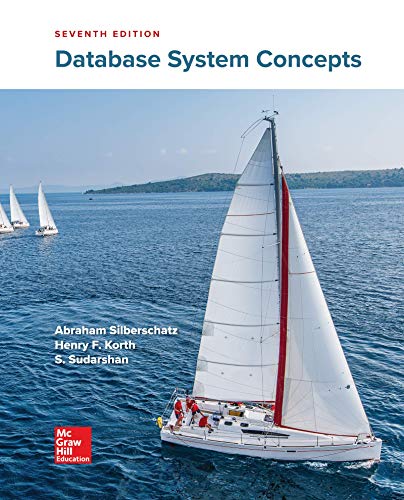
in kotlin
Write two versions of a recursive palindrome check function. One version should have a block body, and the other should have an expression body. Review the lecture slide about the substring function first. Use this
if the length of the string is less than 2, return true
else if the first and last characters are not equal (!=) return false
else return the result of a recursive call with the substring from the second character (the one at index 1) to the second to last character.
Use a main that uses a loop to test the palindrome function using this list of strings:
val ss = listOf("", "A", "AA", "AB", "AAA", "ABA", "ABB", "AAAA", "AABA", "ABBA", "ABCBA", "ABCAB")

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Using recursive functions in Python, given three letters in the alphabet, get their permutations together with the combinations of any two letters from it.arrow_forwardIn kotlin, Write a recursive linear search function that takes a String and a Char and returns true if the String contains the Char. The base cases are that a) the length of the String is 0 or b) the first character in the String is the character we are searching for. then Modify the linear search function so that both the String and the Char are nullable. If either is null, return false.arrow_forwardWrite a recursive function called Rev takes a string argument (str) and and integerargument(i – the initial string position). Rev prints the str in reverse. You may not useany built-in reverse function or method. You may use string’s length method.arrow_forward
- Make a code using Recursion The countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive. For example: countSubstring(“catwoman loves cats”, “cat”) would return 2 countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself. public static int countSubstring(String s, String x) { if (s.length() == 0 || x.length() == 0) return 1; if (s.length() == 1 || x.length() == 1){ if (s.substring(0,1).equals(x.substring(0,1))){ s.replaceFirst((x), " "); return 1 + countSubstring(s.substring(1), x); } else { return 0 + countSubstring(s.substring(1), x); } } return countSubstring(s.substring(0,1), x) + countSubstring(s.substring(1), x); } public class Main { public static void main(String[] args) {…arrow_forwardWrite a recursive function to see if the first letter matches the last letter, return the middle letters and check until only 0 or 1 letters are left. It returns True or False. Here is the original code that needs to be checked: # Returns the first character of the string str def firstCharacter(str): return str[:1] # Returns the last character of a string str def lastCharacter(str): return str[-1:] # Returns the string that results from removing the first # and last characters from str def middleCharacters(str): return str[1:-1] def isPalindrome(str): # base case #1 # base case #2 # recursive case pass Python codearrow_forwardThe 4th problem mimics the situation where eagles flying in the sky can be spotted and counted.FindEagles: a recursive function that examines and counts the number of objects (eagles) in aphotograph. The data is in a two-dimensional grid of cells, each of which may be empty (value 0) orfilled (value 1 to 9). Maximum grid size is 50 x 50. The filled cells that are connected form an object(eagle). Two cells are connected if they are vertically, horizontally, or diagonally adjacent. Thefollowing figure shows 3 x 4 grids with 3 eagles. 0 0 1 21 0 0 01 0 3 1 FindEagle function takes as parameters the 2-D array and the x-y coordinates of a cell that is a part ofan eagle (non-zero value) and erases (change to 0) the image of an eagle. The function FindEagleshould return an integer value that counts how many cells has been counted as part of an eagle and havebeen erased. The following sample data has two pictures, the first one is 3 x 4, and the second one is 5 x 5 grids. Notethat your program…arrow_forward
- In Kotliln, Write a recursive linear search function that takes a String and a Char and returns true if the String contains the Char. The base cases are that a) the length of the String is 0 or b) the first character in the String is the character we are searching for.arrow_forwardWrite a recursive method that will return the number of vowels in a given string.arrow_forwardWrite a statement that calls the recursive function backwards_alphabet() with input starting_letter.arrow_forward
- ANSWER SHOULD BE WRITTEN IN JAVA CODE USING RECURSION Problem Statement Given a string as input return true or false depending on whether it satisfies these rules: 1) The string begins with an 'x' 2) Each 'x' is followed by nothing, 'x' or 'yy' 3) Each 'yy' is followed by nothing or 'x'arrow_forwardsolve q5 only pleasearrow_forwardWrite a recursive function to implement the recursive algorithm (multiplying two positive integers using repeated addition). Also, write a program to test your function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
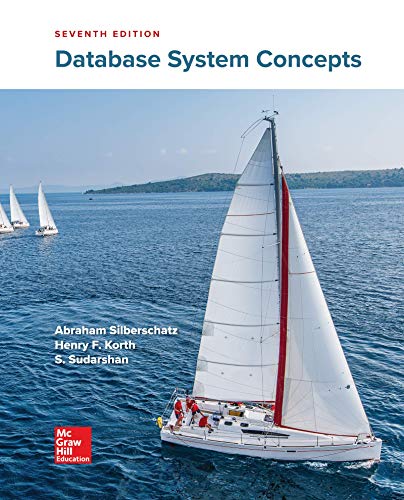
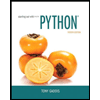
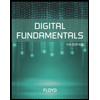
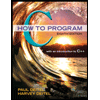
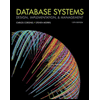
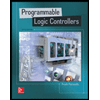