes. That is, it should determine which value or values in the array occurs most often. The mode is the value or values the function should return. If the array has no mode (none of the values occur more than once), the function should return an empty vector. (Assume the array will always contain nonnegative values).
Write functions that fill the array with random numbers.
Test your function thoroughly.
Outcome Criteria
1) program compiles
2) program solves problem according to specification
3) program declares, creates, or initializes static or dynamic array correctly
4) if required program defines function or functions with array parameters or array returns
5) program uses arrays to solve problem
6) program destroys any dynamic arrays
#include <iostream>
using namespace std;
int detectMode(int* data, int size)
{
if (size == 0)
return 0;
int i, j, mode, frequency = 0, count = 0;
int** freq_array = (int**) (2 * sizeof(int*));
for (i = 0;i < 2;i++)
freq_array[i] = (int*) (size * sizeof(int));
for (i = 0;i < size;i++)
freq_array[1][i] = 0;
for (i = 0;i < size;i++)
{
for (j = 0;j < count;j++)
if (freq_array[0][j] == data[i])
{
freq_array[1][j]++;
break;
}
if (j == count)
{
freq_array[0][count] = data[i];
freq_array[1][count] = 1;
count++;
}
}
for (i = 0;i < count;i++)
if (freq_array[1][i] > frequency)
{
mode = freq_array[0][i];
frequency = freq_array[1][i];
}
for (i = 0;i < 2;i++)
free(freq_array[i]);
if (frequency == 1)
return -1;
else
return mode;
}
int main()
{
int n, i, result;
cout << "Enter size of array ";
cin >> n;
int data[n]; //Gives error under the n [n]
for (i = 0;i < n;i++)
cin >> data[i];
result = detectMode(data, n);
if (result == -1)
cout << "Mode not found\n";
else
cout << "Mode is " << result << endl;
return 1;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

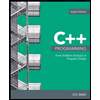
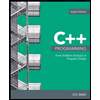