I'm developing a card game that requires one deck of 52 cards using Java. The 52 card has 4 suits from top to bottom: diamonds (d), clubs (c), hearts (h), and spades (s). Each card has a point. Based on the code below, it outputs the total point chosen from the sorted suit out of the 5 cards that gives the highest point. I used hashmaps to program this code. Instead, I want the program to perform the same as before but using comparable/comparator instead of hashmaps. The rest of the instructions are in the images.
I'm developing a card game that requires one deck of 52 cards using Java. The 52 card has 4 suits from top to bottom: diamonds (d), clubs (c), hearts (h), and spades (s). Each card has a point. Based on the code below, it outputs the total point chosen from the sorted suit out of the 5 cards that gives the highest point. I used hashmaps to program this code. Instead, I want the program to perform the same as before but using comparable/comparator instead of hashmaps. The rest of the instructions are in the images.
Chapter3: File And Folder Management
Section: Chapter Questions
Problem P2EYK
Related questions
Question
I'm developing a card game that requires one deck of 52 cards using Java. The 52 card has 4 suits from top to bottom: diamonds (d), clubs (c), hearts (h), and spades (s). Each card has a point.
Based on the code below, it outputs the total point chosen from the sorted suit out of the 5 cards that gives the highest point. I used hashmaps to program this code. Instead, I want the program to perform the same as before but using comparable/comparator instead of hashmaps.
The rest of the instructions are in the images.
import java.util.HashMap;
import java.io.*;
public class CardPointsList {
static HashMap<String,Integer> Code = new HashMap<>();
static HashMap<Character,Integer> Order = new HashMap<>();
static String[][] hand= {{ "c5","s6","sK","dK","dA"},{"s7","s4","dJ","sA","h5"},{"sQ","d3","c9","hK","d5"}};
static int[] scores={0,0,0};
public static void sortHand()
{ String temp;
for (int h=0;h<3;h++)
{for (int i=0;i<5;i++)
{ for (int j=i+1;j<5;j++)
{ if (hand[h][j].charAt(0) < hand[h][i].charAt(0))
{temp = hand[h][j];
hand[h][j] = hand[h][i];
hand[h][i] = temp;
}
else
if (hand[h][j].charAt(0) == hand[h][i].charAt(0))
{ if (Order.get(hand[h][j].charAt(1)) < Order.get(hand[h][i].charAt(1)))
{temp = hand[h][j];
hand[h][j] = hand[h][i];
hand[h][i] = temp;
}
}
} //for j
} // for i
}// for h
}
public static void printHand()
{ for (int h=0;h<3;h++) {
for (int i=0;i<5;i++)
{ System.out.println(hand[h][i]);}
System.out.println();
}
}
public static void getPoints( )
{ int[] suitScores = {0,0,0,0}; // holds the points for c,d,h,s suits, respectively.
