Question 1. Inheritance: You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using inheritance. inherit the following methods below: a. Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. b. push() - take an item as input and push it on the top of the stack c. pop() - remove and return the item at the top of the stack d. isEmpty() - returns True if the stack is empty, False otherwise e. [] - return the item at a given location, [0] is at the bottom of the stack f. len() - return length of the stack Output for Question 1: ##### Stack ##### >>> s = Stack() >>> len( vars(s)) # really inheriting? failure on this test means Stack is probably not properly inheriting 0 >>> isinstance(s,list) # inheriting? True >>> s = Stack() >>> s.push('apple') >>> s Stack(['apple']) >>> s.push('pear') >>> s.push('kiwi') >>> s Stack(['apple', 'pear', 'kiwi']) >>> top = s.pop() >>> top 'kiwi' >>> s Stack(['apple', 'pear']) >>> len(s) 2 >>> s.isEmpty() False >>> s.pop() 'pear' >>> s.pop() 'apple' >>> s.isEmpty() True >>> s = Stack(['apple', 'pear', 'kiwi']) >>> s = Stack(['apple', 'pear', 'kiwi']) >>> s[0] 'apple' >>> if you receive an error on s2 then you are probably not calling the list constructor init. >>> s = Stack() >>> s.push('apple') >>> s Stack(['apple']) >>> s2 = Stack() >>> s2.push('pear') >>> s2 # if this fails - see the TEST file for explanation Stack(['pear']) check that the Stack is really inheriting from list >>> s = Stack() >>> isinstance(s, list) True >>> len( vars(s) )==0 True Question 2: Write a client function parenthesesMatch that given a string containing only the characters for parentheses, braces or curly braces, i.e., the characters in '([{}])', returns True if the parentheses, brackets and braces match and False otherwise. Your solution must use a Stack. Output for Question 2: ##### parenthesesMatch ##### >>> parenthesesMatch('(){}[]') True >>> parenthesesMatch('{[()]}') True >>> parenthesesMatch('((())){[()]}') True >>> parenthesesMatch('(}') False >>> parenthesesMatch(')(][') # right number, but out of order False >>> parenthesesMatch('([)]') # right number, but out of order False >>> parenthesesMatch('({])') False >>> parenthesesMatch('((())') False >>> parenthesesMatch('(()))') False
Please use Python for this question:
Question 1. Inheritance: You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using inheritance.
inherit the following methods below:
a. Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top.
b. push() - take an item as input and push it on the top of the stack
c. pop() - remove and return the item at the top of the stack
d. isEmpty() - returns True if the stack is empty, False otherwise
e. [] - return the item at a given location, [0] is at the bottom of the stack
f. len() - return length of the stack
Output for Question 1:
##### Stack #####
>>> s = Stack()
>>> len( vars(s)) # really inheriting? failure on this test means Stack is probably not properly inheriting
0
>>> isinstance(s,list) # inheriting?
True
>>> s = Stack()
>>> s.push('apple')
>>> s
Stack(['apple'])
>>> s.push('pear')
>>> s.push('kiwi')
>>> s
Stack(['apple', 'pear', 'kiwi'])
>>> top = s.pop()
>>> top
'kiwi'
>>> s
Stack(['apple', 'pear'])
>>> len(s)
2
>>> s.isEmpty()
False
>>> s.pop()
'pear'
>>> s.pop()
'apple'
>>> s.isEmpty()
True
>>> s = Stack(['apple', 'pear', 'kiwi'])
>>> s = Stack(['apple', 'pear', 'kiwi'])
>>> s[0]
'apple'
>>>
if you receive an error on s2 then you are probably not calling the list
constructor init.
>>> s = Stack()
>>> s.push('apple')
>>> s
Stack(['apple'])
>>> s2 = Stack()
>>> s2.push('pear')
>>> s2 # if this fails - see the TEST file for explanation
Stack(['pear'])
check that the Stack is really inheriting from list
>>> s = Stack()
>>> isinstance(s, list)
True
>>> len( vars(s) )==0
True
Question 2: Write a client function parenthesesMatch that given a string containing only the characters for parentheses, braces or curly braces, i.e., the characters in '([{}])', returns True if the parentheses, brackets and braces match and False otherwise. Your solution must use a Stack.
Output for Question 2:
##### parenthesesMatch #####
>>> parenthesesMatch('(){}[]')
True
>>> parenthesesMatch('{[()]}')
True
>>> parenthesesMatch('((())){[()]}')
True
>>> parenthesesMatch('(}')
False
>>> parenthesesMatch(')(][') # right number, but out of order
False
>>> parenthesesMatch('([)]') # right number, but out of order
False
>>> parenthesesMatch('({])')
False
>>> parenthesesMatch('((())')
False
>>> parenthesesMatch('(()))')
False

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

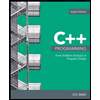
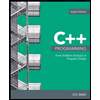