please edit this code so it does not contain breaks, while(true) and BufferedReader. Also keep getting this error " *** IOException *** phonebook.text (No such file or directory) the desired output has been given in the picture. here is the code to edit: import java.io.*; import java.util.Scanner; public class Phonebook { public static void main(String[] args) { int lookupCount = 0; int revLookupCount = 0; int phoneEntryCount = 0; Scanner inputReader = new Scanner(System.in); try { File file = new File("phonebook.txt"); BufferedReader phonebookReader; PhonebookEntry phonebookEntry[] = new PhonebookEntry[100]; phonebookReader = new BufferedReader(new FileReader(file)); String line = phonebookReader.readLine(); while (line != null) { if (phoneEntryCount >= 100) { throw new Exception( "Phonebook capacity exceeded - increase size of underlying array"); } phonebookEntry[phoneEntryCount++] = new PhonebookEntry(line); line = phonebookReader.readLine(); } phonebookReader.close(); mainLoop: while (true) { System.out.print("lookup, reverse-lookup, quit (l/r/q)? "); String option = inputReader.nextLine(); if (option.equalsIgnoreCase("l")) { lookupCount++; System.out.print("last name? "); String lName = inputReader.nextLine(); System.out.print("first name? "); String fName = inputReader.nextLine(); Name name = new Name(lName, fName); for (int i = 0; i < phoneEntryCount; i++) { PhonebookEntry aPhonebookEntry = phonebookEntry[i]; if (aPhonebookEntry.lookup(name) != null) { System.out.println( aPhonebookEntry.name + "'s phone number is " + aPhonebookEntry.phoneNumber ); continue mainLoop; } } System.out.println("--Name not found\n"); } else if (option.equalsIgnoreCase("r")) { revLookupCount++; System.out.print("Phone number (nnn-nnn-nnnn)? "); String phone = inputReader.nextLine(); PhoneNumber phoneNumber; try { phoneNumber = new PhoneNumber(phone); }catch (Exception e1){ System.out.println("*** Exception *** Phone number isn't correct."); continue; } for (int i = 0; i < phoneEntryCount; i++) { PhonebookEntry aPhonebookEntry = phonebookEntry[i]; if (aPhonebookEntry.reverseLookup(phoneNumber) != null) { System.out.println( phoneNumber + " belongs to " + aPhonebookEntry.name ); continue mainLoop; } } System.out.println("-- Phone number not found"); } else if (option.equalsIgnoreCase("q")) { System.out.println("" + lookupCount + " lookups performed."); System.out.println("" + revLookupCount + " reverse lookups performed."); break; } } } catch (FileNotFoundException e) { System.out.println("*** IOException *** phonebook.text (No such file or directory)"); } catch (Exception ex) { System.out.println("*** Exception *** " + ex); ex.printStackTrace(); } } } class Name { private String lastName; private String firstName; public Name(String lastName, String firstName) { this.lastName = lastName; this.firstName = firstName; } public String toString(){ return firstName + " " + lastName; } @Override public boolean equals(Object obj) { Name otherName = (Name) obj; return otherName.lastName.equalsIgnoreCase(lastName) && otherName.firstName.equalsIgnoreCase(firstName); } } class PhoneNumber { private int countryCode; private int areaCode; private int subscriberNumber; PhoneNumber(String phoneNumber) { String temp = phoneNumber.replace(")","-"); temp = temp.replace("(",""); String[] arr1 = temp.split("-"); countryCode = Integer.parseInt(arr1[0]); areaCode = Integer.parseInt(arr1[1]); subscriberNumber = Integer.parseInt(arr1[2]); } @Override public String toString() { return String.format("(%d)%d-%d",countryCode,areaCode,subscriberNumber); } @Override public boolean equals(Object obj) { PhoneNumber phoneNumber = (PhoneNumber) obj; return countryCode == phoneNumber.countryCode && areaCode == phoneNumber.areaCode && subscriberNumber == phoneNumber.subscriberNumber; } } class PhonebookEntry { Name name; PhoneNumber phoneNumber; PhonebookEntry(String entry){ String[] entrySplit = entry.split(" "); name = new Name(entrySplit[0],entrySplit[1]); phoneNumber = new PhoneNumber(entrySplit[2]); } String lookup(Name name1){ if(name.equals(name1)) return phoneNumber.toString(); return null; } String reverseLookup(PhoneNumber phoneNumber1){ if(phoneNumber1.equals(phoneNumber)) return name.toString(); return null; } }
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
please edit this code so it does not contain breaks, while(true) and
BufferedReader. Also keep getting this error " *** IOException *** phonebook.text (No such file or directory)
the desired output has been given in the picture.
here is the code to edit:
import java.io.*;
import java.util.Scanner;
public class Phonebook {
public static void main(String[] args) {
int lookupCount = 0;
int revLookupCount = 0;
int phoneEntryCount = 0;
Scanner inputReader = new Scanner(System.in);
try {
File file = new File("phonebook.txt");
BufferedReader phonebookReader;
PhonebookEntry phonebookEntry[] = new PhonebookEntry[100];
phonebookReader = new BufferedReader(new FileReader(file));
String line = phonebookReader.readLine();
while (line != null) {
if (phoneEntryCount >= 100) {
throw new Exception(
"Phonebook capacity exceeded - increase size of underlying array");
}
phonebookEntry[phoneEntryCount++] = new PhonebookEntry(line);
line = phonebookReader.readLine();
}
phonebookReader.close();
mainLoop:
while (true) {
System.out.print("lookup, reverse-lookup, quit (l/r/q)? ");
String option = inputReader.nextLine();
if (option.equalsIgnoreCase("l")) {
lookupCount++;
System.out.print("last name? ");
String lName = inputReader.nextLine();
System.out.print("first name? ");
String fName = inputReader.nextLine();
Name name = new Name(lName, fName);
for (int i = 0; i < phoneEntryCount; i++) {
PhonebookEntry aPhonebookEntry = phonebookEntry[i];
if (aPhonebookEntry.lookup(name) != null) {
System.out.println(
aPhonebookEntry.name + "'s phone number is " +
aPhonebookEntry.phoneNumber
);
continue mainLoop;
}
}
System.out.println("--Name not found\n");
}
else if (option.equalsIgnoreCase("r")) {
revLookupCount++;
System.out.print("Phone number (nnn-nnn-nnnn)? ");
String phone = inputReader.nextLine();
PhoneNumber phoneNumber;
try {
phoneNumber = new PhoneNumber(phone);
}catch (Exception e1){
System.out.println("*** Exception *** Phone number isn't correct.");
continue;
}
for (int i = 0; i < phoneEntryCount; i++) {
PhonebookEntry aPhonebookEntry = phonebookEntry[i];
if (aPhonebookEntry.reverseLookup(phoneNumber) != null) {
System.out.println(
phoneNumber + " belongs to " +
aPhonebookEntry.name
);
continue mainLoop;
}
}
System.out.println("-- Phone number not found");
} else if (option.equalsIgnoreCase("q")) {
System.out.println("" + lookupCount + " lookups performed.");
System.out.println("" + revLookupCount + " reverse lookups performed.");
break;
}
}
} catch (FileNotFoundException e) {
System.out.println("*** IOException *** phonebook.text (No such file or directory)");
} catch (Exception ex) {
System.out.println("*** Exception *** " + ex);
ex.printStackTrace();
}
}
}
class Name {
private String lastName;
private String firstName;
public Name(String lastName, String firstName) {
this.lastName = lastName;
this.firstName = firstName;
}
public String toString(){
return firstName + " " + lastName;
}
@Override
public boolean equals(Object obj) {
Name otherName = (Name) obj;
return otherName.lastName.equalsIgnoreCase(lastName)
&& otherName.firstName.equalsIgnoreCase(firstName);
}
}
class PhoneNumber {
private int countryCode;
private int areaCode;
private int subscriberNumber;
PhoneNumber(String phoneNumber) {
String temp = phoneNumber.replace(")","-");
temp = temp.replace("(","");
String[] arr1 = temp.split("-");
countryCode = Integer.parseInt(arr1[0]);
areaCode = Integer.parseInt(arr1[1]);
subscriberNumber = Integer.parseInt(arr1[2]);
}
@Override
public String toString() {
return String.format("(%d)%d-%d",countryCode,areaCode,subscriberNumber);
}
@Override
public boolean equals(Object obj) {
PhoneNumber phoneNumber = (PhoneNumber) obj;
return countryCode == phoneNumber.countryCode
&& areaCode == phoneNumber.areaCode
&& subscriberNumber == phoneNumber.subscriberNumber;
}
}
class PhonebookEntry {
Name name;
PhoneNumber phoneNumber;
PhonebookEntry(String entry){
String[] entrySplit = entry.split(" ");
name = new Name(entrySplit[0],entrySplit[1]);
phoneNumber = new PhoneNumber(entrySplit[2]);
}
String lookup(Name name1){
if(name.equals(name1))
return phoneNumber.toString();
return null;
}
String reverseLookup(PhoneNumber phoneNumber1){
if(phoneNumber1.equals(phoneNumber))
return name.toString();
return null;
}
}
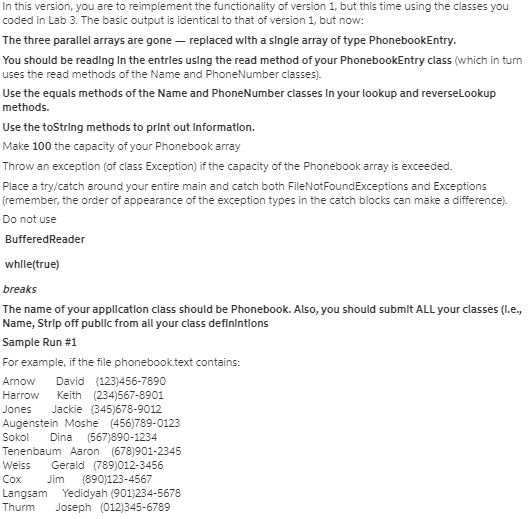
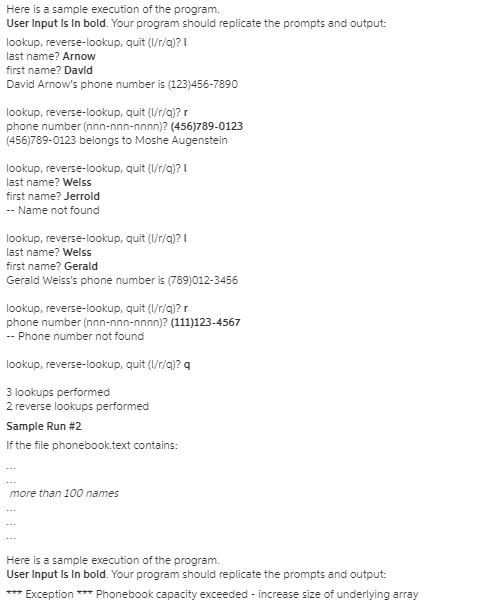

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

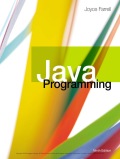
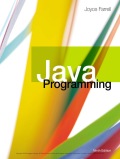