have to create a program file using python. I have created another program that would correlate with this code. I don't where to start. I will attach my program and the instructions. Create a file that has the data for at least five points. You can use notepad to make your file. Each line in the file should have each point’s lat and lon coordinates and a description. It could look like this: 100.200, 123.456, Main Campus 120.33, 142.345, Montoya 153.23, 322.345, Rio Rancho 133.23, 143.345, STEMULUS Center 153.42, 122.345, ATC (Note: The “Points.txt” file attached to the assignment can be used instead of creating your own file.) In the main part of your program do the following: Create a list that you will use to collect the points. Something like pointsList = []. Read points in from a file. As you read lat lon and description from the file, use those values to create a point and add that point to a List. Something like: newPoint = GeoPoint(lat, lon, description) pointsList.append(newPoint) Inside the “Do another (y/n)?” loop do the following: Ask the user for their location. Create a point to represent the user’s location. Iterate through the point list and find the closest point. Tell the user which point they are closest to in this format: You are closest to which is located at Ask “Do another (y/n)?” and loop if they respond with ‘y’
have to create a program file using python. I have created another program that would correlate with this code. I don't where to start. I will attach my program and the instructions. Create a file that has the data for at least five points. You can use notepad to make your file. Each line in the file should have each point’s lat and lon coordinates and a description. It could look like this: 100.200, 123.456, Main Campus 120.33, 142.345, Montoya 153.23, 322.345, Rio Rancho 133.23, 143.345, STEMULUS Center 153.42, 122.345, ATC (Note: The “Points.txt” file attached to the assignment can be used instead of creating your own file.) In the main part of your program do the following: Create a list that you will use to collect the points. Something like pointsList = []. Read points in from a file. As you read lat lon and description from the file, use those values to create a point and add that point to a List. Something like: newPoint = GeoPoint(lat, lon, description) pointsList.append(newPoint) Inside the “Do another (y/n)?” loop do the following: Ask the user for their location. Create a point to represent the user’s location. Iterate through the point list and find the closest point. Tell the user which point they are closest to in this format: You are closest to which is located at Ask “Do another (y/n)?” and loop if they respond with ‘y’
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter9: Sequential Access Files And Menus
Section: Chapter Questions
Problem 11E
Related questions
Question
100%
I have to create a program file using python. I have created another program that would correlate with this code. I don't where to start. I will attach my program and the instructions.
- Create a file that has the data for at least five points. You can use notepad to make your file.
- Each line in the file should have each point’s lat and lon coordinates and a description. It could look like this:
100.200, 123.456, Main Campus
120.33, 142.345, Montoya
153.23, 322.345, Rio Rancho
133.23, 143.345, STEMULUS Center
153.42, 122.345, ATC
(Note: The “Points.txt” file attached to the assignment can be used instead of creating your own file.)
- In the main part of your program do the following:
- Create a list that you will use to collect the points. Something like pointsList = [].
- Read points in from a file.
- As you read lat lon and description from the file, use those values to create a point and add that point to a List. Something like:
newPoint = GeoPoint(lat, lon, description)
pointsList.append(newPoint)
- Inside the “Do another (y/n)?” loop do the following:
- Ask the user for their location.
- Create a point to represent the user’s location.
- Iterate through the point list and find the closest point.
- Tell the user which point they are closest to in this format:
You are closest to <description> which is located at <point’s lat, lon coordinates>
- Ask “Do another (y/n)?” and loop if they respond with ‘y’

Transcribed Image Text:6
7
8
9
10
11
12
IDLE File Edit Shell Debug Options Window
View
125% ✓
Zoom
+
Add Page
Help
v. Finally ask the user if they want to do another.
4. When done display a good bye message outside the loop.
P4-ProgramWithFunctions 2
Insert Table Chart Text Shape Media Comment
Testing
Test your program by finding the Lat and Long for Albuquerque and the Lat and Long for Santa Fe using google maps and
calculate the distance between the two. The answer should be about 93.07458 km.
A
Hints:
Latitude and longitude can be expressed in Degrees, minutes and seconds or decimal degrees. It is easier to tell the user to
input the Latitude and Longitude as decimal degrees, it will be much easier to do this math so you don't have to convert from
hours minutes and seconds to decimal and then covert back to radians. Instead you can just convert the entered decimal
degrees coordinates to radians. (math.radians)
BOO
ddddddd
The function that gets the point coordinates from the user will be called twice. Once for each point. Your code will look
something like:
point1 = get_location()
point2 = get_location()
get_location () will return a tuple or list. Something like (lat, long) depending on what variable names you use in your function.
If you use "input" to get the coordinates don't forget to convert the string into a number (something like float(x)).
The function that returns distance will be called this way:
distance = calcDistance (point1, point2)
point1 and point2 will be the geo point location entered from the get_location() function.
ост 1
19
.
●
tv
+
Collaborate
List P
Font
Cali
B
Text
Sp
Line
Python 3.7.9 Shell
print (f"The distance betwwen {loc1} and {loc2} is {distance (loc1, loc2):.2f}
meters.")
File "/Users/tishenayazzie/Documents/Yazzie TP4.py", line 44, in distance
lat2 locations2. latitude * math.pi / 180
NameError: name 'locations2' is not defined
========= RESTART: /Users/tishenayazzie/Documents/Yazzie TP4.py
>>>
Reg meters.")
Welcome!
This program lets you find the distance between to geographical points
Enter Latitude (degree): 35
Enter Longitude (degree): -106
Enter Latitude (degree): 0
Enter Longitude (degree): 0
File "/Users/tishenayazzie/Documents/Yazzie TP4.py", line 44, in distance
lat2 = locations2. latitude * math.pi / 180
NameError: name 'locations2' is not defined
Chara ============= RESTART: /Users/tishenayazzie/Documents/YazzieTP4.py
2
Traceback (most recent call last):
File "/Users/tishenayazzie/Documents/Yazzie TP4.py", line 60, in <module>
print (f"The distance betwwen {loc1} and {loc2} is {distance(loc1, loc2):.2f}
>>>
Welcome!
This program lets you find the distance between to geographical points
Enter Latitude (degree): 35
Enter Longitude (degree): 106
Enter Latitude (degree): 35
Enter Longitude (degree): 105
Traceback (most recent call last):
File "/Users/tishenayazzie/Documents/YazzieTP4.py", line 60, in <module>
print (f"The distance betwwen {loc1} and {loc2} is {distance(loc1, loc2):.2f}
meters.")
Before
File "/Users/tishenayazzie/Documents/Yazzie TP4.py", line 44, in distance
lat2 = locations2. latitude * math.pi / 180
NameError: name 'locations2' is not defined
>>>
After Paragraph
Wed Oct 19 4:46 PM
> Bullets & Lists Imported Style 2
Drop Cap
A
8 pt
All
Ln: 10
DOWNLOAD
Col: 32
Ln: 55
Col: 0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I'm getting an error 'GeoPoint' not defined when I run the code.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
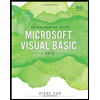
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
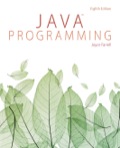
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
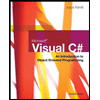
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
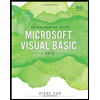
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
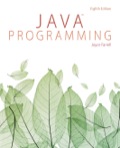
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
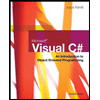
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage