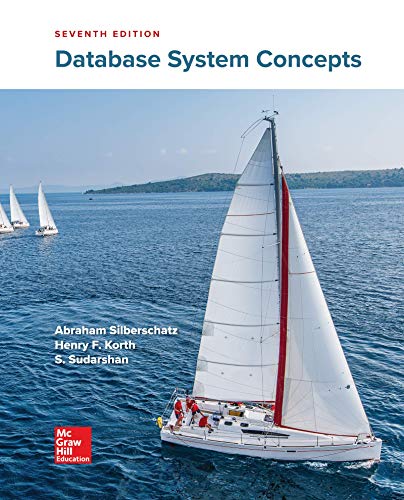
Concept explainers
JAVA PROGRAM ASAP
The program down below does now work in hypergrade please modify it or create a new program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test case as well as the failed test case as a screenshot. It must pass all the test cases because it says 0 out of 2 passed when I upload it to Hypergrade. The program must pass the test case when uploaded to Hypergrade. Thank you
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class MorseCodeConverter {
public static void main(String[] args) {
Map<String, String> morseCodeMap = readMorseCodeTable("Morse.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter the file name or type QUIT to exit:\n");
String fileName = scanner.nextLine().trim();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
String text = convertMorseCodeToText(fileName, morseCodeMap);
System.out.println(text);
} catch (IOException e) {
System.out.println("File '" + fileName + "' is not found.");
}
}
scanner.close();
}
private static Map<String, String> readMorseCodeTable(String fileName) {
Map<String, String> morseCodeMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\s+");
if (parts.length == 2) {
morseCodeMap.put(parts[1], parts[0]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return morseCodeMap;
}
private static String convertMorseCodeToText(String fileName, Map<String, String> morseCodeMap) throws IOException {
StringBuilder result = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] morseWords = line.split("\\s{3,}");
for (String morseWord : morseWords) {
String[] morseLetters = morseWord.split("\\s+");
for (String morseLetter : morseLetters) {
if (morseCodeMap.containsKey(morseLetter)) {
result.append(morseCodeMap.get(morseLetter));
}
}
}
result.append("\n");
}
}
return result.toString().trim();
}
}
File data down below:
Morse.txt
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..
input1.txt
- .... . --- .-. .. --. .. -. .- .-.. --.- ..- . ... - .. --- -. --..--
-.-. .- -. -- .- -.-. .... .. -. . ... - .... .. -. -.- ..--..
.. -... . .-.. .. . ...- . - --- -... . - --- --- -- . .- -. .. -. --. .-.. . ... ... - --- -.. . ... . .-. ...- . -.. .. ... -.-. ..- ... ... .. --- -. .-.-.-
.- .-.. .- -. - ..- .-. .. -. --.
Test Case 1
input1.txtENTER
THEORIGINALQUESTION,\n
CANMACHINESTHINK?\n
IBELIEVETOBETOOMEANINGLESSTODESERVEDISCUSSION.\n
ALANTURING\n
Test Case 2
input2.txtENTER
File 'input2.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
quitENTER
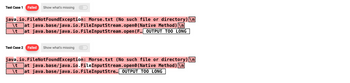
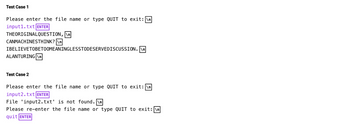

Step by stepSolved in 4 steps with 3 images

- Make the code below offer three ways of to display output.GUI window or Print on screen or save to text file. import randomclass Circle(): def __init__(self, radius=1.0): self.__radius = radius self.__area = self.find_area() def get_radius(self): return self.__radius def set_radius(self, radius): self.__radius = radius def find_area(self): self.__area = 3.14159265 * self.__radius ** 2 return self.__area def display(self): self.find_area() print('Circle, Area of Circle: {:.2f}'.format(self.__area))class Square(): def __init__(self, side=1.0): self.__side = side self.__area = self.find_area() def get_side(self): return self.__side def set_side(self, side): self.__side = side def find_area(self): self.__area = self.__side ** 2 return self.__area def display(self): self.find_area() print('Square, Area of Square: {:.2f}'.format(self.__area))class Cube(): def…arrow_forwardExpert this is warming, don't use any AI platform and don't give plagiarised response to me. If I see plagiarism and AI detection I'll report your account and reduce rating.You have a basic User class for a social media platform. Your task is to enhance the class by introducing a new method called 'post_status.' This method should take a single parameter, which is the status message that the user wants to post. The objective is to implement this method to update the user's status.Next, instantiate a new object of the User class, naming it 'SocialUser.' Utilize the extended class with the newly added 'post_status' method. Your next step is to post three distinct status messages for 'SocialUser'—namely, 'Excited for the weekend!', 'Just finished a great book!', and 'Exploring new hobbies!'To conclude, utilize the 'display_info' method to showcase the updated information about 'SocialUser.' Verify that the output includes details about the newly added status messages, ensuring that they…arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Which option of the setDefaultCloseOperation() method can ensure that Java application willbe properly terminated when the user attempts to close the "form"? A. HALT_ON_CLOSEB. TERMINATE_ON_CLOSEC. EXIT_ON_CLOSED. DELETE_ON_CLOSEE. DESTROY_ON_CLOSE Part 2 - In Java, which can ensure the "Form" class to inherit from JFrame class? A. public class Form gets JFrame { }B. public class Form inherits JFrame { }C. public class Form extends JFrame { }D. public class Form : public JFrame { }E. public class Form implements JFrame { }arrow_forward
