Im trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } } Object lastValue = aStack.pop(); if (!aStack.isEmpty()) { System.out.println("Stack has more operands than operators"); return Double.NEGATIVE_INFINITY; } else return (double) lastValue; //return -1; }
Im trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } } Object lastValue = aStack.pop(); if (!aStack.isEmpty()) { System.out.println("Stack has more operands than operators"); return Double.NEGATIVE_INFINITY; } else return (double) lastValue; //return -1; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 2SA
Related questions
Question
Im trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error.
this is the code I have so far:
static double evaluatePostfixExpression(String expression){
// Write your code
Stack aStack = new Stack();
double value1, value2;
String token;
StringTokenizer tokenizer = new StringTokenizer(expression);
while (tokenizer.hasMoreTokens()) {
token = tokenizer.nextToken();
if(isNumber(expression)) {
value1 = (double)aStack.pop();
value2 = (double)aStack.pop();
if (token.equals("+")) {
aStack.push(value2+value1);
} else if (token.equals("-")) {
aStack.push(value2-value1);
} else if (token.equals("*")) {
aStack.push(value2*value1);
} else if (token.equals("/")) {
aStack.push(value2/value1);
}
}
}
Object lastValue = aStack.pop();
if (!aStack.isEmpty()) {
System.out.println("Stack has more operands than operators");
return Double.NEGATIVE_INFINITY;
}
else
return (double) lastValue;
//return -1;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
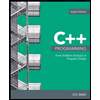
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
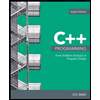
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning