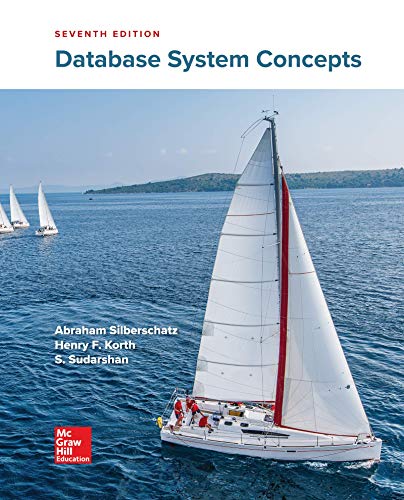
Concept explainers
JAVA PROGRAM ASAP
Please modify or create a new program ASAP BECAUSE hypergrade does not like the program down below and the progran does not pass all the test cases when I upload it to hypergrade. I have provided the correct test case as well as the failed test case as a screenshot. It must pass all the test cases because it says 0 out of 2 passed when I upload it to Hypergrade. The program must pass the test case when uploaded to Hypergrade. Thank you
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class MorseCodeConverter {
public static void main(String[] args) {
Map<String, String> morseCodeMap = readMorseCodeTable("Morse.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter the file name or type QUIT to exit:\n");
String fileName = scanner.nextLine().trim();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
String text = convertMorseCodeToText(fileName, morseCodeMap);
System.out.println(text);
} catch (IOException e) {
System.out.println("File '" + fileName + "' is not found.");
}
}
scanner.close();
}
private static Map<String, String> readMorseCodeTable(String fileName) {
Map<String, String> morseCodeMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\s+");
if (parts.length == 2) {
morseCodeMap.put(parts[1], parts[0]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return morseCodeMap;
}
private static String convertMorseCodeToText(String fileName, Map<String, String> morseCodeMap) throws IOException {
StringBuilder result = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] morseWords = line.split("\\s{3,}");
for (String morseWord : morseWords) {
String[] morseLetters = morseWord.split("\\s+");
for (String morseLetter : morseLetters) {
if (morseCodeMap.containsKey(morseLetter)) {
result.append(morseCodeMap.get(morseLetter));
}
}
}
result.append("\n");
}
}
return result.toString().trim();
}
}
File data down below:
Morse.txt
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..
input1.txt
- .... . --- .-. .. --. .. -. .- .-.. --.- ..- . ... - .. --- -. --..--
-.-. .- -. -- .- -.-. .... .. -. . ... - .... .. -. -.- ..--..
.. -... . .-.. .. . ...- . - --- -... . - --- --- -- . .- -. .. -. --. .-.. . ... ... - --- -.. . ... . .-. ...- . -.. .. ... -.-. ..- ... ... .. --- -. .-.-.-
.- .-.. .- -. - ..- .-. .. -. --.
