java In this assignment you will swap a position in an array list with another. swap() gets 3 arguments, an Arraylist, a position, and another position to swap with. Example swap(["one","two","three"],0,2) returns:["three","two","one"] public static ArrayList swap(ArrayList list,int pos1,int pos2) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int pos1 = in.nextInt(); int pos2 = in.nextInt(); ArrayList list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.next()); } System.out.println(swap(list, pos1, pos2)); } }
java In this assignment you will swap a position in an array list with another. swap() gets 3 arguments, an Arraylist, a position, and another position to swap with. Example swap(["one","two","three"],0,2) returns:["three","two","one"] public static ArrayList swap(ArrayList list,int pos1,int pos2) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int pos1 = in.nextInt(); int pos2 = in.nextInt(); ArrayList list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.next()); } System.out.println(swap(list, pos1, pos2)); } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
java
In this assignment you will swap a position in an array list with another.
swap() gets 3 arguments, an Arraylist, a position, and another position to swap with.
Example
swap(["one","two","three"],0,2)
returns:["three","two","one"]
public static ArrayList<String> swap(ArrayList<String> list,int pos1,int pos2)
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int size = in.nextInt();
int pos1 = in.nextInt();
int pos2 = in.nextInt();
ArrayList<String> list = new ArrayList<>();
for(int i=0; i < size; i++) {
list.add(in.next());
}
System.out.println(swap(list, pos1, pos2));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
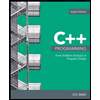
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
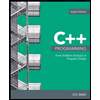
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning