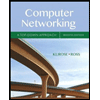
Need help making a java file that combines both linearSearch and binarySearch
•Both search methods must use the Comparable<T> interface and the compareTo() method.
•Your program must be able to handle different data types, i.e., use generics.
•For binarySearch, if you decide to use a midpoint computation formula that is different from
the textbook, explain that formula briefly as a comment within your code.
//code from textbook
//linearSearch
public static <T>
boolean linearSearch(T[] data, int min, int max, T target) {
int index = min;
boolean found = false;
while (!found && index <= max) {
found = data [index].equals(target);
index++;
}
return found;
}
//binarySearch
public static <T extends Comparable<T>>
boolean binarySearch(T[] data, int min, int max, T target) {
boolean found = false;
int midpoint = (min + max)/2;
if (data[midpoint].compareTo(target)==0)
found = true;
else if(data[midpoint].compareTo(target)>0) {
if(min<=midpoint-1)
found = binarySearch(data, min, midpoint-1, target);
}
else if (midpoint+1<=max)
found=binarySearch(data, midpoint+1, max, target);
return found;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Need help with this Java review If you can also send a screenshot will be helpful to understan Objective: The purpose of this lab exercise is to create a Singly Linked List data structure . Please note that the underlying implementation should follow with the descriptions listed below. Instructions : Create the following Linked List Data Structure in your single package and use "for loops" for your repetitive tasks. Task Check List ONLY "for" loops should be used within the data structure class. Names of identifiers MUST match the names listed in the description below. Deductions otherwise. Description The internal structure of this Linked List is a singly linked Node data structure and should have at a minimum the following specifications: data fields: The data fields to declare are private and you will keep track of the size of the list with the variable size and the start of the list with the reference variable data. first is a reference variable for the first Node in…arrow_forwardAssume that you need to organize the data of books. Existing books should be rearranged so that only one side of the shelf is empty, or new volumes should be placed there. In this case, what would be the best data structure? Make it possible to dynamically implement the process of adding books to the bookcase. Furthermore, what is the circumstance where fresh data need to be entered into a data structure, but there is no room?arrow_forwardIn java, the Arrays class contains a static binarySearch() method that returns …… if element is found. false true (-k–1) where k is the position before which the element should be inserted The index of the elementarrow_forward
- In C++, write a program that reads in an array of type int. You may assume that there are fewer than 20 entries in the array. The output must be a two-column list. The first column is a list of the distinct array elements and the second column is the count of the number of occurences of each element.arrow_forwardIn this task you will work with the linked list of digits we have created in the lessons up to this point. As before you are provided with some code that you should not modify: A structure definition for the storage of each digit's information. A main() function to test your code. The functions createDigit(), append(), printNumber(), freeNumber(), readNumber() and divisibleByThree() (although you may not need to use all of these). Your task is to write a new function changeThrees() which takes as input a pointer that holds the address of the start of a linked list of digits. Your function should change all of those digits in this linked list that equal 3 to the digit 9, and count how many replacements were made. The function should return this number of replacements. Provided codearrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forward
- Write a program to check whether a given word is a palindrome by using pointers A palindrome is a word that is the same when spelled from the front and from the back. For example, Kayak, Level, Racecar, Radar, Rotator Create 2 char arrays and 2 corresponding pointers to the arrays Initialize array1 to the word Copy array1 backwards to array2 Compare array1 and array2, if they are the same, the word is a palindrome Modify the pseudocode below to produce the sample output. char word[5]= "kayak"; char reverseWord[5]; char *f = word; char *b = reverseWord; for () *(b_from_start) = *(f_from_end); Copy word to reverseWord but starting from the back for() Compare word and reverseWord if same palindrome; Sample: word word_reverse word k word_reverse k а a e a k Palindrome? Yes e a Palindrome? Noarrow_forwardWrite a C++ application to linearly explore an array. Make array private.arrow_forwardWrite a java program that uses an ArrayList object to store the following set of names in memory: [Steve, Tim, Lucy, Pat, Angela, Tom] Now write some more code so that the same ArrayList object is augmented with the name 'Steve' after the name 'Lucy' After the ArrayList object has been augmented with the new name, display the original and new lists on the console (as shown below), to verify that the new name is positioned correctly in the list.arrow_forward
- Classes, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
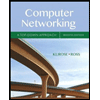
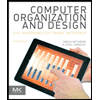
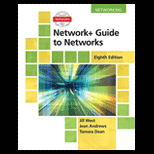
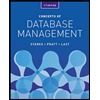
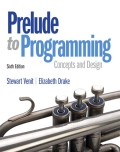
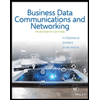