Test Case 1
input1.txtENTER
THEORIGINALQUESTION,\n
CANMACHINESTHINK?\n
IBELIEVETOBETOOMEANINGLESSTODESERVEDISCUSSION.\n
ALANTURING\n
Test Case 2
input2.txtENTER
File 'input2.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
quitENTER
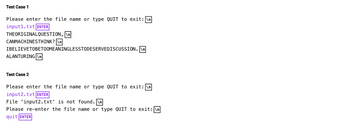
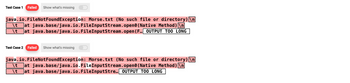

Step by stepSolved in 4 steps with 3 images

- user story: place order as a new customer, i want to place a coffee order using the web application, so that i can buy coffee. acceptance criteria: the user can log in to the application. the user can access their account and place an order. tasks: when the user logs in, take them to a page that allows them to place a coffee order. Create an cart.js script and reference it in the page. In this script, create a class that represents an order. it should have a date, product ( you will need to create another class for this that contains the name and price), size (could be small, medium or large) and quantity. As the user selects coffee items, update a cart with this script. Use an array to or web storage to store the items they select. Implement a checkout page that displays the items they ordered. The checkout page should display the total they need to pay.arrow_forwardPlease the code in Java eclipse. Please add comments for each instructions given in the image. I'll appreciate your help. And send the screenshoot of the output.arrow_forwardJAVA PROGRAM ASAP The program down below does now work in hypergrade please modify it or create a new program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test case as well as the failed test case as a screenshot. It must pass all the test cases because it says 0 out of 2 passed when I upload it to Hypergrade. The program must pass the test case when uploaded to Hypergrade. Thank you Chapter 9. PC #16. Morse Code Translator (modified *** Read carefully ***) Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Write a program that asks the user to enter a file name containing morse code, and then converts that code to text and prints it on the screen. The Morse code table is given in a text file morse.txt. When printing resulting text, display one sentence on each line. There should be no extra spaces at the…arrow_forward
- in java but don't write as a GUI (Graphical User Interface) Write a program that reads a file named input.txt and writes a file that contains the same contents, but is named output.txt. The input file will contain more than one line when I test this and so should your output file. Do not use a path name when opening these files. This means the files should be located in the top level folder of the project. This would be the folder that contains the src folder, probably named FileCopy depending on what name you gave the project. Do not use a copy method that is supplied by Java. Your program must read the file line by line and write the file itself. Class should be named FileCopy do not write to input.txt!arrow_forward2. Create a simple program that includes JFrame, JPanel, JLabel, JFields, JTextArea, and JButton. The program should ask the user for the following input:• First name• Last name• Middle name• Mobile number• E-mail address.3. Use the following methods and classes in creating the program: 4. Two (2) frames will be used in this program: OUTPUT and INPUT. The following conditions must be satisfied by the program.Input Frame: • Set the window name to INPUT.• The input frame should collect all the details listed on Step 2.• It should contain Submit and Clear All buttons.• When the Submit button is clicked, it should generate the output frame and disable the Submit button.• When the Clear All button is clicked, it should clear all the content that were entered by the user in the input frame, and close the output frame if it is open. Output Frame: • Set the window name to OUTPUT.• The output frame should display all the details that were entered by the user with the corresponding label.• The…arrow_forwardJAVA PROGRAM ASAP The program does not run in hypergrade can you please MODIFY THIS program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test cases as a screenshot as well as the failed test cases. It says 0 out of 4 passed. The program must pass the test case when uploaded to Hypergrade. Files data are down below: text1.txt StopAndSmellTheRoses. text2.txt ATrueRebelYouAre!EveryoneWasImpressed.You'llDoWellToContinueInTheSameSpirit.PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;class Main { // Driver code public static void main(String[] args) { // scanner object used to take user input Scanner sc = new Scanner(System.in); // loop iterates until user enters "quit" or "QUIT" while (true) { System.out.print("Please enter…arrow_forward
- Make the code below offer three ways of to display output.GUI window or Print on screen or save to text file. import randomclass Circle(): def __init__(self, radius=1.0): self.__radius = radius self.__area = self.find_area() def get_radius(self): return self.__radius def set_radius(self, radius): self.__radius = radius def find_area(self): self.__area = 3.14159265 * self.__radius ** 2 return self.__area def display(self): self.find_area() print('Circle, Area of Circle: {:.2f}'.format(self.__area))class Square(): def __init__(self, side=1.0): self.__side = side self.__area = self.find_area() def get_side(self): return self.__side def set_side(self, side): self.__side = side def find_area(self): self.__area = self.__side ** 2 return self.__area def display(self): self.find_area() print('Square, Area of Square: {:.2f}'.format(self.__area))class Cube(): def…arrow_forwardCreate a program using classes that does the following in the zyLabs developer below. For this lab, you will be working with two different class files. To switch files, look for where it says "Current File" at the top of the developer window. Click the current file name, then select the file you need.(1) Create two files to submit: ItemToPurchase.java - Class definition ShoppingCartPrinter.java - Contains main() method Build the ItemToPurchase class with the following specifications: Private fields String itemName - Initialized in default constructor to "none" int itemPrice - Initialized in default constructor to 0 int itemQuantity - Initialized in default constructor to 0 Default constructor Public member methods (mutators & accessors) setName() & getName() setPrice() & getPrice() setQuantity() & getQuantity() (2) In main(), prompt the user for two items and create two objects of the ItemToPurchase class. Before prompting for the second item, call…arrow_forwardcreate an image file programmatically. Your image file should be called OnTheFlyPy.ppm and the code you write to create should be in a public static void makePPM() method defined in the provided Main.java class. Your OnTheFlyPy.ppm should be be 100 pixels wide and 100 pixels tall and the PPM header lines should be correct. The first two rows and last two rows in the OnTheFlyPy.ppm should be red (Red=255, Green=0, Blue= 0). The first two columns and last two columns in the OnTheFlyPy.ppm (except for the part that overlaps with the red pixels you created in the previous part) should should be blue (Red=0, Green=0, Blue= 255). The remaining pixels in your OnTheFlyPy.ppm image should be white (Red=255, Green=255, Blue= 255). Here is a picture of what your final image should look like: import java.io.*; public class Main{ public static void main(String[] args) { makePPM(); } public static void makePPM() { } }arrow_forward
- JAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 0 OUT OF 1 TEST CASES. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); String fileName; System.out.println("Please enter the file name or type QUIT to exit:"); fileName = keyboard.nextLine().trim(); while (!fileName.equalsIgnoreCase("QUIT")) { File file = new File(fileName); if (file.exists()) { int wordCount = countWords(file); System.out.println("Total number of words: " + wordCount + "\n);…arrow_forwardThis project utilizes three new classes: · Word - an immutable class representing a word · Words - a class representing a list of Word objects · WordTester - a class used to test the Word and Words classes. WordTester (the application) is complete and only requires uncommenting to test the Word and Words classes as they are completed. The needed imports, class headings, method headings, and block comments are provided for the remaining classes. Javadocs are also provided for the Word and Words classes. Word The Word class represents a single word. It is immutable. You have been provided a skeleton for this class. Complete the Word class using the block comments, Javadocs, and the following instructions. 1. Add a String instance variable named word which will contain the word. 2. Complete the one parameter constructor to initialize the word instance variable. 3. Complete the getLength method to return the number of characters in the…arrow_forwardJAVA PROGRAM ASAP There is an extra space in the program down below as shown in the screesshot. Please modify this program even more ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade. import java.util.HashMap;import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;public class MorseEncoder { private static HashMap<Character, String> codeMappings = new HashMap<>(); public static void main(String[] args) { initializeMappings(); Scanner textScanner = new Scanner(System.in); System.out.println("Please enter a string to convert to Morse code:"); String textForEncoding = textScanner.nextLine().toUpperCase(); if ("ENTER".equals(textForEncoding)) { System.out.println(); return; } String encodedOutput = encodeText(textForEncoding);…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
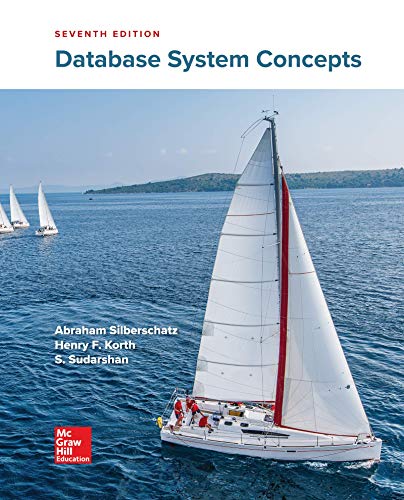
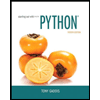
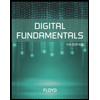
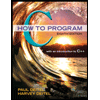
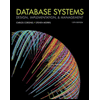
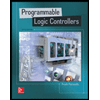