Please fix the errors in this code to make it have an output similar to this: Here is the C code to be fixed: #include int Read(int, int, int [][10], int [][10],int []
Please fix the errors in this code to make it have an output similar to this:
Here is the C code to be fixed:
#include<stdio.h>
int Read(int, int, int [][10], int [][10],int [],int []);
int *Process(int, int, int [][10], int [][10],int [],int [], int []);
void Display (int, int[], int, int[], int [], int [][10]);
static int mark[20];
int i,j,np,nr;
int alloc[10][10],request[10][10],avail[10],r[10],w[10];
int main()
{
Read (np, nr, alloc[10], request[10], avail[], r[]);
Process (np, nr, alloc[10], request[10],avail[],w[], mark[]);
Display (np, request[10],nr, mark[], r[], alloc[10]);
return 0;
}
int Read(int np, int nr, int alloc[][10], int request[][10],int avail[],int r[])
{
int i,j;
FILE* file = fopen ("input.txt", "r");
// get the no of process
fscanf (file, "%d", &np);
// get the no of resources
fscanf (file, "%d", &nr);
// get availale resources
for(i=0;i<nr;i++)
{
fscanf (file, "%d", &r[i]);
}
// get the allocation matrix
for(i=0;i<np;i++)
for(j=0;j<nr;j++)
fscanf (file, "%d", &alloc[i][j]);
// get the request matrix
for(i=0;i<np;i++)
for(j=0;j<nr;j++)
fscanf (file, "%d", &request[i][j]);
fclose (file);
return request,alloc,np,nr;
}
int *Process(int np, int nr, int alloc[][10], int request[][10],int avail[],int w[], int mark[])
{
int i,j,count=0;
for(i=0;i<np;i++)
{
for(j=0;j<nr;j++)
{
if(alloc[i][j]==0)
count++;
else
break;
}
if(count==nr)
mark[i]=1;
}
for(j=0;j<nr;j++)
w[j]=avail[j];
//mark processes with request less than or equal to W
for(i=0;i<np;i++)
{
int canbeprocessed=0;
if(mark[i]!=1)
{
for(j=0;j<nr;j++)
{
if(request[i][j]<=w[j])
canbeprocessed=1;
else
{
canbeprocessed=0;
break;
}
}
if(canbeprocessed)
{
mark[i]=1;
for(j=0;j<nr;j++)
w[j]+=alloc[i][j];
}
}
}
return w;
}
void Display(int np, int request[][10], int nr, int mark, int r[], int alloc[][10])
{
int i,j,deadlock=0;
printf("Numer of processes: %d",np);
printf("\n");
printf("Numer of resources: %d",nr);
printf("\n");
printf("\nAvailbaility
for(i=0;i<nr;i++)
{
printf("%d ",r[i]);
}
printf("\n");
printf("\nAllocation Matrix\n");
for(i=0;i<np;i++)
{
for(j=0;j<nr;j++)
printf("%d ", alloc[i][j]);
printf("\n");
}
printf("\n");
printf("Request Matrix\n");
for(i=0;i<np;i++)
{
for(j=0;j<nr;j++)
printf("%d ", request[i][j]);
printf("\n");
}
printf("\n");
