Using java, answer the following questions about the code below. 1) Create a new function or method that checks to see if an int parameter is present in the list. 2) Create a main() method or JUnit tests to show that your list code functions as intended. (Code) private class Node int data; Node next; Node(int data) { this.data = data; this.next = null; } } private Node first; private Node last; public void addToEnd(int data){ Node newNode = new Node(data); if (first == null){ first = newNode; last = newNode; } else { last.next = newNode; last = newNode; } } public void printList(){ Node current = first; while (current != null){ System.out.print(current.data + " "); current = current.next; } System.out.println(); } public static void main(String[] args){ node list = new node(); list.addToEnd(2); list.addToEnd(4); list.addToEnd(6); list.printList(); } }
Using java, answer the following questions about the code below. 1) Create a new function or method that checks to see if an int parameter is present in the list. 2) Create a main() method or JUnit tests to show that your list code functions as intended. (Code) private class Node int data; Node next; Node(int data) { this.data = data; this.next = null; } } private Node first; private Node last; public void addToEnd(int data){ Node newNode = new Node(data); if (first == null){ first = newNode; last = newNode; } else { last.next = newNode; last = newNode; } } public void printList(){ Node current = first; while (current != null){ System.out.print(current.data + " "); current = current.next; } System.out.println(); } public static void main(String[] args){ node list = new node(); list.addToEnd(2); list.addToEnd(4); list.addToEnd(6); list.printList(); } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 5PE
Related questions
Question
Using java, answer the following questions about the code below.
1) Create a new function or method that checks to see if an int parameter is present in the list.
2) Create a main() method or JUnit tests to show that your list code functions as intended.
(Code)
private class Node
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
private Node first;
private Node last;
public void addToEnd(int data){
Node newNode = new Node(data);
if (first == null){
first = newNode;
last = newNode;
} else {
last.next = newNode;
last = newNode;
}
}
public void printList(){
Node current = first;
while (current != null){
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
public static void main(String[] args){
node list = new node();
list.addToEnd(2);
list.addToEnd(4);
list.addToEnd(6);
list.printList();
}
}
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
private Node first;
private Node last;
public void addToEnd(int data){
Node newNode = new Node(data);
if (first == null){
first = newNode;
last = newNode;
} else {
last.next = newNode;
last = newNode;
}
}
public void printList(){
Node current = first;
while (current != null){
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
public static void main(String[] args){
node list = new node();
list.addToEnd(2);
list.addToEnd(4);
list.addToEnd(6);
list.printList();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
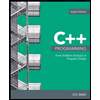
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
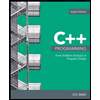
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning