Tie-Tac-Toe Problem Description: Tic Tac Toe game is traditionally played on a 3x3 board bewoen two players x and o. A player can mark a single cell by placing a symbol in it (either an x'or an w character). A player who manages to mark a row, column, or a diagonal completely with his symbol fiest wins the game. The game ends in a tie if no player manages to win after all the cells have boen marked. You will design a textal version of this game, in which a human player gets to play against a computer opponent. Program Specification: In this project, you will implement a complete Tio-Tac-Toe game in a Java program. Your implementation should follow this specification. You may use one or two dimensional amay as data structure for the game bourd. Your program should include the following list of methods: 1. initloard ()- update this method This function initializes the game board. There are 9 cells represenied by 1 oc 2 dimensional amay. 2. gameloard ()- update this method This method prints the game boand. 3. checkWinner ()- update this method This method examines the array to see if either player has won. A player has won if he has marked a row, oolumn or diagonal with his symbol. The fimnction retums different values based on results of winner, loser and no winner. 4. void yourTum() This method handles moves made by the wuser. It displays the game board and asks the user to eater a move. If the move is invalid, in peompts user to eater a valid move again. A valid move sefers to one of the empty cells in the board. 5. void machine lum ) This method handles moves by the oompuler. You can implement a simple logic where you just search for an empty position in the array and mark it for computer player. You can get really creative and design the method so that it makes a well thought out move. The logic is entirely up to you. Finally, you should display the ocomputer's move. 6 main ) The main method controls the game. You will declare an amay for the game and make e of the methods that you have writen to produce behavior of the Tie-Tae-Toe game. The human player always makes the finst move in the game. You should alternate hetwoen the human player and the computer. After each move you should check for a wimer using the checkWinner method. While there is no winner, you should display the penchoard, ak the ser for nest valid mowe, update computer move until one of player wim or the gme is tie. Easy vernsion: human vs. human (machineTum method will he skipped) Challenging version: human vs. smant computer (ie. computer can't he heaten) Sample Output: ter dinate le tahi
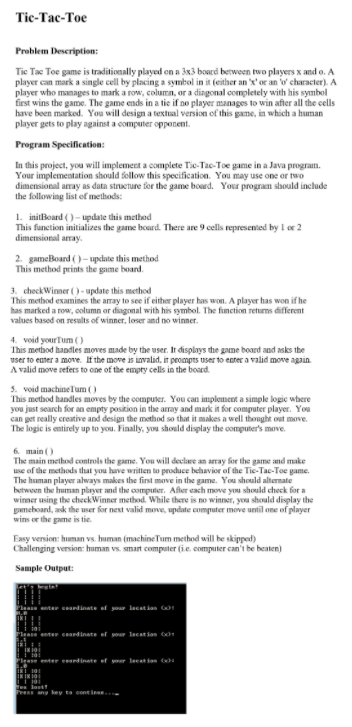

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Hi I like this code but is it possible to incorportate the 3 methods, because i dont see it in the current code -
void yourTurn ( ) - This method handles moves made by the user. It displays the game board and asks the user to enter a move. If the move is invalid, it prompts user to enter a valid move again. A valid move refers to one of the empty cells in the board.
void machineTurn ( ) - This method handles moves by the computer. One possibility is to search for an empty position in the array and mark it for the computer player
main ( ) - The main method controls the game. You will declare an array for the game and make use of the methods that you have written to produce behavior of the Tic-Tac-Toe game. The human player always makes the first move in the game. You should alternate between the human player and the computer. After each move you should check for a winner using the checkWinner method. While there is no winner, you should display the gameboard, ask the user for next valid move, update computer move until one of player wins or the game is tie.
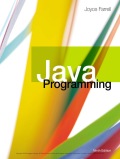
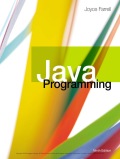