import java.util.Scanner; public class SportsBus extends Bus { private int competitorArea; private int numSecurity; private Bus bus; public SportsBus(Bus b, int competitorArea, int numSecurity) { super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry()); bus = b; this.competitorArea = competitorArea; this.numSecurity=numSecurity; } mport java.util.Scanner; public class TrainingBus extends Bus { private int teacherArea; public TrainingBus(Bus b, int teacherArea) { super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry()); this.teacherArea = teacherArea; } import java.util.Scanner; public class PartyBus extends SportsBus{ int barArea; public PartyBus(SportsBus s, int barArea) { super( s.getBus(), s.getCompetitorArea(), s.getNumSecurity()); this.barArea = barArea; } public class Bus implements Comparable { private String name; private int size; private int price; private ArrayList approvedTrips; private int level; // 1,2,3 for low,medium, high repectively; private int id; private static int nextId=0; private Ministry mny; protected String tripTypes; private int getNextId(){ return ++nextId; } public Bus(){ approvedTrips=new ArrayList(); } public Bus( String name, int size, int price, int lev, Ministry mny) { approvedTrips=new ArrayList(); this.name = name; this.size =size; this.price = price; this.level = lev; this.id = getNextId(); this.mny = mny; tripTypes = "BASICTRANSPORT"; } public int compareTo(Bus other) { return this.getName().compareTo(other.getName()); } Task: to be completed : Complete the method loadBuses(String bfile) that reads information bus information from a file (bfile contains the filename) . Each row in the file should trigger the creation of an instance of bus or an appropriate subclass, according to the data in table 1. All buses created should be added to an arraylist. LoadBuses should return the populated arraylist. please view the images aswell thanks in advance
import java.util.Scanner; public class SportsBus extends Bus { private int competitorArea; private int numSecurity; private Bus bus; public SportsBus(Bus b, int competitorArea, int numSecurity) { super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry()); bus = b; this.competitorArea = competitorArea; this.numSecurity=numSecurity; } mport java.util.Scanner; public class TrainingBus extends Bus { private int teacherArea; public TrainingBus(Bus b, int teacherArea) { super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry()); this.teacherArea = teacherArea; } import java.util.Scanner; public class PartyBus extends SportsBus{ int barArea; public PartyBus(SportsBus s, int barArea) { super( s.getBus(), s.getCompetitorArea(), s.getNumSecurity()); this.barArea = barArea; } public class Bus implements Comparable { private String name; private int size; private int price; private ArrayList approvedTrips; private int level; // 1,2,3 for low,medium, high repectively; private int id; private static int nextId=0; private Ministry mny; protected String tripTypes; private int getNextId(){ return ++nextId; } public Bus(){ approvedTrips=new ArrayList(); } public Bus( String name, int size, int price, int lev, Ministry mny) { approvedTrips=new ArrayList(); this.name = name; this.size =size; this.price = price; this.level = lev; this.id = getNextId(); this.mny = mny; tripTypes = "BASICTRANSPORT"; } public int compareTo(Bus other) { return this.getName().compareTo(other.getName()); } Task: to be completed : Complete the method loadBuses(String bfile) that reads information bus information from a file (bfile contains the filename) . Each row in the file should trigger the creation of an instance of bus or an appropriate subclass, according to the data in table 1. All buses created should be added to an arraylist. LoadBuses should return the populated arraylist. please view the images aswell thanks in advance
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 15RQ
Related questions
Question
import java.util.Scanner;
public class SportsBus extends Bus {
private int competitorArea;
private int numSecurity;
private Bus bus;
public SportsBus(Bus b, int competitorArea, int numSecurity)
{
super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry());
bus = b;
this.competitorArea = competitorArea;
this.numSecurity=numSecurity;
}
mport java.util.Scanner;
public class TrainingBus extends Bus {
private int teacherArea;
public TrainingBus(Bus b, int teacherArea)
{
super(b.getName(), b.getSize(), b.getPrice(), b.getLevel(), b.getMinistry());
this.teacherArea = teacherArea;
}
import java.util.Scanner;
public class PartyBus extends SportsBus{
int barArea;
public PartyBus(SportsBus s, int barArea)
{
super( s.getBus(), s.getCompetitorArea(), s.getNumSecurity());
this.barArea = barArea;
}
public class Bus implements Comparable<Bus> {
private String name;
private int size;
private int price;
private ArrayList<Trip> approvedTrips;
private int level; // 1,2,3 for low,medium, high repectively;
private int id;
private static int nextId=0;
private Ministry mny;
protected String tripTypes;
private int getNextId(){
return ++nextId;
}
public Bus(){
approvedTrips=new ArrayList<Trip>();
}
public Bus( String name, int size, int price, int lev, Ministry mny) {
approvedTrips=new ArrayList<Trip>();
this.name = name;
this.size =size;
this.price = price;
this.level = lev;
this.id = getNextId();
this.mny = mny;
tripTypes = "BASICTRANSPORT";
}
public int compareTo(Bus other)
{
return this.getName().compareTo(other.getName());
}
Task: to be completed : Complete the method loadBuses(String bfile) that reads information bus information from a
file (bfile contains the filename) . Each row in the file should trigger the creation of an
instance of bus or an appropriate subclass, according to the data in table 1. All buses created
should be added to an arraylist. LoadBuses should return the populated arraylist.
file (bfile contains the filename) . Each row in the file should trigger the creation of an
instance of bus or an appropriate subclass, according to the data in table 1. All buses created
should be added to an arraylist. LoadBuses should return the populated arraylist.
please view the images aswell thanks in advance
![On reading a line, the appropriate type of bus is to be created and added to the arraylist of bus.
LoadBuses should return the full list of buses as specified in the file. The "[L]ist bus" option should
invoke the method listBuses in the reportWriter class, passing System.out as an argument. Listing
the buses should serve as a useful method to determine whether the bus information was
successfully loaded. Task2 assesses whether the program is able to successfully read a generic bus.
Task3 assesses whether the program is able to successfully read a training bus. Task4 assesses
whether the program is able to successfully read a sport bus. Task5 assesses whether the program is
able to successfully read a party bus. Task 6 assesses whether the program is able to successfully read
a combination of bus types.
Please enter the number of the test case to be loaded [ 0..11]:
2
Test case 2 loaded: Show data? [y/n]Y
------Bus List-------
ID:1;BUS_SML; #Price:100; Area:200
ID:2:BUS_BIG;#Price:850; Area:20000
ID
----Planner List------
Name Budget #Plans #Trips
--User Data-
[P]lanner data
[B]us management
[M]inistry interface
[L]oad test case
[Slave test case
[T]est all cases
-System settings------
[I]D:6202398
Code folder:C:\User:
Test case folder:C:
Javadoc folder:C:\U
Update s[y]stem settings
[A]utodetect system settings
E[x]it
B
[A]dd bus
[E]dit/Update bus
[L]ist/Read bus
[D]elete bus
E[x]it](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ca3eaf2-3bda-40e1-af2e-245059bd57cf%2F517dea06-5dee-4abd-8fd8-edce5cc0580b%2Fqzpmz8e_processed.png&w=3840&q=75)
Transcribed Image Text:On reading a line, the appropriate type of bus is to be created and added to the arraylist of bus.
LoadBuses should return the full list of buses as specified in the file. The "[L]ist bus" option should
invoke the method listBuses in the reportWriter class, passing System.out as an argument. Listing
the buses should serve as a useful method to determine whether the bus information was
successfully loaded. Task2 assesses whether the program is able to successfully read a generic bus.
Task3 assesses whether the program is able to successfully read a training bus. Task4 assesses
whether the program is able to successfully read a sport bus. Task5 assesses whether the program is
able to successfully read a party bus. Task 6 assesses whether the program is able to successfully read
a combination of bus types.
Please enter the number of the test case to be loaded [ 0..11]:
2
Test case 2 loaded: Show data? [y/n]Y
------Bus List-------
ID:1;BUS_SML; #Price:100; Area:200
ID:2:BUS_BIG;#Price:850; Area:20000
ID
----Planner List------
Name Budget #Plans #Trips
--User Data-
[P]lanner data
[B]us management
[M]inistry interface
[L]oad test case
[Slave test case
[T]est all cases
-System settings------
[I]D:6202398
Code folder:C:\User:
Test case folder:C:
Javadoc folder:C:\U
Update s[y]stem settings
[A]utodetect system settings
E[x]it
B
[A]dd bus
[E]dit/Update bus
[L]ist/Read bus
[D]elete bus
E[x]it
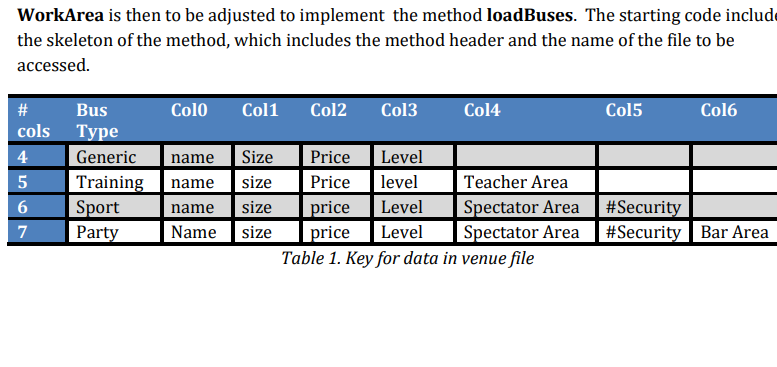
Transcribed Image Text:WorkArea is then to be adjusted to implement the method loadBuses. The starting code include
the skeleton of the method, which includes the method header and the name of the file to be
accessed.
#
cols
4
5
6
7
Colo Col1 Col2 Col3 Col4
Level
level
Bus
Type
Generic
name Size
Training name size
Sport
name size
Party
Name size
Price
Price
Teacher Area
Spectator Area
Spectator Area
price
Level
price Level
Table 1. Key for data in venue file
Col5
Col6
#Security
#Security Bar Area
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
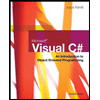
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
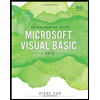
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
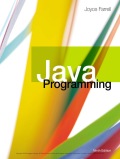
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
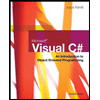
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
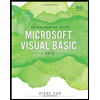
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
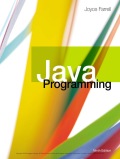
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
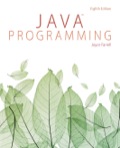
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT