in C# i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. For example, an error message: For Goodnight Moon, ratio is invalid. ...Price is $12.99 for 25 pages. Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page. Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s price to the maximum of 10 cents per page. At the end of the program, display all the entered, and possibly corrected, records. In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US"))); code so far is using System; using static System.Console; using System.Globalization; class BookExceptionDemo { static void Main() { // Your code here } }
in C# i need to
Write the
For Goodnight Moon, ratio is invalid. ...Price is $12.99 for 25 pages.
Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page.
Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s price to the maximum of 10 cents per page. At the end of the program, display all the entered, and possibly corrected, records.
In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US")));
code so far is

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

i keep getting the errors of
Input
Book1
Author1
10.99
200
Book2
Author2
19.99
100
Book3
Author2
25.00
600
Book4
Author3
5.00
20
Book5
Author4
8.00
120
Output
Enter the title of the book: Enter the name of the author: Enter the price of the book:
Unhandled Exception:
BookException: For Book1, ratio is invalid. Price is ¤10.99 for 0 pages.
at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
[ERROR] FATAL UNHANDLED EXCEPTION: BookException: For Book1, ratio is invalid. Price is ¤10.99 for 0 pages.
at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
Results
For Book2, ratio is invalid....Price is $19.99 for 100 pages.For Book4, ratio is invalid....Price is $5.00 for 20 pages.Book1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $10.00 100 pages.Book3 by Author2 Price $25.00 600 pages.Book4 by Author3 Price $2.00 20 pages.Book5 by Author4 Price $8.00 120 pages.
and
Input
Where The Crawdads Sing
Delia Owens
9.59
384
American Dirt
Jeanine Cummins
16.79
400
Golden in Death
J.D. Robb
18.41
400
The Silent Patient
Alex Michaelides
13.49
336
The Dutch House
Ann Patchett
15.99
352
Output
Enter the title of the book: Enter the name of the author: Enter the price of the book:
Unhandled Exception:
BookException: For Where The Crawdads Sing, ratio is invalid. Price is ¤9.59 for 0 pages.
at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
[ERROR] FATAL UNHANDLED EXCEPTION: BookException: For Where The Crawdads Sing, ratio is invalid. Price is ¤9.59 for 0 pages.
at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0
Results
Where The Crawdads Sing by Delia Owens Price $9.59 384 pagesAmerican Dirt by Jeanine Cummins Price $16.79 400 pagesGolden in Death by J.D. Robb Price $18.41 400 pagesThe Silent Patient by Alex Michaelides Price $13.49 336 pagesThe Dutch House by Ann Patchett Price $15.99 352 pages
the last error is
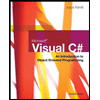
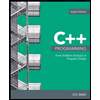
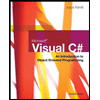
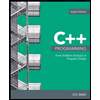