In python, please complete both sections Section 1: The Course class A simple class that stores the id, name, and number of credits for a class. Attributes Type Name Description str cid Stands for course id, uniquely identifies a course like “CMPSC132”. str cname Stands for course name and is the long form of the course title. int credits The number of credits a course is worth. Special methods Type Name Description str __str__(self) Returns a formatted summary of the course as a string. str __repr__(self) Returns the same formatted summary as __str__. bool __eq__(self, other) Does an equality check based only on course id. __str__(self), __repr__(self) Returns a formatted summary of the course as a string. The format to use is: cid(credits): cname Output str Formatted summary of the course. __eq__(self, other) Determines if two objects are equal. For instances of this class, we will define equality when the course id of one object is the same as the course id of the other object. You can assume at least one of the objects is a Couse object. Input (excluding self) any other The object to check for equality against a Course object. Output bool True if other is a Course object with the same course id, False otherwise. Section 2: The Catalog class Stores a collection of Course objects and their capacity as a dictionary, accessible by their ids. Attributes Type Name Description dict courseOfferings Stores courses with the id as the key and a tuple (Course, capacity) as the value. Methods Type Name Description str addCourse(self, cid, cname, credits, capacity) Adds a course with the given information. str removeCourse(self, cid) Removes a course with the given id. addCourse(self, cid, cname, credits, capacity) Creates a Course object with the parameters and stores it as a value in courseOfferings. Inputs (excluding self) str cid The id of the course to add. str cname The name of the course to add int credits The number of credits the course is worth. int capacity The maximum number of students allowed to register in the course Output str “Course added successfully” str “Course already added” if course is already in courseOfferings. removeCourse(self, cid) Removes a course with the given id. Input (excluding self) str cid The id of the course to remove. Output str “Course removed successfully” str“Course not found” if a course with the given id is not in the dictionary.
In python, please complete both sections Section 1: The Course class A simple class that stores the id, name, and number of credits for a class. Attributes Type Name Description str cid Stands for course id, uniquely identifies a course like “CMPSC132”. str cname Stands for course name and is the long form of the course title. int credits The number of credits a course is worth. Special methods Type Name Description str __str__(self) Returns a formatted summary of the course as a string. str __repr__(self) Returns the same formatted summary as __str__. bool __eq__(self, other) Does an equality check based only on course id. __str__(self), __repr__(self) Returns a formatted summary of the course as a string. The format to use is: cid(credits): cname Output str Formatted summary of the course. __eq__(self, other) Determines if two objects are equal. For instances of this class, we will define equality when the course id of one object is the same as the course id of the other object. You can assume at least one of the objects is a Couse object. Input (excluding self) any other The object to check for equality against a Course object. Output bool True if other is a Course object with the same course id, False otherwise. Section 2: The Catalog class Stores a collection of Course objects and their capacity as a dictionary, accessible by their ids. Attributes Type Name Description dict courseOfferings Stores courses with the id as the key and a tuple (Course, capacity) as the value. Methods Type Name Description str addCourse(self, cid, cname, credits, capacity) Adds a course with the given information. str removeCourse(self, cid) Removes a course with the given id. addCourse(self, cid, cname, credits, capacity) Creates a Course object with the parameters and stores it as a value in courseOfferings. Inputs (excluding self) str cid The id of the course to add. str cname The name of the course to add int credits The number of credits the course is worth. int capacity The maximum number of students allowed to register in the course Output str “Course added successfully” str “Course already added” if course is already in courseOfferings. removeCourse(self, cid) Removes a course with the given id. Input (excluding self) str cid The id of the course to remove. Output str “Course removed successfully” str“Course not found” if a course with the given id is not in the dictionary.
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
In python, please complete both sections
Section 1: The Course class
A simple class that stores the id, name, and number of credits for a class.
Attributes
Type Name Description
str cid Stands for course id, uniquely identifies a course like “CMPSC132”.
str cname Stands for course name and is the long form of the course title.
int credits The number of credits a course is worth.
Special methods
Type Name Description
str __str__(self) Returns a formatted summary of the course as a string.
str __repr__(self) Returns the same formatted summary as __str__.
bool __eq__(self, other) Does an equality check based only on course id.
__str__(self), __repr__(self)
Returns a formatted summary of the course as a string.
The format to use is: cid(credits): cname
Output
str Formatted summary of the course.
__eq__(self, other)
Determines if two objects are equal. For instances of this class, we will define equality when the
course id of one object is the same as the course id of the other object. You can assume at least
one of the objects is a Couse object.
Input (excluding self)
any other The object to check for equality against a Course object.
Output
bool True if other is a Course object with the same course id, False otherwise.
A simple class that stores the id, name, and number of credits for a class.
