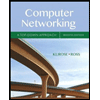
C++ Coding Assignment: Redo the 8 queens 1-dimensional array
GIVEN C++ CODE - WITH GOTO's:
#include <iostream>
using namespace std;
int main() {
int q[8], c=0;
q[0] = 0; // place a queen in row 0 of column 0
int counter = 0; // to count solutions
// c: column as q[c]: row in column c
next_col:
++c; // or c++;
// next column
if(c==8) goto print;
q[c]=-1; // 0
next_row:
++q[c]; // or q[c]++;
// next row
if(q[c]==8) goto backtrack;
for(int i=0; i<c; ++i)
if(q[i]==q[c] || (c-i)== abs(q[c]-q[i])) // || = row test OR diagonal tests
goto next_row;
goto next_col;
backtrack:
--c; // or c--
if(c==-1) // once we backtrack to column -1, we have all the solutions.
return 0; // ends the program
goto next_row;
print:
counter++; // new solutions
cout << counter << ":" << endl;
for(int i=0; i<8; i++)
cout << q[i];
cout << "\n" << endl;
goto backtrack;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- C++arrow_forwardTask using C language Write a program that checks both of the following conditions hold for a given array a1, a2, …, an. If the conditions hold, display “yes”, otherwise, display “no”. a1 < a2 < … < an For every i from 2 to n, ai isn’t divisible by ai-1. Requirements Follow the format of the examples below. The program reads in the number of elements of the array, for example, 4, then read in the numbers in the array, for example, 3 6 8 9. In the main function, declare the input array after reading in the number of elements of the array, then read in the elements. Examples (your program must follow this format precisely) Example #1 Enter the length of the input array: 7Enter the array elements: 2 5 4 1 6 9 12Output: no Example #2 Enter the length of the input array: 6Enter the array elements: 2 7 13 19 23 43Output: yesarrow_forwardC++ program: Write a function that accepts an array/vector as an input and calculate the sum of all the elements in the array. This function must use recursion to achieve this. Show code: Please show all steps.arrow_forward
- PYTHON 3.7 CODELABarrow_forwardGiven an integer array of positive single digit values such as:int a[] = {8,4,2,6,9};1) Write a recursive arrayToN function which returns the concatenation of all array values as an integer value.This function should accept all required data as parameters and return a long integer value.ExamplesIf the array is 8 4 2 6 9 the arrayToN function returns the integer 84269.If the array is 0 2 6 8 9 3 5 1 the arrayToN function returns the integer 2689351.arrow_forwardUsing C++ Language Need help on below code, I have the code but need to compute maximum of the array. Code: #include<iostream> using namespace std; int main() { int size; cout<<" Enter array size: "; cin>>size; int *numList=new int[size]; cout<<"Enter "<<size<<" elements: "; for(int i=0;i<size;i++) { cin>> *(numList+i); } delete numList; return 0; }arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
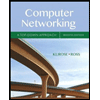
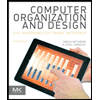
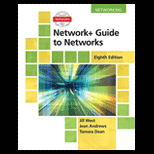
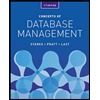
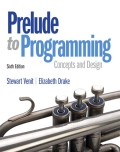
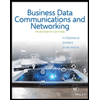