Here is the code I have written in Python but I need to make DRY using one single get_array function pulling in 5 times def display_common_items(plant_xml): plant_root = plant_xml.getroot() common_array = [] for item in plant_root.findall("PLANT"): common_array.append(item.find("COMMON").text) return common_array def display_botanical_items(plant_xml): plant_root = plant_xml.getroot() botanical_array = [] for item in plant_root.findall("PLANT"): botanical_array.append(item.find("BOTANICAL").text) return botanical_array def display_zone_items(plant_xml): plant_root = plant_xml.getroot() zone_array = [] for item in plant_root.findall("PLANT"): zone_array.append(item.find("ZONE").text) return zone_array def display_light_items(plant_xml): plant_root = plant_xml.getroot() light_array = [] for item in plant_root.findall("PLANT"): light_array.append(item.find("LIGHT").text) return light_array def display_price_items(plant_xml): plant_root = plant_xml.getroot() price_array = [] for item in plant_root.findall("PLANT"): price_array.append(item.find("PRICE").text) return price_array
Here is the code I have written in Python but I need to make DRY using one single get_array function pulling in 5 times
def display_common_items(plant_xml):
plant_root = plant_xml.getroot()
common_array = []
for item in plant_root.findall("PLANT"):
common_array.append(item.find("COMMON").text)
return common_array
def display_botanical_items(plant_xml):
plant_root = plant_xml.getroot()
botanical_array = []
for item in plant_root.findall("PLANT"):
botanical_array.append(item.find("BOTANICAL").text)
return botanical_array
def display_zone_items(plant_xml):
plant_root = plant_xml.getroot()
zone_array = []
for item in plant_root.findall("PLANT"):
zone_array.append(item.find("ZONE").text)
return zone_array
def display_light_items(plant_xml):
plant_root = plant_xml.getroot()
light_array = []
for item in plant_root.findall("PLANT"):
light_array.append(item.find("LIGHT").text)
return light_array
def display_price_items(plant_xml):
plant_root = plant_xml.getroot()
price_array = []
for item in plant_root.findall("PLANT"):
price_array.append(item.find("PRICE").text)
return price_array

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

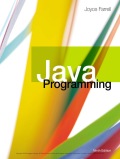
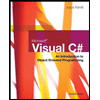
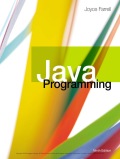
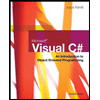