for (int h=0;h<3;h++)
{
// calculate the point for each suit
for (int i=0;i<5;i++)
{ String myStr = Character.toString(hand[h][i].charAt(1));
String mySuit = Character.toString(hand[h][i].charAt(0));
if (mySuit.equals("c"))
suitScores[0] += Code.get(myStr);
if (mySuit.equals("d"))
suitScores[1]+= Code.get(myStr);
if (mySuit.equals("h"))
suitScores[2]+= Code.get(myStr);
if (mySuit.equals("s"))
suitScores[3]+= Code.get(myStr);
}
int max=0;
//find the max point which is the point for the hand
for (int j=0;j<4;j++)
{ if (suitScores[j]>max)
{ max=suitScores[j];}
suitScores[j]=0;
}
scores[h]=max;
System.out.println(scores[h]);
}
}
public static int getmax()
{ int max=0;
int handNum=0;
for (int h=0;h<3;h++)
{ if (scores[h]>max)
{max=scores[h];
handNum=h;
}
}
System.out.println("Maximum points :" + max);
return handNum+1;
}
public static void main(String[] args) throws IOException {
Code.put("A",1);
Code.put("2",2);
Code.put("3",3);
Code.put("4",4);
Code.put("5",5);
Code.put("6",6);
Code.put("7",7);
Code.put("8",8);
Code.put("9",9);
Code.put("X",10);
Code.put("J",10);
Code.put("K",10);
Code.put("Q",10);
Order.put('A',1);
Order.put('2',2);
Order.put('3',3);
Order.put('4',4);
Order.put('5',5);
Order.put('6',6);
Order.put('7',7);
Order.put('8',8);
Order.put('9',9);
Order.put('X',10);
Order.put('K',11);
Order.put('Q',12);
Order.put('J',13);
sortHand();
printHand();
getPoints();
int maxh=getmax();
System.out.println("Maximum points are for hand " + maxh);
}
}
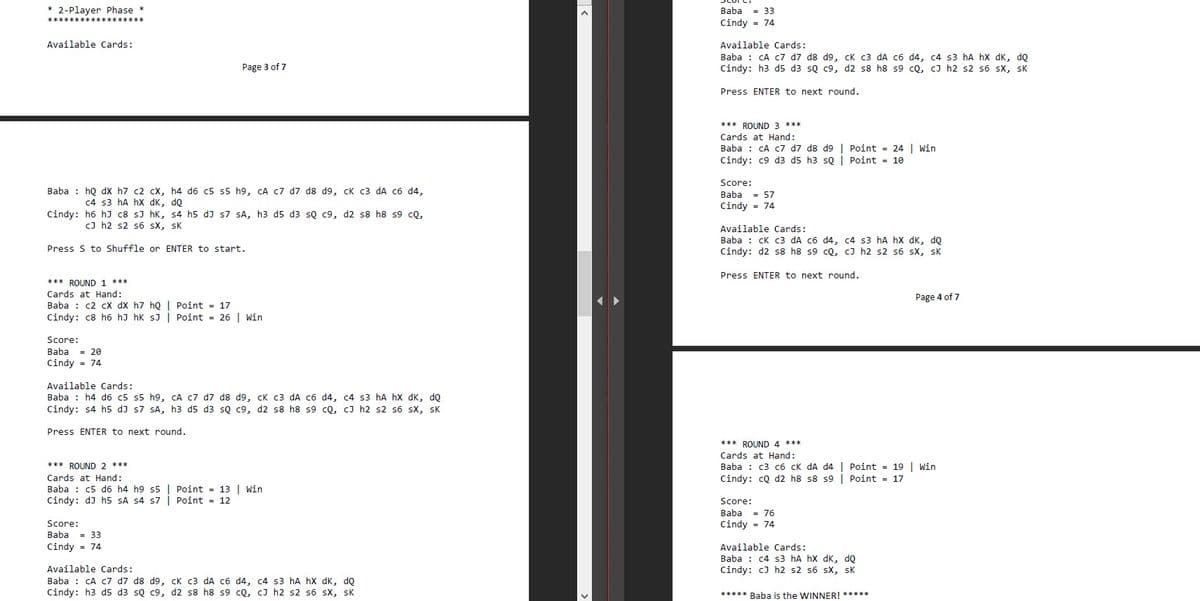
Transcribed Image Text:* 2-Player Phase *
Baba
= 33
***********
Cindy = 74
Available Cards:
Available Cards:
Baba : CA c7 d7 d8 d9, cK c3 dA c6 d4, c4 s3 hA hX dK, dQ
Cindy: h3 d5 d3 so c9, d2 s8 h8 s9 cọ, cJ h2 s2 s6 sX, sK
Page 3 of 7
Press ENTER to next round.
*** ROUND 3 ***
Cards at Hand:
Baba : CA c7 d7 d8 d9 | Point = 24 | Win
Cindy: c9 d3 d5 h3 sQ | Point = 10
Score:
Baba : họ dx h7 c2 cX, h4 d6 c5 s5 h9, CA c7 d7 d8 d9, cK c3 dA c6 d4,
= 57
Cindy = 74
Baba
c4 s3 hA hx dK, dQ
Cindy: h6 hJ c8 sJ hk, s4 h5 dJ s7 sSA, h3 d5 d3 sQ c9, d2 s8 h8 s9 cQ,
c) h2 s2 s6 sx, sK
Available Cards:
Baba : cK c3 dA có d4, c4 s3 hA hx dK, do
Cindy: d2 s8 h8 s9 cQ, cJ h2 s2 s6 sX, sK
Press S to Shuffle or ENTER to start.
