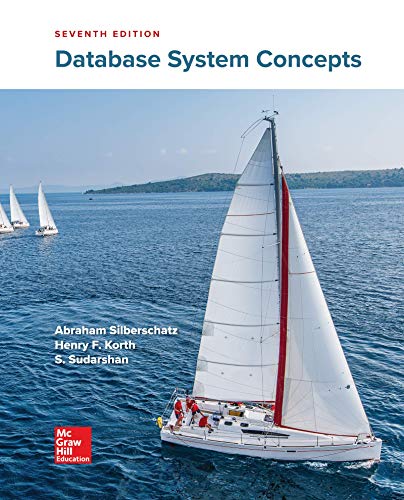
Using PyCharm, create a new Project lab12. Under lab12 create a new Directory exercise,
then create a Python file called name.py in which to write a class named Name. This class
holds the entire name of a person, that is, first, middle and last names and a suffix, and
keeps count of how many of these names are being used (this is explained below).
1. The class has the following fields: count, first, middle, last and suffix.
2. The class has a constructor that receives one string parameter named name whose
default value is an empty string (that is, calling the constructor with no parameters
sets the value of name to an empty string). The constructor initializes all fields based
on the value of name, as explained next:
– The value of count depends on the number of words in name. For example,
given the name Robert John Downey Jr then count is 4.
– If name has one word then it has a first name.
– If name has two words then it has a first and last names in that order.
– If name has three words then it has a first, middle and last names in that order.
– If name has four words then it has a first, middle, last names and suffix in that
order.
Assume that name will never have more than 4 names; that is, it will have one of the
following formats exactly:
– first
– first last
– first middle last
– first middle last suffix
Hint: Use split( ) to get an array of words.
3. The class has getter and setter methods for fields first, middle, last and suffix.
Each setter should adjust (as needed) the value of count each time a name is given or
made empty.
That is, if the name is empty and is set to a non-empty value then count is
incremented. If the name is not empty and is set to an empty value then count is
decremented. Note that count is not modified if the name has a value and is set to
another value, or if it is empty and is set to an empty value (which is kind of pointless,
really, but can happen). For example, if the middle name is empty and we call method
set_middle("Bob") then the middle name changes to "Bob" and count is incremented.
No other fields should change.
The methods to implement are:
– set_first(self, value ): sets the value of first.
– get_first(self): returns the value of first.
– set_middle(self, value ): sets the value of middle.
– get_middle(self): returns the value of middle.
– set_last(self, value ): sets the value of last.
– get_last(self): returns the value of last.
– set_suffix(self, value ): sets the value of suffix.
– get_suffix(self): returns the value of suffix.
4. The class has the following additional methods
– get_count(self): returns the value of count.
– get_name(self): returns the concatenation of first + " " + middle + " " + last
+ " " + suffix with only one space between names as appropriate. For example,
if middle name is empty then the method returns first + " " + last + " " +
suffix with one space in between.
– get_initials(self): returns the concatenation of the first letter in first +
middle + last if each respective name exists. For example, if there is no middle
name, then it returns the initials of the first and last names.
Hints
• In your setter methods: Pay close attention to when you need to increment and
decrement the name count.
• In your get_name(self) method: Pay attention to spacing to ensure that there are no
additional whitespaces in your output. For example, if the first name is "Robert", the
middle name is empty and the last name is "Downey" then the output should be
"Robert Downey" (one space in between), not "Robert Downey" (two+ spaces in
between).
Done!

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 8 images

- Given main(), complete the Artist class (in files Artist.h and Artist.cpp) with constructors to initialize an artist's information, get member functions, and a PrintInfo() member function. The default constructor should initialize the artist's name to "unknown" and the years of birth and death to -1. PrintInfo() displays "Artist:", then a space, then the artist's name, then another space, then the birth and death dates in one of three formats: Answer in c++ (XXXX to YYYY) if both the birth and death years are nonnegative (XXXX to present) if the birth year is nonnegative and the death year is negative (unknown) otherwise Complete the Artwork class (in files Artwork.h and Artwork.cpp) with constructors to initialize an artwork's information, get member functions, and a PrintInfo() member function. The default constructor should initialize the title to "unknown", the year created to -1. PrintInfo() displays an artist's information by calling the PrintInfo() function in the Artist class,…arrow_forward1. Create a python file with a class called "Mobile ()" which will allow us to create new mobile phone objects. 2. Each phone has 4 attributes: make model OS color 3. As there are many things a mobile phone can do create at least four methods the phone can do, examples can be receiving a call, playing a video etc...Within the methods, use print statements to reflect the actions. One Method should use more than one attribute in its print statement 4. Create a separate python file to call the Mobile() class and create at least 3 phone objects. 5. After creating the objects, let each phone perform at least two of the methods and printthem on the screen 6. Add comments to both files to explain your processarrow_forwardUse pythonarrow_forward
- A file has r=20000 STUDENT records of fixed-length. Each record has the following fields: NAME (30 bytes), SSN (10 bytes), ADDRESS (40 bytes), PHONE (9 bytes), BIRTHDATE (8 bytes), SEX (1 byte), MAJORDEPTCODE (4 bytes), MINORDEPTCODE (4 bytes), CLASSCODE (4 bytes, integer), and DEGREEPROGRAM (3 bytes). Suppose the block size is 512 bytes. (a) Calculate the record size R in bytes. (b) Calculate the blocking factor (number of tuples per block) and the number of blocks required. (c) Calculate the average block accesses it takes to find a record by doing a linear search on the file. (d) Assume the file is ordered by SSN; calculate the block accesses takes to search for a record given its SSN value by doing a binary search.arrow_forwardCreate a Python file called adopter.py. In this file, write a class called Adopter that matches the following docstring: '''Class -- AdopterRepresents a person that would like to adopt a pet.Attributes:name -- The adopter's name, a string.species_choice -- The species the person would like to adopt e.g. "cat" or "dog"has_pets -- A boolean, which indicates whether or not the adopter alreadyhas pets.Method:...See part B...''' Important: make sure the attributes are passed to the constructor in the order shown above. Here's an example showing how an Adopter object will be created: mr_t = Adopter("Mr. T", "cat", False) The adopter's name is Mr. T, he would like to adopt a cat, and he does not currently have any pets at home. Part B Add a method called is_match to the Adopter class. The method should take a parameter, an instance of a Pet class and return True if the Adopter would be a good match for the Pet and False if not. You do not need to write the Pet class—you can assume someone…arrow_forwardGDB and Getopt Class Activity activity [-r] -b bval value Required Modify the activity program from last week with the usage shown. The value for bval is required to be an integer as is the value at the end. In addition, there should only be one value. Since everything on the command line is read in as a string, these now need to be converted to numbers. You can use the function atoi() to do that conversion. You can do the conversion in the switch or you can do it at the end of the program. The number coming in as bval and the value should be added together to get a total. That should be the only value printed out at the end. Total = x should be the only output from the program upon success. Remove all the other print statements after testing is complete. Take a screenshot of the output to paste into a Word document and submit. Practice Compile the program with the -g option to load the symbol table. Run the program using gdb and use watch on the result so the program stops when the…arrow_forward
- Sometimes data is not always in the format we need it to be in. In this hypothetical situation, you are writing a program to load phone numbers into a database from user entered survey data. The 10 digit phone number will be stored in the database as an integer (e.g. 1234567890), however the user may enter the phone number in any format (e.g. 123-456-7890). You will write a program that creates a new string from the user entered number consisting of only the digits so it should have the same structure as word_quiz.py in the Unit 5 lecture slides. Below are two examples of how your program should run with different inputs: Enter phone number: (530) 555-1212 Numbers: 5305551212 Enter phone number: 916-123-4567 Numbers: 9161234567 The last line of your program should be: print("Numbers:", phoneNum)arrow_forwardI have a text file that have a list of employee's Id and name correspond to it. All the IDs are unique. What I want to do is I want to read from the text file and then sort the Id in order then put it back into the text file. How can I do this in java? Can you write a code for it in java. For example: the text file text.txt contains Id Name 3 Kark 1 Mia 5 Tyrus 2 John After sort, the text.txt will be 1 Mia 2 John 3 Karl 5 Tyrusarrow_forwardFor this exercise, you will be given a project file digital_pet containing two (2) classes, the DigiPet class andthe DigiPetTester class.Every DigiPet has five fields:- name- lifespan (in cycles) – max age- age (in cycles) – current age- mood (2 = joyful, 1 = happy, 0 = neutral, -1 = sad, -2 = angry)- size – current size of pet (size ≥ 1)A DigiPet has a default constructor, overloaded constructor, and some various methods which allow one tointeract with it or modify its state.Poke or pet increases or decreases its mood.Feed or exercise increases or decreases its size by a specified amount.Every time you interact with the digipet (poke, pet, feed, exercise), its age increases. When the age reaches thelifespan, it dies (or becomes a zombie).You need to modify and customize the DigiPet class as follows:- Implement the overloaded constructor: Initialize all five fields. Use the values passed as parameters toinitialize name and lifespan. Use the same default values that the default…arrow_forward
- JAVA Problem – CycleFileOutput Revisit the Cycle class. Modify your application such that the properties will be written to a text file called “Cycle.txt” instead of to the screen. Directions Examine your application for the class called Cycle. Add an appropriate throws statement in the main method. Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Use appropriate code to ensure that the text file exist. Output the values of the variables to the text file. Close the file. Note: Verify the contents were written to the text file using notepad (or any word processor). public class Cycle { // Declear integer instance variable private int numberOfWheels; private int weight; // Constructer declear class public Cycle(int numberOfWheels, int weight ) { this.numberOfWheels = numberOfWheels; this.weight = weight; } // String method for output public String toString() { String wheel = String.valueOf(this.numberOfWheels); String load =…arrow_forwardWrite a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardimplement a database about people, they could be made up, but you want to include a sizable number, maybe 10-20. The data that you store for each person must include a name and at least one additional piece of information, such as a birthday. You can assume that the names are unique. The database should enable you to enter, remove, modify, or search this data. You also should be able to save the data in a file for use later. Define a class Person to represent a person and another one to represent the database. A dictionary should be a data member of the database class, with Person objects as search keys for this dictionary. Write a program that tests and demonstrates your database.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
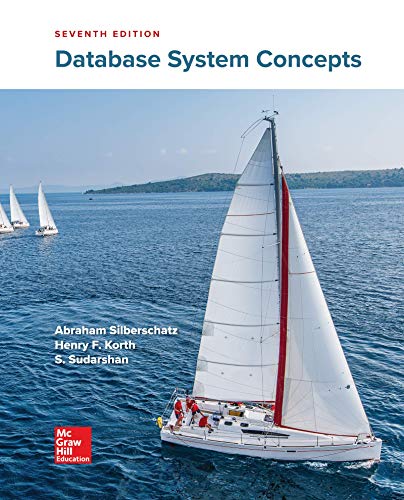
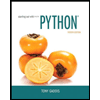
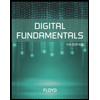
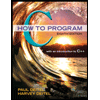
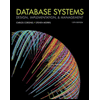
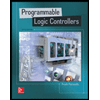