Modify the Java class for the abstract stack type shown below to use a linked list representation and test it with the same code that appears in this chapter. class StackClass { private int [] stackRef; private int maxLen, topIndex; public StackClass() { // A constructor stackRef = new int [100]; maxLen = 99; topIndex = -1; } public void push(int number) { if (topIndex == maxLen) System.out.println("Error in push–stack is full"); else stackRef[++topIndex] = number; } public void pop() { if (empty()) System.out.println("Error in pop–stack is empty"); else --topIndex; } public int top() { if (empty()) { System.out.println("Error in top–stack is empty"); return 9999; } else return (stackRef[topIndex]); } public boolean empty() {return (topIndex == -1);} } An example class that uses StackClass follows: public class TstStack { public static void main(String[] args) { StackClass myStack = new StackClass(); myStack.push(42); myStack.push(29); System.out.println("29 is: " + myStack.top()); myStack.pop(); System.out.println("42 is: " + myStack.top()); myStack.pop(); myStack.pop(); // Produces an error message } }
Modify the Java class for the abstract stack type shown below
to use a linked list representation and test it with the same code that
appears in this chapter.
class StackClass {
private int [] stackRef;
private int maxLen,
topIndex;
public StackClass() { // A constructor
stackRef = new int [100];
maxLen = 99;
topIndex = -1;
}
public void push(int number) {
if (topIndex == maxLen)
System.out.println("Error in push–stack is full");
else stackRef[++topIndex] = number;
}
public void pop() {
if (empty())
System.out.println("Error in pop–stack is empty");
else --topIndex;
}
public int top() {
if (empty()) {
System.out.println("Error in top–stack is empty");
return 9999;
}
else
return (stackRef[topIndex]);
}
public boolean empty() {return (topIndex == -1);}
}
An example class that uses StackClass follows:
public class TstStack {
public static void main(String[] args) {
StackClass myStack = new StackClass();
myStack.push(42);
myStack.push(29);
System.out.println("29 is: " + myStack.top());
myStack.pop();
System.out.println("42 is: " + myStack.top());
myStack.pop();
myStack.pop(); // Produces an error message
}
}

Step by step
Solved in 2 steps with 1 images

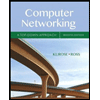
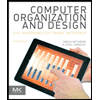
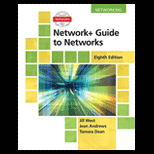
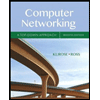
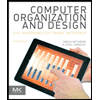
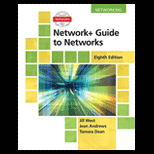
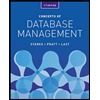
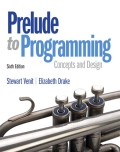
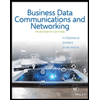