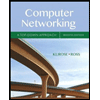
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
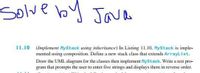
Transcribed Image Text:Solve by Jaa
11.10 (Implement Mystack using inheritance) In Listing 11.10, flyStack is imple-
mented using composition. Define a new stack class that extends Arraylist.
Draw the UML diagram for the classes then implement MyStack. Write a test pro-
gram that prompts the user to enter five strings and displays them in reverse order.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Implement the copy constructor for the StringVar class using the options given on the right side window. Place the code into the left side using the arrows. NOTE: Be careful! There are decoys! Assume that StringVar.h has the following declaration: #include <iostream> class StringVar {public:StringVar() : max_length(20) { // Default constructor size is 20value = new char[max_length+1];value[0] = '\0';}StringVar(int size); // Takes an int for sizeStringVar(const char cstr[]); // Takes a c-string and copies itStringVar(const StringVar& strObj); // Copy Constructor~StringVar(); // Destructorint size() const { return max_length; } // Access capacityconst char* c_str() const { return value; } // Access valueint length() const { return strlen(value); } // Access lengthStringVar& operator= (const StringVar& rightObj);std::istream& operator>> (std::istream& in, StringVar& strVar);std::ostream& operator<< (std::ostream& out, const StringVar&…arrow_forwardWe have a parking office class for an object-oriented parking management system using java Implement the following methods for our class Add equals and hashCode methods to any class used in a List. Add a method to the Parking Office to return a collection of customer ids (getCustomerIds) Add a method to the Parking Office to return a collection of permit ids (getPermitIds) Add a method to the Parking Office to return the collection of permit ids for a specific customer (getPermitIds(Customer)) The above methods are not included in the parking office class of our class diagram. I have attached class diagrams with definitions of related classes in our system (i.e car, customer, .....)arrow_forwardSuppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.Code: #include <iostream>#include <queue>#include <string>#include <utility> using namespace std; class Stack{public: Stack(); string top(); void pop(); void push(string element);private: int count; priority_queue<pair<int, string>> pqueue;}; Stack::Stack(){ /* Your code goes here */} string Stack::top(){ /* Your code goes here */} void Stack::pop(){ /* Your code goes here */} void Stack::push(string element){ /* Your code goes here */} int main(){ Stack…arrow_forward
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardIn this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forwardHi, I am a bit lost on how to do this coding assignment. It would be great if someone could help me fill in the MyStack.java file with the given information. All the information is in the pictures. Thank you!arrow_forward
- Write a Java program for a matrix class that can add and multiply arbitrary two dimensional arrays of integers. Textbook Project P-3.36, pp. 147 Implement Singly Linked List - use textbook Chapter 3.2 as an exaple. Write a main driver to test basic list implementations. Textbook reference : Data structures and algorithms in Java Micheal Goodricharrow_forwardpublic class PokerAnalysis implements PokerAnalyzer { privateList<Card>cards; privateint[]rankCounts; privateint[]suitCounts; /** * The constructor has been partially implemented for you. cards is the * ArrayList where you'll be adding all the cards you're given. In addition, * there are two arrays. You don't necessarily need to use them, but using them * will be extremely helpful. * * The rankCounts array is of the same length as the number of Ranks. At * position i of the array, keep a count of the number of cards whose * rank.ordinal() equals i. Repeat the same with Suits for suitCounts. For * example, if your Cards are (Clubs 4, Clubs 10, Spades 2), your suitCounts * array would be {2, 0, 0, 1}. * * @param cards * the list of cards to be added */ publicPokerAnalysis(List<Card>cards){ this.cards=newArrayList<Card>(); this.rankCounts=newint[Rank.values().length]; this.suitCounts=newint[Suit.values().length];…arrow_forwardin Javaarrow_forward
- Add missing code to turn this class a singleton in Java package com.gamingroom; import java.util.ArrayList;import java.util.List; /** * A singleton service for the game engine */public class GameService { /** * A list of the active games */ private static List<Game> games = new ArrayList<Game>(); /* * Holds the next game identifier */ private static long nextGameId = 1; // FIXME: Add missing pieces to turn this class a singleton /** * Construct a new game instance * * @param name the unique name of the game * @return the game instance (new or existing) */ public Game addGame(String name) { // a local game instance Game game = null; // FIXME: Use iterator to look for existing game with same name // if found, simply return the existing instance // if not found, make a new game instance and add to list of games if (game == null) { game = new Game(nextGameId++, name); games.add(game); } // return the new/existing game instance to the caller return…arrow_forwardGiven that an ArrayList of Strings named friendList has already been created and names have already been inserted, write the statement necessary to change the name "Tyler", stored at index 8, to "Bud". This is needed in Javaarrow_forwardGiven the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
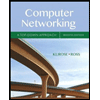
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
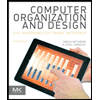
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
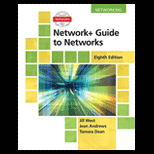
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
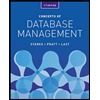
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
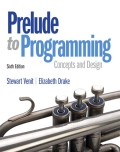
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
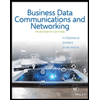
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY