Rewrite a C++ Program with the given code and instructions: Example 5-6 implements the Number Guessing Game program. If the guessed number is not correct, the program outputs a message indicating whether the guess is low or high. Modify the program as follows: Suppose that the variables num and guess are as declared in Example 5-6 and diff is an int variable. Let diff = the absolute value of (num - guess). If diff is 0, then guess is correct and the program outputs a message indicating that the user guessed the correct number. Suppose diff is not 0. Then the program outputs the message as follows: If diff is greater than or equal to 50, the program outputs the message indicating that the guess is very high (if guess is greater than num) or very low (if guess is less than num). If diff is greater than or equal to 30 and less than 50, the program outputs the message indicating that the guess is high (if guess is greater than num) or low (if guess is less than num). If diff is greater than or equal to 15 and less than 30, the program outputs the message indicating that the guess is moderately high (if guess is greater than num) or moderately low (if guess is less than num). If diff is greater than 0 and less than 15, the program outputs the message indicating that the guess is somewhat high (if guess is greater than num) or somewhat low (if guess is less than num). As in Programming Exercise 15, give the user no more than five tries to guess the number. (To find the absolute value of num - guess, use the expression abs(num - guess). The function abs is from the header file cstdlib.)
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Rewrite a C++ Program with the given code and instructions:
Example 5-6 implements the Number Guessing Game program. If the guessed number is not correct, the program outputs a message indicating whether the guess is low or high.
Modify the program as follows: Suppose that the variables num and guess are as declared in Example 5-6 and diff is an int variable. Let diff = the absolute value of (num - guess).
If diff is 0, then guess is correct and the program outputs a message indicating that the user guessed the correct number.
Suppose diff is not 0. Then the program outputs the message as follows:
- If diff is greater than or equal to 50, the program outputs the message indicating that the guess is very high (if guess is greater than num) or very low (if guess is less than num).
- If diff is greater than or equal to 30 and less than 50, the program outputs the message indicating that the guess is high (if guess is greater than num) or low (if guess is less than num).
- If diff is greater than or equal to 15 and less than 30, the program outputs the message indicating that the guess is moderately high (if guess is greater than num) or moderately low (if guess is less than num).
- If diff is greater than 0 and less than 15, the program outputs the message indicating that the guess is somewhat high (if guess is greater than num) or somewhat low (if guess is less than num).
As in
(To find the absolute value of num - guess, use the expression abs(num - guess). The function abs is from the header file cstdlib.)
Code:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

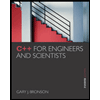
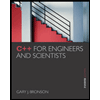