I need to do an email generator on python. once again im asking for assistance. I've attatched my code/what i've done so far. The assignment asks me to do the following: You will write a function email_generator() that creates email IDs for new members joining Cal Poly. This function must accept the member’s name as its only argument. An email ID for a member should contain five letters that are randomly picked from the member’s name, followed by the string “@calpoly.edu”. For generating random numbers, include the statement “import random” in your program. Once you do this, you can use the random.randint(0,N) statement to generate a random number between 0 and N (you will have to replace N with an appropriate integer value). Note that email IDs do not contain whitespaces. To ensure that the email IDs created for the new members do not contain whitespaces, you will need to remove whitespaces from the names. This can be done using the in-built replace(" ", "") function. For e.g., the replace function can be invoked on a string name as name.replace(" ", ""), and it will return a new string whose elements are the same as the original string name, but with all whitespaces removed from it. Similarly, since the member names can contain upper case letters (upper case letters are not typically used in email IDs), you will have to convert the input to all lower case letters (hint: you can use name.lower() function to convert all letters in a string name to lower cases. Note that this function returns a new string). Call the email_generator() function for three new members to Cal Poly: “Ada Lovelace” “Grace Hopper” “Barbara Liskov” Your program must display the three email IDs created for the three new members.
I need to do an email generator on python. once again im asking for assistance. I've attatched my code/what i've done so far.
The assignment asks me to do the following:
You will write a function email_generator() that creates email IDs for new members joining Cal Poly. This function must accept the member’s name as its only argument.
An email ID for a member should contain five letters that are randomly picked from the member’s name, followed by the string “@calpoly.edu”. For generating random numbers, include the statement “import random” in your program. Once you do this, you can use the random.randint(0,N) statement to generate a random number between 0 and N (you will have to replace N with an appropriate integer value).
Note that email IDs do not contain whitespaces. To ensure that the email IDs created for the new members do not contain whitespaces, you will need to remove whitespaces from the names. This can be done using the in-built replace(" ", "") function. For e.g., the replace function can be invoked on a string name as name.replace(" ", ""), and it will return a new string whose elements are the same as the original string name, but with all whitespaces removed from it. Similarly, since the member names can contain upper case letters (upper case letters are not typically used in email IDs), you will have to convert the input to all
lower case letters (hint: you can use name.lower() function to convert all letters in a string name to lower cases. Note that this function returns a new string).
Call the email_generator() function for three new members to Cal Poly:
“Ada Lovelace”
“Grace Hopper”
“Barbara Liskov”
Your program must display the three email IDs created for the three new members.
![PC File Edit View Navigate Code Refactor Run Iools VCS Window Help
conditonalmidterm_funcs.py - email genorator.py
CPE101Labs Lab 6
fo email genorator.py
email genorator
Week 7 Notes.py
Calculator.py
fe palindrome.py
e email genorator.py
CPE101Labs C:\Users\thepe
import random
A1 A 13 A v
lab2
vlab3
letters = ["a", "b","c", "d","e", "f","g", "h","i", "j","k", "l","m", "n",
"0", "p","q", "r","s", "t","u", "v","w", "x","y", "z",]
lab3
v conditional
fo conditional.py
6.
random.randint(0, 5)
e conditional_tes
logic.py
> logic
8
random1 = random.choice(letters)
> lab4
print(random1 + '@calpoly.edu')
9
> Lab5
10
v Lab 6
11
Calculator.py
12
o email genorator.py
e fuckaroundandfindou
fe palindrome.py
> Moonlander
13
def get_domain(domain):
14
return domain[random.randint(0, len(domain) - 1)]
15
16
v Practice for Midterm
def generate_random_emails (letters):
17
e conditionmidtermtest
, FORCANVASMidterm 18
stri = name.lower("Ada Lovelace")
email_name = str1
fo funcs2.py
v venv library root
19
20
for i in range(5):
> Lib
email_name = email_name + letters[random.randint(0, 5) + '@calpoly.edu']
21
> Scripts
22
return email_name
v share
23
> doc
24
>Iman
25
def main():
o gitignore
A LICENSE
1 pyvenv.cfg
print(generate_random_emails (11, 5))|
26
27
28
funcs.py
o funcs_tests.py
| lab2.zip
l lab3.zip
Il Lab5.zip
E LinkToCalcudokuProblen
o main.py
a Proj3How2Start copy(1).ji
2 Project1F2021a.pdf
I Project3(1).zip
1 Project3Hints(1).rtf
o testing_proj_1.py
> ulı External Libraries
29 >
if -_name_
== "_main_":
30
main()
31
> O Scratches and Consoles
main()
> Run
E TODO
O Problems
2 Terminal
E Python Packages
2 Python Console
1 Event Log
O Packages installed successfully: Installed packages: 'replace' (11 minutes ago)
26:41 CRLF UTF-8 4 spaces Python 3.8 (CPE101Labs) 1
6:52 PM
M
11/3/2021
H. Structure](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5cbd8d8a-fbca-4571-b1f6-9ae14d58fa08%2Fade20302-4b66-47e8-a046-1e5b2f903e0e%2Frexw5w_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

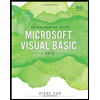
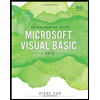