I am receiving a NameError even though I though I had defined 'randomInt'. How would I remove this error? (Using Python)
I am receiving a NameError even though I though I had defined 'randomInt'. How would I remove this error? (Using Python)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I am receiving a NameError even though I though I had defined 'randomInt'. How would I remove this error?
(Using Python)
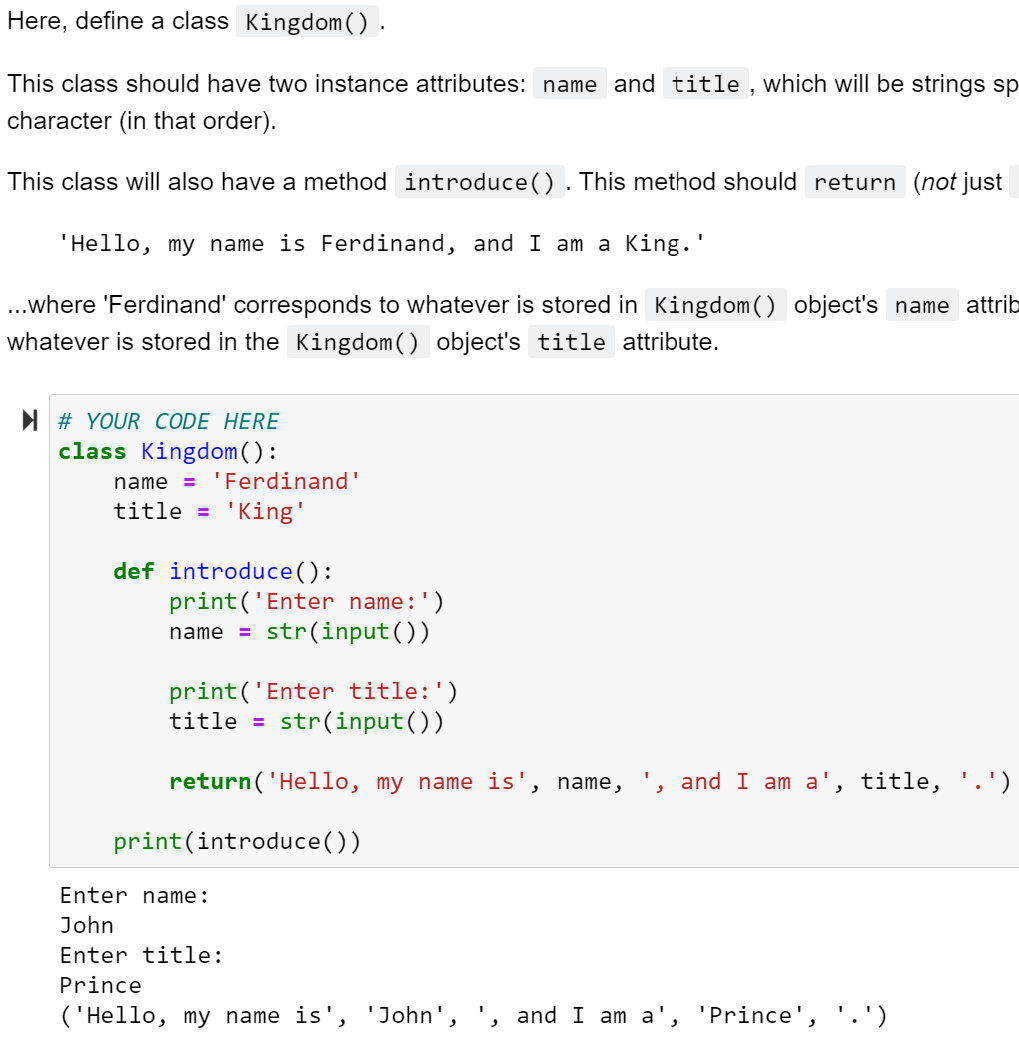
Transcribed Image Text:Here, define a class Kingdom().
This class should have two instance attributes: name and title , which will be strings sp
character (in that order).
This class will also have a method introduce(). This method should return (not just
'Hello, my name is Ferdinand, and I am a King.'
..where 'Ferdinand' corresponds to whatever is stored in Kingdom() object's name attrib
whatever is stored in the Kingdom() object's title attribute.
I # YOUR CODE HERE
class Kingdom():
name = 'Ferdinand'
title =
'King'
def introduce():
print('Enter name:')
name =
str(input())
print('Enter title:')
str(input())
title =
return('Hello, my name is', name,
, and I am a', title,
.')
print(introduce())
Enter name:
John
Enter title:
Prince
('Hello, my name is', 'John',
and I am a', 'Prince'
![This object should inherit all attributes and methods from Kingdom().
Additionally, the CourtJester() object should have:
• a class attribute headwear , storing the value 'fool's cap'
• a method tell_a_joke() that should return a joke at random from the list provided below
(Reminder: You imported the random module earlier. Use of a function from that module will likely be helpful in
tell_a_joke().)
