Implement a C++ program that prompts the user to enter two positive numbers as formatted below Enter num1: Enter num2: Input validation MUST be completed input must be all digits input must be a positive number, Integer Overflow for the individual inputs and the sum of the inputs is now VALID and needs to be handled as described below with the ADDITION ALGORITHM. If NOT valid (non-digits or negative) then re-prompt as formatted below INVALID RE-Enter num 3 incorrect inputs in a row and stop program. This is the 3 strikes and you're out rule. PROGRAM ABORT ADDITION ALGORITH The input CANNOT be converted to a true int, unsigned int, long, unsigned long or long long data type The solution is to use an array to store each individual digit of the number For example, the inputted number: "1234" could be stored in the array nums backwards, so the ones position is at index 0 of the array. 4 = 10^0 (ones position) so that nums[0] = 4 3 = 10^1 (tens position) so that nums[1] = 3 2 = 10^2 (hundreds position) so that nums[2] = 2 1 = 10^3 (thousands position) so that nums[3] = 1 The program will perform the addition operation by implementing the usual paper-and-pencil addition algorithm (adding the ones digits and if greater than 10, then carry the 1 to the tens position, etc) Add starting with ones digits and carry the 1 if 10 or over. For the below example the following steps are completed sum[0] = 5+6+0(no carry over) =11 store the 1 and carry the 1 to the next index. sum[1] = 4+7+1(carry over)=12 store the 2 and carry the 1 to the next index. sum[2] = 3+8+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[3] = 2+9+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[4] = 1+0+1(carry over) = 2 store the 2 Reverse the sum array to get the answer. The ones place at index 0, should be written as the right most value. Answer: 22221 Outputs the sum of the two positive integers when input is valid as formatted below in the example test runs. Make sure to follow the Grading Rubric and Academic Integrity guidelines outlined in the syllabus. Expected Program Output: Test Run #1 Enter num1: 12345 Enter num2: 9876 12345 + 9876 ---------- 22221 Test Run #2 Enter num1: 378 Enter num2: 16429 378 + 16429 ----------- 16807 Test Run #3 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551616 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: 18446744073709551616 18446744073709551616 + 18446744073709551616 ----------------------------------- 36893488147419103232 Test Run #4 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551614 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: -2 PROGRAM ABORT Test Run #5 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551619 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: 1 18446744073709551619 + 1 ------------------------------------ 18446744073709551620 Test Run #6 Enter num1: 111213141516171819 Enter num2: 2132435465768798 111213141516171819 + 2132435465768798 --------------------------------- 113345576981940617
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
-
Implement a C++ program that prompts the user to enter two positive numbers as formatted below
Enter num1:Enter num2:- Input validation MUST be completed
- input must be all digits
- input must be a positive number,
- Integer Overflow for the individual inputs and the sum of the inputs is now VALID and needs to be handled as described below with the ADDITION
ALGORITHM . - If NOT valid (non-digits or negative) then re-prompt as formatted below
INVALID RE-Enter num
- 3 incorrect inputs in a row and stop program. This is the 3 strikes and you're out rule.
PROGRAM ABORT-
-
ADDITION ALGORITH
-
The input CANNOT be converted to a true int, unsigned int, long, unsigned long or long long data type
-
The solution is to use an array to store each individual digit of the number
-
For example, the inputted number: "1234" could be stored in the array nums backwards, so the ones position is at index 0 of the array.
4 = 10^0 (ones position) so that nums[0] = 43 = 10^1 (tens position) so that nums[1] = 32 = 10^2 (hundreds position) so that nums[2] = 21 = 10^3 (thousands position) so that nums[3] = 1 -
The program will perform the addition operation by implementing the usual paper-and-pencil addition algorithm (adding the ones digits and if greater than 10, then carry the 1 to the tens position, etc)
- Add starting with ones digits and carry the 1 if 10 or over. For the below example the following steps are completed
- sum[0] = 5+6+0(no carry over) =11 store the 1 and carry the 1 to the next index.
- sum[1] = 4+7+1(carry over)=12 store the 2 and carry the 1 to the next index.
- sum[2] = 3+8+1(carry over) = 12 store the 2 and carry the 1 to the next index.
- sum[3] = 2+9+1(carry over) = 12 store the 2 and carry the 1 to the next index.
- sum[4] = 1+0+1(carry over) = 2 store the 2
- Reverse the sum array to get the answer. The ones place at index 0, should be written as the right most value.
- Answer: 22221
- Add starting with ones digits and carry the 1 if 10 or over. For the below example the following steps are completed
-
- Outputs the sum of the two positive integers when input is valid as formatted below in the example test runs.
- Make sure to follow the Grading Rubric and Academic Integrity guidelines outlined in the syllabus.
Expected Program Output:
Test Run #1
Enter num1: 12345Enter num2: 987612345
+ 9876
----------
22221Test Run #2
Enter num1: 378Enter num2: 16429378+ 16429-----------16807Test Run #3
Enter num1: -378INVALID RE-Enter num1: abcINVALID RE-Enter num1: 18446744073709551616Enter num2: 16abcINVALID RE-Enter num2: -16INVALID RE-Enter num2: 1844674407370955161618446744073709551616+ 18446744073709551616-----------------------------------36893488147419103232Test Run #4
Enter num1: -378INVALID RE-Enter num1: abcINVALID RE-Enter num1: 18446744073709551614Enter num2: 16abcINVALID RE-Enter num2: -16INVALID RE-Enter num2: -2PROGRAM ABORTTest Run #5
Enter num1: -378INVALID RE-Enter num1: abcINVALID RE-Enter num1: 18446744073709551619Enter num2: 16abcINVALID RE-Enter num2: -16INVALID RE-Enter num2: 118446744073709551619+ 1------------------------------------18446744073709551620Test Run #6
Enter num1: 111213141516171819Enter num2: 2132435465768798111213141516171819+ 2132435465768798---------------------------------113345576981940617
- Input validation MUST be completed

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

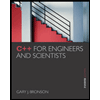
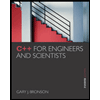