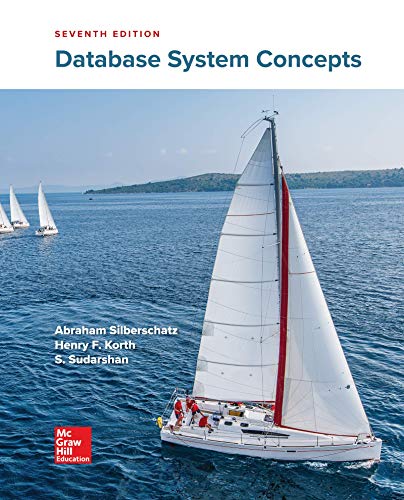
Concept explainers
Java
Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode.
ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example:
for a from 1+1 to c-6
Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names.
Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple:
ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR.
One more thing - type limits. Remember that these are a way to put a range on your variables.
variables numberOfCards : integer from 0 to 52
These apply to integer, real, string. Array already has this built in with the from and to. We can reuse that from/to range. We will have to make a mirror pair (realFrom, realTo - both float) for supporting ranges on real.
Make the variable declaration parser changes and add the floating-point ranges to VariableNode.
Below is what should AST claases and Parse.java must have. Make sure to give full working codes & output:
IfNode: Has constructor(s), ToString, Conditions, Statements, NextIf
WhileNode: Has constructor(s), ToString, Conditions, Statements
ForNode: Has constructor(s), ToString, from, to, Statements
RepeatNode: Has constructor(s), ToString, Conditions, Statements
FunctionCallNode: Has constructor(s), ToString, name, collection of parameters
ParameterNode: Has VariableReferenceNode, Node, constructor(s), ToString()
ParseFor(): Parses complex for statements correctly
ParseWhile(): Parses complex while statements correctly
ParseIf(): Parses complex, chained if statements correctly
ParseFunctionCalls(): Parses complex function calls with expressions and vars correctly
Ranges: Implemented for integers, strings, reals

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode. ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example: for a from 1+1 to c-6 Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names. Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple: ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR. One more thing -…arrow_forwardJava Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardOnly 2arrow_forward
- In Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forwardThe play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…arrow_forwardAssume we have an IntBST class, which implements a binary search tree of integers. The field of the class is a Node variable called root that refers to the root element of the tree. 1) Write a recursive method for this class that computes and returns the sum of all integers less than the root element. Assume the tree is not empty and there is at least one element less than the root. 2) Write a recursive method that prints all of the leaves, and only the leaves, of a binary search tree. 3) Write a method, using recursion or a loop, that returns the smallest element in the tree.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
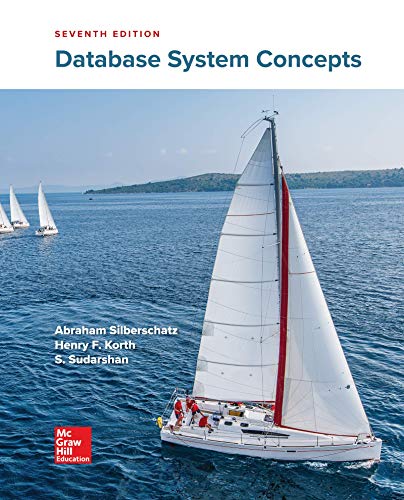
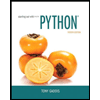
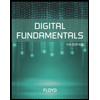
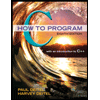
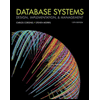
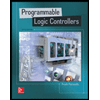