for(i=0;i<np;i++)
{
if(mark[i]!=1)
deadlock=1;
if(deadlock)
printf("\nThere is a Deadlock");
else
printf("\nThere is a no Deadlock");
}
return;
}
For Basis:
#include<stdio.h>
static int mark[20];
int i,j,np,nr;
int main(){
int alloc[10][10],request[10][10],avail[10],r[10],w[10];
// open the input file
// use fscanf (file, "%d", &x) to read integer from file
FILE* file = fopen ("input.txt", "r");
// get the no of process
fscanf (file, "%d", &np);
// get the no of resources
fscanf (file, "%d", &nr);
// get availale resources
for(i=0;i<nr;i++){
fscanf (file, "%d", &r[i]);
}
// get the allocation matrix
for(i=0;i<np;i++)
for(j=0;j<nr;j++)
fscanf (file, "%d", &alloc[i][j]);
// get the request matrix
for(i=0;i<np;i++)
for(j=0;j<nr;j++)
fscanf (file, "%d", &request[i][j]);
// print all the details read from input to console
printf("Numer of processes: %d",np);
printf("\n");
printf("Numer of resources: %d",nr);
printf("\n");
printf("\nAvailbaility vector\n");
for(i=0;i<nr;i++){
printf("%d ",r[i]);
}
printf("\n");
printf("\nAllocation Matrix\n");
for(i=0;i<np;i++){
for(j=0;j<nr;j++)
printf("%d ", alloc[i][j]);
printf("\n");
}
printf("\n");
printf("Request Matrix\n");
for(i=0;i<np;i++){
for(j=0;j<nr;j++)
printf("%d ", request[i][j]);
printf("\n");
}
printf("\n");
//marking processes with zero allocation
for(i=0;i<np;i++){
int count=0;
for(j=0;j<nr;j++){
if(alloc[i][j]==0)
count++;
else
break;
}
if(count==nr)
mark[i]=1;
}
// initialize W with avail
for(j=0;j<nr;j++)
w[j]=avail[j];
//mark processes with request less than or equal to W
for(i=0;i<np;i++){
int canbeprocessed=0;
if(mark[i]!=1){
for(j=0;j<nr;j++){
if(request[i][j]<=w[j])
canbeprocessed=1;
else{
canbeprocessed=0;
break;
}
}
if(canbeprocessed){
mark[i]=1;
for(j=0;j<nr;j++)
w[j]+=alloc[i][j];
}
}
}
//checking for unmarked processes
int deadlock=0;
for(i=0;i<np;i++)
if(mark[i]!=1)
deadlock=1;
if(deadlock)
printf("\nThere is a Deadlock");
else
printf("\nThere is a no Deadlock");
fclose (file);
}
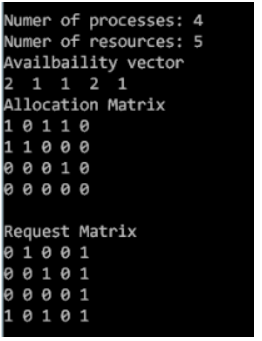

Step by step
Solved in 2 steps

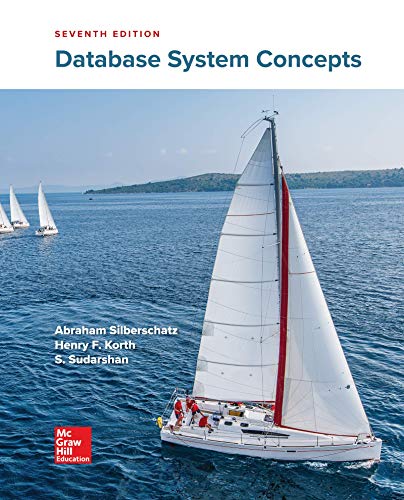
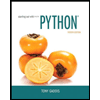
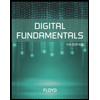
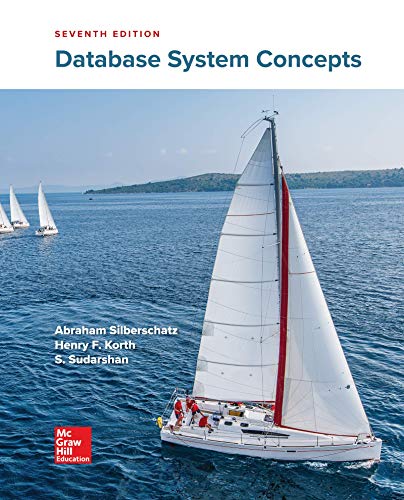
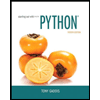
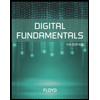
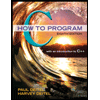
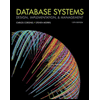
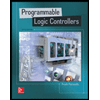