Attributes
Type Name Description
str cid Stands for course id, uniquely identifies a course like “CMPSC132”.
str cname Stands for course name and is the long form of the course title.
int credits The number of credits a course is worth.
Special methods
Type Name Description
str __str__(self) Returns a formatted summary of the course as a string.
str __repr__(self) Returns the same formatted summary as __str__.
bool __eq__(self, other) Does an equality check based only on course id.
__str__(self), __repr__(self)
Returns a formatted summary of the course as a string.
The format to use is: cid(credits): cname
Output
str Formatted summary of the course.
__eq__(self, other)
Determines if two objects are equal. For instances of this class, we will define equality when the
course id of one object is the same as the course id of the other object. You can assume at least
one of the objects is a Couse object.
Input (excluding self)
any other The object to check for equality against a Course object.
Output
bool True if other is a Course object with the same course id, False otherwise.
Section 2: The Catalog class
Stores a collection of Course objects and their capacity as a dictionary, accessible by their ids.
Attributes
Type Name Description
dict courseOfferings Stores courses with the id as the key and a tuple (Course, capacity)
as the value.
Methods
Type Name Description
str addCourse(self, cid, cname, credits, capacity) Adds a course with the given
information.
str removeCourse(self, cid) Removes a course with the given id.
addCourse(self, cid, cname, credits, capacity)
Creates a Course object with the parameters and stores it as a value in courseOfferings.
Inputs (excluding self)
str cid The id of the course to add.
str cname The name of the course to add
int credits The number of credits the course is worth.
int capacity The maximum number of students allowed to register in the course
Output
str “Course added successfully”
str “Course already added” if course is already in courseOfferings.
removeCourse(self, cid)
Removes a course with the given id.
Input (excluding self)
str cid The id of the course to remove.
Output
str “Course removed successfully”
str“Course not found” if a course with the given id is not in the dictionary.
Stores a collection of Course objects and their capacity as a dictionary, accessible by their ids.
Attributes
Type Name Description
dict courseOfferings Stores courses with the id as the key and a tuple (Course, capacity)
as the value.
Methods
Type Name Description
str addCourse(self, cid, cname, credits, capacity) Adds a course with the given
information.
str removeCourse(self, cid) Removes a course with the given id.
addCourse(self, cid, cname, credits, capacity)
Creates a Course object with the parameters and stores it as a value in courseOfferings.
Inputs (excluding self)
str cid The id of the course to add.
str cname The name of the course to add
int credits The number of credits the course is worth.
int capacity The maximum number of students allowed to register in the course
Output
str “Course added successfully”
str “Course already added” if course is already in courseOfferings.
removeCourse(self, cid)
Removes a course with the given id.
Input (excluding self)
str cid The id of the course to remove.
Output
str “Course removed successfully”
str“Course not found” if a course with the given id is not in the dictionary.
Expert Solution

Step 1
Python Code
import random
class Course:
def __init__(self, cid, cname, credits):
self.cid = cid
self.cname = cname
self.credits = credits
def __str__(self):
return f"{self.cid}({self.credits}) : {self.cname}"
def __repr__(self):
return f"{self.cid}({self.credits}) : {self.cname}"
def __eq__(self, other):
if(other is None):
return False
if(self.cid==other.cid):
return True
else:
return False
def isValid(self):
if(type(self.cid)==str and type(self.cname)==str and type(self.credits)==int):
return True
return False
class Catalog:
def __init__(self):
self.courseOfferings = {}
def addCourse(self, cid, cname, credits):
self.courseOfferings[cid] = Course(cid,cname,credits)
return "Course Added successfully"
def removeCourse(self, cid):
del self.courseOfferings[cid]
return "Course removed successfully"
class Semester:
def __init__(self, sem_num):
self.sem_num = sem_num
self.courses = []
def __str__(self):
if(len(self.courses)==0):
return "No courses"
else:
formattedS = ""
for course in self.courses:
formattedS += str(course)
formattedS += ","
return formattedS
def __repr__(self):
if(len(self.courses)==0):
return "No courses"
else:
formattedS = ""
for course in self.courses:
formattedS += str(course)
formattedS += ","
return formattedS
def addCourse(self, course):
if(not isinstance(course,Course) or not course.isValid()):
return "Invalid Course"
if(course in self.courses):
return "Course already added"
else:
self.courses.append(course)
def dropCourse(self, course):
if(not isinstance(course,Course) or not course.isValid()):
return "Invalid Course"
if(course not in self.courses):
return "No such course"
else:
self.courses.remove(course)
@property
def totalCredits(self):
totalcredit = 0
for course in self.courses:
totalcredit += course.credits
return totalcredit
@property
def isFullTime(self):
if(self.totalCredits>=12):
return True
return False
class Loan:
def __init__(self, amount):
self.loan_id = self.__loanID
self.amount = amount
def __str__(self):
return f"Balance: {self.amount}"
def __repr__(self):
return f"Balance: {self.amount}"
@property
def __loanID(self):
return random.randint(10000,99999)
class Person:
def __init__(self, name, ssn):
self.name = name
self.__ssn = ssn
def __str__(self):
return f"Person({self.name},***-**-{self.get_ssn()[-4:]}"
def __repr__(self):
return f"Person({self.name},***-**-{self.get_ssn()[-4:]}"
def get_ssn(self):
return self.__ssn
def __eq__(self, other):
if(self.get_ssn()==other.get_ssn()):
return True
return False
class Staff(Person):
def __init__(self, name, ssn, supervisor=None):
Person.__init__(self,name,ssn)
self.supervisor = supervisor
def __str__(self):
return f"Staff({self.name},{self.id})"
def __repr__(self):
return f"Staff({self.name},{self.id})"
@property
def id(self):
return "905" + self.name.split()[0][0].lower() + self.name.split()[1][0].lower() + self.get_ssn()[-4:]
@property
def getSupervisor(self):
return self.supervisor
def setSupervisor(self, new_supervisor):
if(type(new_supervisor)!="Staff"):
return None
else:
self.supervisor = new_supervisor
return "Completed"
def applyHold(self, student):
if(not isinstance(student,Student)):
return None
else:
student.hold = True
return "Completed"
def removeHold(self, student):
if(not isinstance(student,Student)):
return None
else:
student.hold = False
return "Completed"
def unenrollStudent(self, student):
if(not isinstance(student,Student)):
return None
else:
student.active = False
return "Completed"
class Student(Person):
def __init__(self, name, ssn, year):
random.seed(1)
Person.__init__(self,name,ssn)
self.year = year
self.semesters = {}
self.hold = False
self.active = True
self.account = self.__createStudentAccount()
def __createStudentAccount(self):
if(not self.active):
return None
return StudentAccount(self)
@property
def id(self):
return self.name.split()[0][0].lower() + self.name.split()[1][0].lower() + self.get_ssn()[-4:]
def registerSemester(self):
if(not self.active or self.hold):
return "Unsuccessful Operation"
else:
self.semesters[str(len(self.semesters)+1)] = Semester(len(self.semesters)+1)
def enrollCourse(self, cid, catalog, semester):
if(not self.active or self.hold):
return "Unsuccessful Operation"
for ccid in catalog.courseOfferings:
if(ccid==cid):
if(catalog.courseOfferings[cid] in self.semesters[str(semester)].courses):
return "Course already enrolled"
self.semesters[str(semester)].courses.append(catalog.courseOfferings[cid])
self.account.balance += ((self.account.ppc)*(catalog.courseOfferings[cid]).credits)
return "Course added successfully"
return "Course not found"
def dropCourse(self, cid, semester):
if(not self.active or self.hold):
return "Unsuccessful Operation"
for course in semester.courses:
if(cid==course.cid):
semester.courses.remove(course)
self.account.balance -= ((self.account.ppc)*course.credits)
return "Course Dropped Successfully"
return "Course not found"
def getLoan(self, amount):
l = Loan(amount)
if(not self.active):
return "Unsuccessful Operation"
if(len(self.semesters)==0):
return "Student is not enrolled in any of semesters yet"
if(len(self.semesters)>0):
if(not self.semesters[str(len(self.semesters))].isFullTime):
return "Not Full-time"
if(l.loan_id in StudentAccount(self).loans):
self.account.loans[l.loan_id] += amount
else:
self.account.loans[l.loan_id] = amount
class StudentAccount:
def __init__(self, student):
self.student = student
self.ppc = 1000
self.balance = 0
self.loans = {}
def __str__(self):
return f"Name: {self.student.name} \n ID: {self.student.id} \n Balance: ${self.balance}"
def __repr__(self):
return f"Name: {self.student.name} \n ID: {self.student.id} \n Balance: ${self.balance}"
def makePayment(self, amount, loan_id=None):
if(loan_id is None):
self.balance -= amount
return self.balance
else:
if(loan_id not in self.loans):
return None
elif(self.loans[loan_id]<amount):
return f"Loan Balance : {self.loans[loan_id]}"
else:
self.balance -= amount
self.loans[loan_id] -= amount
return self.balance
def chargeAccount(self, amount):
self.balance += amount
return self.balance
def createStudent(person):
return Student(person.name,person.get_ssn(),"Freshman")
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
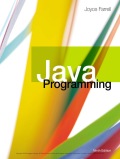
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
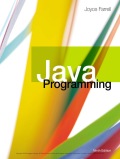
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage