In java please Create a splice method from the implementation perspective. 2nd queue should not be altered when complete. Try to account for speical cases like empty and singleton queues. method header: public void splice(LinkedQueue secondQueue)
In java please Create a splice method from the implementation perspective. 2nd queue should not be altered when complete. Try to account for speical cases like empty and singleton queues. method header: public void splice(LinkedQueue secondQueue)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In java please
Create a splice method from the implementation perspective.
2nd queue should not be altered when complete. Try to account for speical cases like empty and singleton queues.
method header:
public void splice(LinkedQueue<T> secondQueue)

Transcribed Image Text:61
current
current.next;
}
System.out.println();
}
62
63
64
65
public void splice(LinkedQueue<T> anotherQueue) {
// YOUR CODE HERE!
}
66
67
68
69
public T getSecond() {
// YOUR EXTRA CREDIT CODE HERE!
return null; // placeholder: replace with your own code
}
70
71
72
73
74
75
{
public class Node implements java.io.Serializable
public T data; // entry in queue
public Node next; // link to next node
76
77
78
79
private Node(T dataPortion) {
data = dataPortion;
next = null;
}
80
81
82
83
84
private Node(T dataPortion, Node linkPortion){
data = dataPortion;
next = linkPortion;
}
85
86
87
88
89
private T getData() {
return data;
}
90
91
92
93
private void setData(T newData) {
data = newData;
}
94
95
96
97
private Node getNextNode() {
return next;
}
98
99
100
101
{
private void setNextNode(Node nextNode)
next = nextNode;
}
}
102
103
104
105
106
107
}
Line 1, Column 1
Tab Size: 4
Java

Transcribed Image Text:1
public class LinkedQueue<T> implements QueueInterface<T>, java.io.Serializable {
2
public Node firstNode; // references node at front of queue
public Node lastNode; // references node at back of queue
3
4
public LinkedQueue()
firstNode = null;
lastNode = null;
}
{
7
10
public void enqueue(T newEntry)
Node newNode = new Node(newEntry, null);
11
{
12
13
if (isEmpty()) {
firstNode = newNode;
} else {
lastNode.setNextNode(newNode);
}
14
15
16
17
18
19
20
lastNode
newNode;
21
}
22
public T getFront()
I front = null;
23
{
24
25
if (!isEmpty()) {
front
}
26
27
firstNode.getData();
28
29
return front;
}
30
31
32
public T dequeue() {
I front
33
34
null;
35
if (!isEmpty()) {
front =
firstNode =
36
firstNode.getData();
firstNode.getNextNode( );
37
38
39
if (firstNode
lastNode = null;
}
}
40
null) {
41
42
43
44
return front;
}
45
46
47
public boolean isEmpty()
return (firstNode
}
48
{
49
null) && (lastNode
null);
50
51
public void clear() {
firstNode = null;
lastNode = null;
}
52
53
54
55
56
public void display() {
firstNode;
57
Node current =
while(current!=null) {
System.out.print(current.data +
current
58
59
60
");
%3D
61
current.next;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
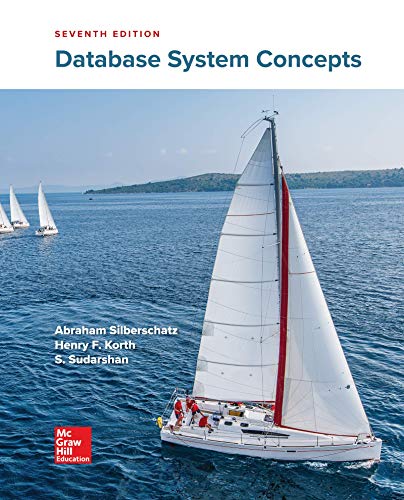
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
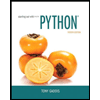
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
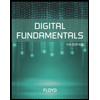
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
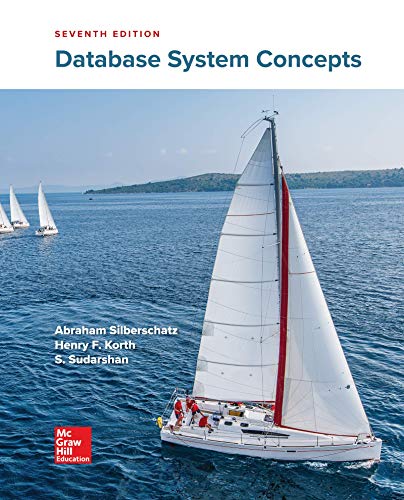
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
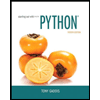
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
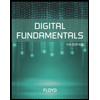
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
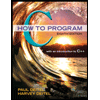
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
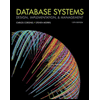
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
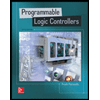
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education