Press ENTER to next round.
*** ROUND 1 ***
Cards at Hand:
Page 4 of 7
Baba : c2 cX dX h7 hQ | Point = 17
Cindy: c8 h6 hJ hk sJ
Point = 26 | win
Score:
Baba
= 20
Cindy = 74
Available Cards:
Baba : h4 d6 c5 s5 h9, CA c7 d7 d8 d9, ck c3 dA C6 d4, c4 s3 hA hX dK, dQ
Cindy: s4 h5 dJ s7 sA, h3 d5 d3 sQ c9, d2 s8 h8 s9 cQ, c) h2 s2 s6 sx, sK
Press ENTER to next round.
*** ROUND 4 ***
Cards at Hand:
Baba : c3 c6 ck dA d4 | Point = 19 | Win
Cindy: cQ d2 h8 s8 s9 | Point = 17
*** ROUND 2 ***
Cards at Hand:
Baba : c5 d6 h4 h9 s5 | Point = 13 | Win
Cindy: dJ h5 SA s4 s7 | Point = 12
Score:
Baba
= 76
Score:
Cindy - 74
Baba
= 33
Cindy - 74
Available Cards:
Baba : c4 s3 hA hx dK, dọ
Cindy: cJ h2 s2 s6 sX, sk
Available Cards:
Baba : CA c7 d7 d8 d9, cK
Cindy: h3 d5 d3 sQ c9, d2 s8 h8 s9 cQ, cJ h2 s2 s6 sX, sK
c3
dA c6 d4, c4 s3 hA hX dK, dQ
***** Baba is the WINNER! *****
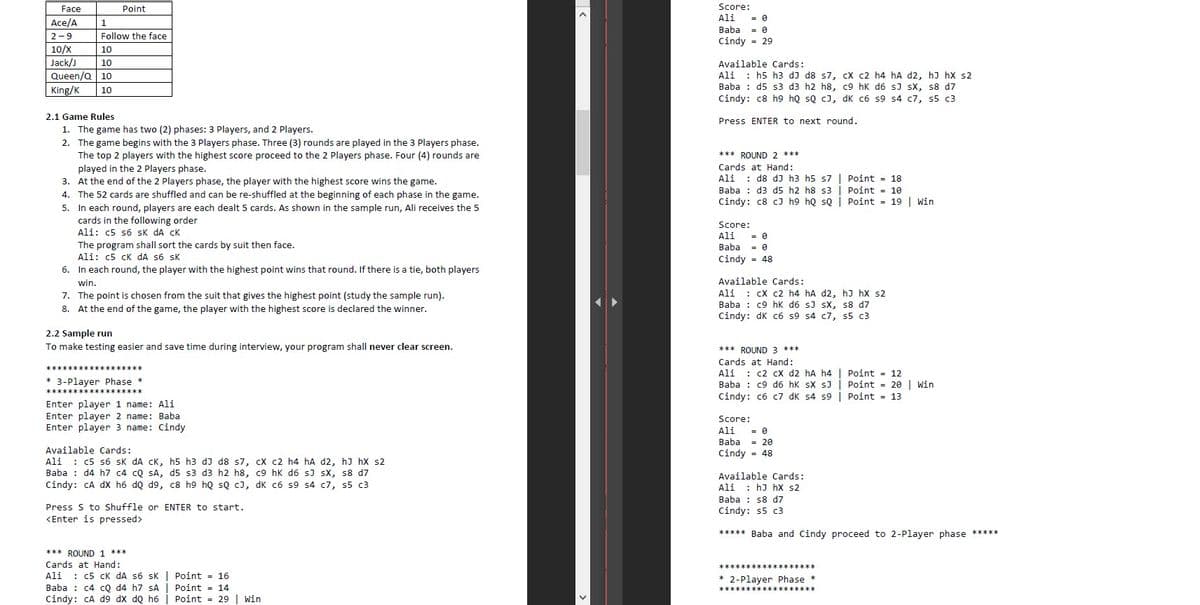
Transcribed Image Text:Face
Point
Score:
Ali
= 0
= 0
Ace/A
1
Baba
2-9
Follow the face
Cindy = 29
10/X
10
Jack/J
Queen/Q 10
King/K
10
Available Cards:
: h5 h3 dJ d8 s7, cX c2 h4 hA d2, hJ hX s2
Ali
Baba : d5 s3 d3 h2 h8, c9 hk d6 s) sX, s8 d7
Cindy: c8 h9 hQ sQ cJ, dK c6 s9 s4 c7, s5 c3
10
2.1 Game Rules
Press ENTER to next round.