N # joke list provided
['A clown held the door open for me yesterday. I thought it was a nice jester',
'How does the court jester address the King of Ducks? Mal’Lard',
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparent
'What do you call a joke made by using sign language? A jester']
0]: ['A clown held the door open for me yesterday. I thought it was a nice jester',
'How does the court jester address the King of Ducks? Mal’Lard'
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparen
t',
'What do you call a joke made by using sign language? A jester']
I # YOUR CODE HERE
class CourtJester(Kingdom):
headwear = "fool's cap"
jokelist
'How does the court jester address the King of Ducks? Mal’Lard',
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparent
'What do you call a joke made by using sign language? A jester']
['A clown held the door open for me yesterday. I thought it was a nice jester',
def tell_a_joke(self):
randomInt = random.randint (0, len(self.jokelist) - 1)
return jokelist[randomInt]
print(jokelist[randomInt])
NameError
Traceback (most recent call last)
<ipython-input-53-8a761d92414e> in <module>
1 # YOUR CODE HERE
-> 2 class CourtJester(Kingdom):
headwear = "fool's cap"
jokelist
4
['A clown held the door open for me yesterday. I thought it was a nice jest
%3D
er',
'How does the court jester address the King of Ducks? MalLard',
<ipython-input-53-8a761d92414e> in CourtJester()
return jokelist[randomInt]
11
12
---> 13
print(jokeList[randomInt])
NameError: name 'randomInt' is not defined](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3200e892-7b83-4670-8aa5-c4c84f2a6adb%2F04c7a164-81e9-4edb-a3e3-7c128350db6a%2Fltdmxn_processed.png&w=3840&q=75)
Transcribed Image Text:This object should inherit all attributes and methods from Kingdom().
Additionally, the CourtJester() object should have:
• a class attribute headwear , storing the value 'fool's cap'
• a method tell_a_joke() that should return a joke at random from the list provided below
(Reminder: You imported the random module earlier. Use of a function from that module will likely be helpful in
tell_a_joke().)
N # joke list provided
['A clown held the door open for me yesterday. I thought it was a nice jester',
'How does the court jester address the King of Ducks? Mal’Lard',
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparent
'What do you call a joke made by using sign language? A jester']
0]: ['A clown held the door open for me yesterday. I thought it was a nice jester',
'How does the court jester address the King of Ducks? Mal’Lard'
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparen
t',
'What do you call a joke made by using sign language? A jester']
I # YOUR CODE HERE
class CourtJester(Kingdom):
headwear = "fool's cap"
jokelist
'How does the court jester address the King of Ducks? Mal’Lard',
'What did the court jester call the balding crown prince? The Heir Apparent with no Hair Apparent
'What do you call a joke made by using sign language? A jester']
['A clown held the door open for me yesterday. I thought it was a nice jester',
def tell_a_joke(self):
randomInt = random.randint (0, len(self.jokelist) - 1)
return jokelist[randomInt]
print(jokelist[randomInt])
NameError
Traceback (most recent call last)
<ipython-input-53-8a761d92414e> in <module>
1 # YOUR CODE HERE
-> 2 class CourtJester(Kingdom):
headwear = "fool's cap"
jokelist
4
['A clown held the door open for me yesterday. I thought it was a nice jest
%3D
er',
'How does the court jester address the King of Ducks? MalLard',
<ipython-input-53-8a761d92414e> in CourtJester()
return jokelist[randomInt]
11
12
---> 13
print(jokeList[randomInt])
NameError: name 'randomInt' is not defined
Expert Solution

Step 1: explanation:
The reason you are getting this error is because print function is in the class and not in the function.
what's happening now is:
1. class is called
2. it is asking to print a random joke from jokelist
3. it found the jokelist in main but randomint is not in the class. it is defined in function.
that is why you are getting the NameError.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
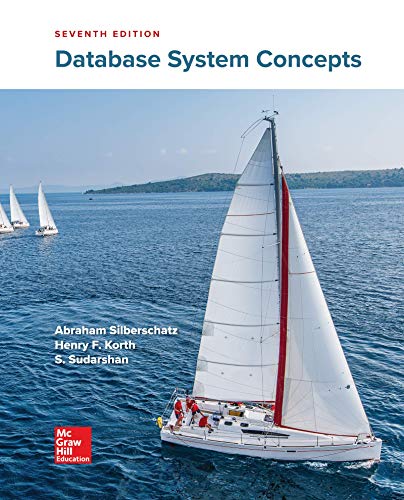
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
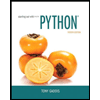
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
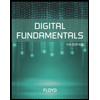
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
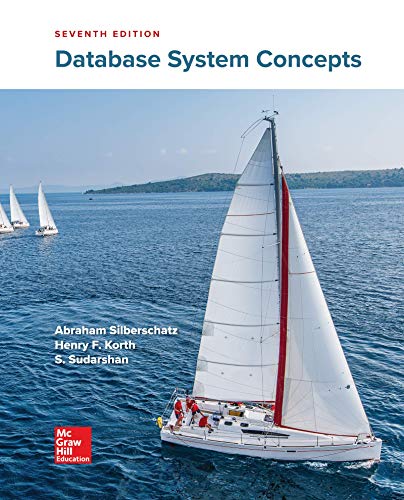
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
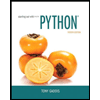
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
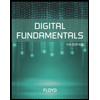
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
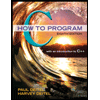
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
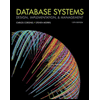
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
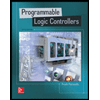
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education