heritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty, False otherwise [] – return the item at a given location, [0] is at the bottom
Use Python for this question. Also please comment what each line of code means for this question as well:
- Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!)
- Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top.
- push() – take an item as input and push it on the top of the stack
- pop() – remove and return the item at the top of the stack
- isEmpty() – returns True if the stack is empty, False otherwise
- [] – return the item at a given location, [0] is at the bottom of the stack
- len() – return length of the stack
Output for this problem:
##### Stack #####
>>> s = Stack()
>>> len( vars(s)) # really inheriting? failure on this test means Stack is probably not properly inheriting
0
>>> isinstance(s,list) # inheriting?
True
>>> s = Stack()
>>> s.push('apple')
>>> s
Stack(['apple'])
>>> s.push('pear')
>>> s.push('kiwi')
>>> s
Stack(['apple', 'pear', 'kiwi'])
>>> top = s.pop()
>>> top
'kiwi'
>>> s
Stack(['apple', 'pear'])
>>> len(s)
2
>>> s.isEmpty()
False
>>> s.pop()
'pear'
>>> s.pop()
'apple'
>>> s.isEmpty()
True
>>> s = Stack(['apple', 'pear', 'kiwi'])
>>> s = Stack(['apple', 'pear', 'kiwi'])
>>> s[0]
'apple'
>>>
if you receive an error on s2 then you are probably not calling the list
constructor init.
>>> s = Stack()
>>> s.push('apple')
>>> s
Stack(['apple'])
>>> s2 = Stack()
>>> s2.push('pear')
>>> s2 # if this fails - see the TEST file for explanation
Stack(['pear'])
check that the Stack is really inheriting from list
>>> s = Stack()
>>> isinstance(s, list)
True
>>> len( vars(s) )==0
True

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

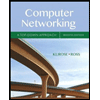
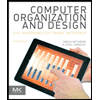
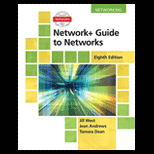
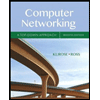
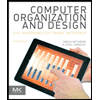
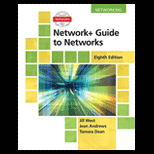
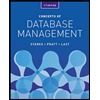
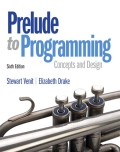
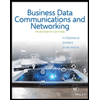