In C++ please replace the evaluate function with a single output statement without changing the program behavior. The simplest solution replaces. evaluate(hselect, rselect); with cout << names[0] << ' ' << outcome[?] << " because " << messages[?] << endl; Other solutions are possible. Write the simplicity solution and the similarity of its output to the original program's output. Criteria compile, run, output the program compiles without error, runs without crashing, and, given the same input, produces the same output as the original program. arrays and statements the solution uses 1d arrays effectively the solution uses 2d arrays effectively the solution replaces the evaluate function with one or more statements containing one or more array references better solutions replace the evaluate function with more array references and fewer statements. the best solution replaces the evaluate function with one statement that contains five array references. Code #include #include #include #include #include #include using namespace std; const int Paper = 0; const int Rock = 1; const int Scissors = 2; const int Human = 0; const int Robot = 1; const int Win = 2; const int Lose = 1; const int Tie = 0; const int nOutcomes = 3; const int nMessages = 4; string objects[] = { "Paper", "Rock", "Scissors" }; string outcome[] = { "ties", "loses", "wins" }; string names[] = { "Hugh", "Roba"}; string messages[]{ "the selections are identical", "paper covers rock", "rock breaks scissors", "scissors cut paper" }; int outcome_map[][nOutcomes] { {0, 2, 1}, {1, 0, 2}, {2, 1, 0} }; int messages_map[][nOutcomes]{ {0, 1, 3}, {1, 0, 2}, {3, 2, 0} }; default_random_engine e((unsigned)time(nullptr)); uniform_int_distribution u(Paper, Scissors); int humanSelection(); int robotSelection(); void evaluate(int human_selection, int robot_selection); int main() { int hselect, rselect; do { hselect = humanSelection(); rselect = robotSelection(); cout << names[Human] << " selects " << objects[hselect] << endl; cout << names[Robot] << " selects " << objects[rselect] << endl; evaluate(hselect, rselect); } while (hselect == rselect); } void evaluate(int human_selection, int robot_selection) { int state = nOutcomes * human_selection + robot_selection; switch (state) { case 0: case 4: case 8: cout << names[0] << ' ' << outcome[0] << " because " << messages[0] << endl; break; case 1: cout << names[0] << ' ' << outcome[2] << " because " << messages[1] << endl; break; case 5: cout << names[0] << ' ' << outcome[2] << " because " << messages[2] << endl; break; case 6: cout << names[0] << ' ' << outcome[2] << " because " << messages[3] << endl; break; case 2: cout << names[0] << ' ' << outcome[1] << " because " << messages[3] << endl; break; case 3: cout << names[0] << ' ' << outcome[1] << " because " << messages[1] << endl; break; case 7: cout << names[0] << ' ' << outcome[1] << " because " << messages[2] << endl; break; default: cout << "Programming error" << endl; } } int robotSelection() { return u(e); } int humanSelection() { string object; do { cout << "Enter P to select paper, R rock, or S scissors: "; getline(cin, object); string::const_iterator bit = find_if_not(object.cbegin(), object.cend(), isspace); object.erase(object.begin(), bit); string::const_iterator eit = find_if_not(object.cbegin(), object.cend(), isalpha); object.erase(eit, object.end()); transform(object.begin(), object.end(), object.begin(), toupper); } while (object.length() == 0 || !(object[0] == 'P' || object[0] == 'R' || object[0] == 'S')); return object[0] == 'P' ? Paper : object[0] == 'R' ? Rock : Scissors; }
In C++ please replace the evaluate function with a single output statement without changing the program behavior.
The simplest solution replaces.
evaluate(hselect, rselect);
with
cout << names[0] << ' ' << outcome[?] << " because " << messages[?] << endl;
Other solutions are possible. Write the simplicity solution and the similarity of its output to the original program's output.
Criteria
compile, run, output
the program compiles without error, runs without crashing, and, given the same input, produces the same output as the original program.
arrays and statements
the solution uses 1d arrays effectively
the solution uses 2d arrays effectively
the solution replaces the evaluate function with one or more statements containing one or more array references
better solutions replace the evaluate function with more array references and fewer statements.
the best solution replaces the evaluate function with one statement that contains five array references.
Code
#include <algorithm>
#include <ctime>
#include <iostream>
#include <random>
#include <string>
#include <iterator>
using namespace std;
const int Paper = 0;
const int Rock = 1;
const int Scissors = 2;
const int Human = 0;
const int Robot = 1;
const int Win = 2;
const int Lose = 1;
const int Tie = 0;
const int nOutcomes = 3;
const int nMessages = 4;
string objects[] = { "Paper", "Rock", "Scissors" };
string outcome[] = { "ties", "loses", "wins" };
string names[] = { "Hugh", "Roba"};
string messages[]{ "the selections are identical", "paper covers rock", "rock breaks scissors", "scissors cut paper" };
int outcome_map[][nOutcomes] { {0, 2, 1}, {1, 0, 2}, {2, 1, 0} };
int messages_map[][nOutcomes]{ {0, 1, 3}, {1, 0, 2}, {3, 2, 0} };
default_random_engine e((unsigned)time(nullptr));
uniform_int_distribution<int> u(Paper, Scissors);
int humanSelection();
int robotSelection();
void evaluate(int human_selection, int robot_selection);
int main()
{
int hselect, rselect;
do
{
hselect = humanSelection();
rselect = robotSelection();
cout << names[Human] << " selects " << objects[hselect] << endl;
cout << names[Robot] << " selects " << objects[rselect] << endl;
evaluate(hselect, rselect);
}
while (hselect == rselect);
}
void evaluate(int human_selection, int robot_selection)
{
int state = nOutcomes * human_selection + robot_selection;
switch (state)
{
case 0:
case 4:
case 8:
cout << names[0] << ' ' << outcome[0] << " because " << messages[0] << endl;
break;
case 1:
cout << names[0] << ' ' << outcome[2] << " because " << messages[1] << endl;
break;
case 5:
cout << names[0] << ' ' << outcome[2] << " because " << messages[2] << endl;
break;
case 6:
cout << names[0] << ' ' << outcome[2] << " because " << messages[3] << endl;
break;
case 2:
cout << names[0] << ' ' << outcome[1] << " because " << messages[3] << endl;
break;
case 3:
cout << names[0] << ' ' << outcome[1] << " because " << messages[1] << endl;
break;
case 7:
cout << names[0] << ' ' << outcome[1] << " because " << messages[2] << endl;
break;
default:
cout << "
}
}
int robotSelection()
{
return u(e);
}
int humanSelection()
{
string object;
do
{
cout << "Enter P to select paper, R rock, or S scissors: ";
getline(cin, object);
string::const_iterator bit = find_if_not(object.cbegin(), object.cend(), isspace);
object.erase(object.begin(), bit);
string::const_iterator eit = find_if_not(object.cbegin(), object.cend(), isalpha);
object.erase(eit, object.end());
transform(object.begin(), object.end(), object.begin(), toupper);
} while (object.length() == 0 || !(object[0] == 'P' || object[0] == 'R' || object[0] == 'S'));
return object[0] == 'P' ? Paper : object[0] == 'R' ? Rock : Scissors;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

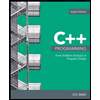
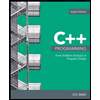