- create an image file programmatically. Your image file should be called OnTheFlyPy.ppm and the code you write to create should be in a public static void makePPM() method defined in the provided Main.java class. Your OnTheFlyPy.ppm should be be 100 pixels wide and 100 pixels tall and the PPM header lines should be correct. The first two rows and last two rows in the OnTheFlyPy.ppm should be red (Red=255, Green=0, Blue= 0). The first two columns and last two columns in the OnTheFlyPy.ppm (except for the part that overlaps with the red pixels you created in the previous part) should should be blue (Red=0, Green=0, Blue= 255). The remaining pixels in your OnTheFlyPy.ppm image should be white (Red=255, Green=255, Blue= 255). Here is a picture of what your final image should look like: import java.io.*; public class Main{ public static void main(String[] args) { makePPM(); } public static void makePPM() { } }arrow_forwardJAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 0 OUT OF 1 TEST CASES. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); String fileName; System.out.println("Please enter the file name or type QUIT to exit:"); fileName = keyboard.nextLine().trim(); while (!fileName.equalsIgnoreCase("QUIT")) { File file = new File(fileName); if (file.exists()) { int wordCount = countWords(file); System.out.println("Total number of words: " + wordCount + "\n);…arrow_forwardJAVA PROGRAM ASAP There is an extra space in the program down below as shown in the screesshot. Please modify this program even more ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade. import java.util.HashMap;import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;public class MorseEncoder { private static HashMap<Character, String> codeMappings = new HashMap<>(); public static void main(String[] args) { initializeMappings(); Scanner textScanner = new Scanner(System.in); System.out.println("Please enter a string to convert to Morse code:"); String textForEncoding = textScanner.nextLine().toUpperCase(); if ("ENTER".equals(textForEncoding)) { System.out.println(); return; } String encodedOutput = encodeText(textForEncoding);…arrow_forward
- JAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 1 OUT OF 7 TEST CASES. I have provided the failed the test cases as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); String fileName; while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); fileName = keyboard.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; // Exit the loop when "QUIT" is entered } File file = new File(fileName); if (file.exists()) { int wordCount = countWords(file);…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The text files are located in Hypergrade. The program down below does not work in Hypergrade and does not pass the test cases. Please modify it or create a new program so when I upload to hypergrade it passes the test cases. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it…arrow_forwardAccording to the below screenshot of an app, build an Android App that has a set of java files that implement an adapter with RecyclerView. The details of the java files you need to create are described below. The first java file should contain a data field which is in this case: Movie_name, Director_name, Production_year. The second java file contains MovieViewHolder. The third java file contains the MovieAdapter which is act as a bridge between the data items and the View inside of RecycleView. The Mainactivity java file will contain some samples data to display.arrow_forward
- Java Proram ASAP There is an extra /n in the program in test case 1-3 and after Please re-enter the file name or type QUIT to exit:\n quitENTER in test case 4 there needs to be nothing as shown in the screenshot. The text files are located in Hypergrade. Please modify this code below so it passes the test cases. Also I have the correct test case as a screenshot. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if…arrow_forwardCreate a simple chat application using Java programming to allow two clients to communicate with one another by sending a message to the server and having it transfer the message. The following needs to be implemented: Two classes with one named server and the other named client. Server can allow the clients to create their own nicknames before entering the chatroom. Add a ".exit" command to allow the users to disconnect after they are done chatting. In order to understand exactly what the program is doing, please provide both comments and screenshots for the codes including the input/output. If there are similar questions that have been asked before, please do not copy the same answers. Take your time creating the application. Just have it successfully run in the Java software you are using.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
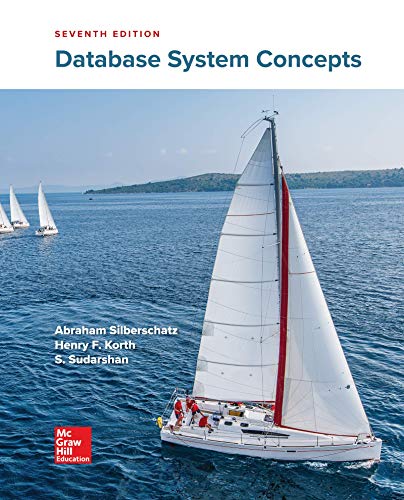
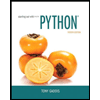
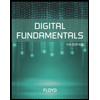
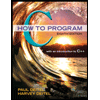
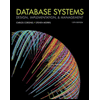
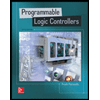