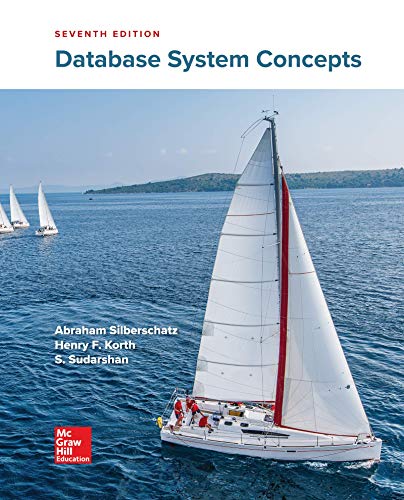
Python
Create a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four peices of information as data attributes: a part number(string), a part description(string), a quantity of the item being purchased(an int), and a price per item(a decimal). Your class should have an __init__ method that initializies the four data attributes. Provide a property for each data attribute. The quantity and price per item should each be non-negative, use validation in the properties for these data attributes to ensure that they remain valid. Provide a calculate_invoice method that returns the invoice amount (that is, multiplies the quantity by the price per item). Demonstrate class Invoice's capabilities.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Part 1: Write a program that tracks employee information. The program should use classes for employee records. Each employee should have a record containing the following information: First Name Last Name Phone Number Address City State Zip Position Job title Payment type The program should be able to allow for the following: the input of new employees, display of existing employees, editing existing employees, deleting employees. To display payment type/salary This must calculate pay for either an hourly paid worker or a salaried worker. Hourly paid workers are paid their hourly pay rate times the number of hours worked. Salaried workers are paid their regular salary plus any bonus they may have earned. The program should declare two structures for the following data: Hourly Paid: HoursWorked HourlyRate Salaried: Salary Bonus The program should ask the user whether he or she is calculating the pay for an hourly paid worker or a salaried worker. Regardless of which the user selects,…arrow_forwardCreate a class called Invoice that a hardware store might use to represent an invoice for an item sold at store. An Invoice should include six data members- a) part number (type string) b) part description (type string) c) quantity of the item being purchased (type int) d) price per item (type int) e) value-added tax (VAT) rate as a decimal (type double) f) Discount rate as a decimal (type double). Your class should have a constructor that initializes the six data members. The constructor should initialize the first four data members with values from parameters and the last two data members to default values of 0.20 per cent and zero respectively. Provide a set and a get functions for each data member. In addition, provide a member function named getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by the price per item and applies the tax and discount amounts), then returns the amount. Have the set data members perform validity checks…arrow_forwardCode should be in Pythonarrow_forward
- in C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forwardPython Code please Point of Sale Write a program that will manage the point of sale in a store. Build the ItemToPurchase class with the following: Attributes item_name (string) item_price (int) item_quantity (int) Default constructor Initializes item's name = "none", item's price = 0, item's quantity = 0 Method print_item_cost() Example of print_item_cost() output:Bottled Water 10 @ $1 = $10 Extend the ItemToPurchase class to contain a new attribute. item_description (string) - Set to "none" in default constructor Implement the following method for the ItemToPurchase class. print_item_description() - Prints item_description attribute for an ItemToPurchase object. Has an ItemToPurchase parameter. Example of print_item_description() output:Bottled Water: Deer Park, 12 oz. Build the ShoppingCart class with the following data attributes and related methods. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Parameterized constructor…arrow_forwardQuestion 31 class Person: def __init__(mysillyobject, name, age): = name mysillyobject.name mysillyobject.age = age def myfunc(abc): print("Hello my age is", abc.age) p1 = Person("John", 36) p1.age = 40 p1.myfunc() O 40 Hello my age is 40 36 Hello my age is 36arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
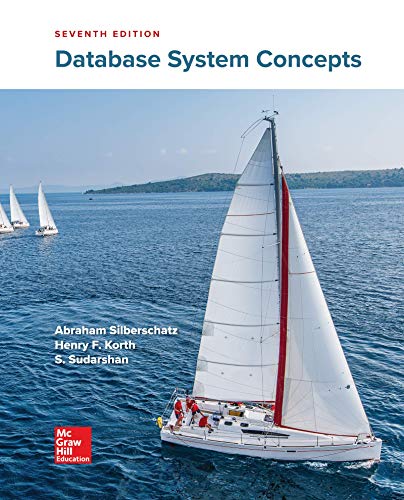
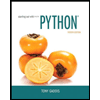
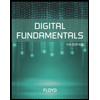
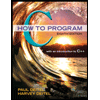
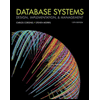
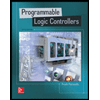