I need help In C This program calculates and prints the total grades for students out of 100; then, it prints the number of students who got A’s, B’s, C’s, D’s, and F’s. This program must implement the following functions: double get_total(double grades[], int size); This function receives a list of grades in the grades[] array and its size in the variable size. This function must calculate the total of the grades in grades[]. However, the function must drop the lowest quiz grade before calculating the total. Each grades[] list has the following elements order: {[Lab Exam/20] [Quiz 1/5] [Quiz 2/5] [Quiz 3/5] [Mid Exam/20] [Final Exam/50]}. The function will remove the lowest Quiz grade, and then it will precede to sum the rest of the grade. The total must not exceed 100. For example, if you pass 15, 5, 5, 3, 19, 46 to the function, the function will remove the three, and you will get 90. Another example, 17, 3, 1, 2, 17, 45, the function will return 84. char get_letter_grade(double *grade); This function receives a pointer to a grade and returns A if the grade is greater or equal to 90. B if the grade is less than 90 and greater than or equal to 80. C if the grade is less than 80 and greater or equal to 70. D if the grade is less than 70 and greater than or equal to 60. Finally, F if the grade is less than 60. grades_s get_distribution(double totals[], int totals_size); The function accepts a list of total grades totals[] for a number of students total_size. This function must return a grade_s struct which contains the number of occurrences of each grade letter in the list totals. The function must use char get_letter_grade(double *grade) to get the letter grade and the function must use a switch statement. For example, if totals are {70, 80, 74, 83, 100, 95, 94, 92, 99, 50, 60}, the return value must be a struct containing: a = 5, b = 2, c = 2, d = 1, f = 1. should use the following structure: typedef struct grade_distribution_s { int f, d, c, b, a; } grades_s;
I need help In C
This program calculates and prints the total grades for students out of 100; then, it prints the number of students who got A’s, B’s, C’s, D’s, and F’s.
This program must implement the following functions:
double get_total(double grades[], int size);
This function receives a list of grades in the grades[] array and its size in the variable size. This function must calculate the total of the grades in grades[]. However, the function must drop the lowest quiz grade before calculating the total. Each grades[] list has the following elements order: {[Lab Exam/20] [Quiz 1/5] [Quiz 2/5] [Quiz 3/5] [Mid Exam/20] [Final Exam/50]}. The function will remove the lowest Quiz grade, and then it will precede to sum the rest of the grade. The total must not exceed 100. For example, if you pass 15, 5, 5, 3, 19, 46 to the function, the function will remove the three, and you will get 90. Another example, 17, 3, 1, 2, 17, 45, the function will return 84.
char get_letter_grade(double *grade);
This function receives a pointer to a grade and returns A if the grade is greater or equal to 90. B if the grade is less than 90 and greater than or equal to 80. C if the grade is less than 80 and greater or equal to 70. D if the grade is less than 70 and greater than or equal to 60. Finally, F if the grade is less than 60. grades_s get_distribution(double totals[], int totals_size); The function accepts a list of total grades totals[] for a number of students total_size. This function must return a grade_s struct which contains the number of occurrences of each grade letter in the list totals. The function must use char get_letter_grade(double *grade) to get the letter grade and the function must use a switch statement. For example, if totals are {70, 80, 74, 83, 100, 95, 94, 92, 99, 50, 60}, the return value must be a struct containing: a = 5, b = 2, c = 2, d = 1, f = 1.
should use the following structure:
typedef struct grade_distribution_s {
int f, d, c, b, a;
} grades_s;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

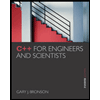
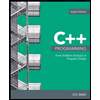
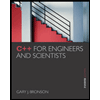
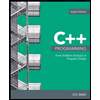