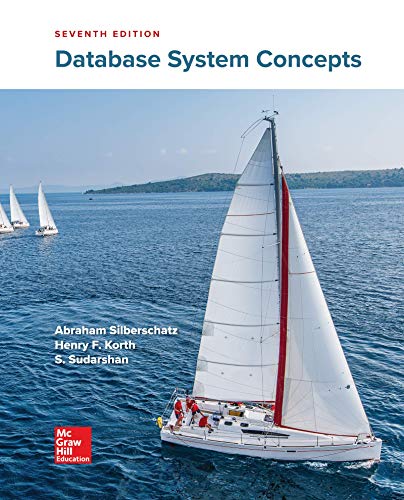
Focus on classes, objects, methods and good programming style.
Program has to be written in basic python.
Your task is to create a BankAccount class.
Class name BankAccount
Attributes __balance float
__pin integer
Methods __init_()
get_pin()
check_pin()
deposit()
withdraw()
get_balance()
The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The
pin should be generated randomly when the account object is created. The initial balance should
be 0.
get_pin()should return the pin.
check_pin(pin) should check the argument against the saved pin and return True if it
matches, False if it does not.
deposit(amount) should receive the amount as the argument, add the amount to the account
and return the new balance.
withraw(amount) should check if the amount can be withdrawn (not more than is in
the account), If so, remove the argument amount from the account and return the new balance if
the transaction was successful. Return False if it was not.
get_balance() should return the current balance.
Finally, write a main() to demo your bank account class. Present a menu offering a few actions and
perform the action the user requests, checking the pin where appropriate.
1. New Account – if selected, create a new account and tell the user the pin.
2. Deposit – if selected, prompt the user to input the pin, check the pin and then, if correct,
prompt for the amount to deposit. Process the deposit and report the new balance
3. Withdraw – if selected, prompt the user to input the pin, check the pin and then, if correct,
prompt for the amount to withdraw. Process the withdrawal and report the new balance or a
message if the transaction was not successful.
4. