Implement all the classes using Java programming language from the given UML Class diagram. Note: This problem requires you to submit just two classes: Circle.java, Cylinder.java. Do NOT include "public static void main()" method inside all of these classes. In this exercise, a subclass called Cylinder is derived from the superclass Circle as shown in the class diagram (where an arrow pointing up from the subclass to its superclass). Study how the subclass Cylinder invokes the superclass' constructors (via super() and super(radius)) and inherits the variables and methods from the superclass Circle. You can reuse the Circle class that you have created in the previous exercise. Make sure that you keep "Circle.class" in the same directory. Method Overriding and "Super": The subclass Cylinder inherits getArea() method from its superclass Circle. Try overriding the getArea() method in the subclass Cylinder to compute the surface area (=π×radius×radius×height) of the cylinder instead of the base area. That is, if getArea() is called by a Circle instance, it returns the area. If getArea() is called by a Cylinder instance, it returns the surface area of the cylinder. If you override the getArea() in the subclass Cylinder, the getVolume() no longer works. This is because the getVolume() uses the overridden getArea() method found in the same class. (Java runtime will search the superclass only if it cannot locate the method in this class). Fix the getVolume().
Implement all the classes using Java
Note: This problem requires you to submit just two classes: Circle.java, Cylinder.java.
Do NOT include "public static void main()" method inside all of these classes.
In this exercise, a subclass called Cylinder is derived from the superclass Circle as shown in the class diagram (where an arrow pointing up from the subclass to its superclass). Study how the subclass Cylinder invokes the superclass' constructors (via super() and super(radius)) and inherits the variables and methods from the superclass Circle.
You can reuse the Circle class that you have created in the previous exercise. Make sure that you keep "Circle.class" in the same directory.
Method Overriding and "Super": The subclass Cylinder inherits getArea() method from its superclass Circle. Try overriding the getArea() method in the subclass Cylinder to compute the surface area (=π×radius×radius×height) of the cylinder instead of the base area. That is, if getArea() is called by a Circle instance, it returns the area. If getArea() is called by a Cylinder instance, it returns the surface area of the cylinder.
If you override the getArea() in the subclass Cylinder, the getVolume() no longer works. This is because the getVolume() uses the overridden getArea() method found in the same class. (Java runtime will search the superclass only if it cannot locate the method in this class). Fix the getVolume().
Hints: After overriding the getArea() in subclass Cylinder, you can choose to invoke the getArea() of the superclass Circle by calling super.getArea().
TRY: Provide a toString() method to the Cylinder class, which overrides the toString() inherited from the superclass Circle, e.g.,
Try out the toString() method in TestCylinder.
Note: @Override is known as an annotation (introduced in JDK 1.5), which asks the compiler to check whether there is such a method in the superclass to be overridden. This helps greatly if you misspell the name of the toString(). If @Override is not used and toString() is misspelled as ToString(), it will be treated as a new method in the subclass, instead of overriding the superclass. If @Override is used, the compiler will signal an error. @Override annotation is optional but certainly nice to have.
![Circle
-radius:double = 1.0
-color:String = "red"
%3D
+Circle()
+Circle(radius: double)
+Circle(radius:double,color:String)
+getRadius (): double
+setRadius (radius:double):void
+getColor():String
+setColor(color:String):void
+getArea(): double
+tostring():String.
"Circle[radius=r,color=c]"
superclass
subclass
extends
Cylinder
-height:double = 1.0
+Cylinder()
+Cylinder(radius:double)
+Cylinder(radius:double, height:double)
+Cylinder (radius:double, height:double,
color:String)
+getHeight():double
+setHeight (height:double):void
+getVolume (): double](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff3dad014-c6bb-4735-812f-053559c6a885%2F7c6cfd7e-da66-4e28-8f80-0046586e554e%2Fb28n6wg_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

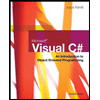
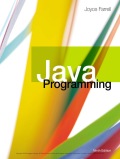
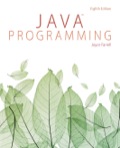
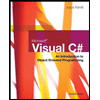
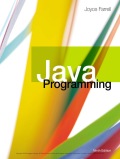
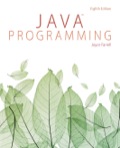