1. The game has two (2) phases: 3 Players, and 2 Players.
2. The game begins with the 3 Players phase. Three (3) rounds are played in the 3 Players phase.
The top 2 players with the highest score proceed to the 2 Players phase. Four (4) rounds are
played in the 2 Players phase.
3. At the end of the 2 Players phase, the player with the highest score wins the game.
*** ROUND 2 ***
Cards at Hand:
Ali : d8 dJ h3 h5 s7| Point = 18
Baba : d3 d5 h2 h8 s3 | Point = 10
Cindy: c8 cJ h9 hQ sQ | Point = 19 | win
4. The 52 cards are shuffled and can be re-shuffled at the beginning of each phase in the game.
5. In each round, players are each dealt 5 cards. As shown in the sample run, Ali receives the 5
cards in the following order
Ali: c5 s6 sk dA CK
Score:
Ali
Baba
The program shall sort the cards by suit then face.
Ali: c5 cK dA s6 sK
6. In each round, the player with the highest point wins that round. If there is a tie, both players
Cindy = 48
win.
Available Cards:
: CX c2 h4 hA d2, hJ hx s2
Baba : c9 hK d6 sJ sX, s8 d7
Cindy: dK c6 s9 s4 c7, s5 c3
Ali
7. The point is chosen from the suit that gives the highest point (study the sample run).
8. At the end of the game, the player with the highest score is declared the winner.
2.2 Sample run
To make testing easier and save time during interview, your program shall never clear screen.
*** ROUND 3 ***
Cards at Hand:
: c2 cX d2 hA h4 | Point = 12
Ali
Baba : c9 d6 hk sX sJ | Point = 20 | win
Cindy: c6 c7 dK s4 s9 | Point = 13
* 3-Player Phase *
****
Enter player 1 name: Ali
Enter player 2 name: Baba
Enter player 3 name: Cindy
Score:
Ali
Baba
= 20
Available Cards:
Cindy = 48
: c5 s6 sk dA CK, h5 h3 dJ d8 s7, cXx c2 h4 hA d2, hJ hx s2
Ali
Baba : d4 h7 c4 cQ SA, d5 s3 d3 h2 h8, c9 hk d6 sJ sx, s8 d7
Cindy: CA dx h6 dQ d9, c8 h9 hQ sQ cJ, dK c6 s9 s4 c7, s5 c3
Available Cards:
Ali
: hJ hX s2
Baba : s8 d7
Cindy: s5 c3
Press S to Shuffle or ENTER to start.
<Enter is pressed>
***** Baba and Cindy proceed to 2-Player phase *****
*** ROUND 1 ***
Cards at Hand:
Ali : c5 ck dA s6 sK | Point = 16
Baba : c4 cQ d4 h7 SA| Point = 14
Cindy: CA d9 dX dQ h6
*****
* 2-Player Phase *
******************
Point = 29 | win
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:
9780357392607
Author:
FREUND
Publisher:
Cengage
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:
9780357392607
Author:
FREUND
Publisher:
Cengage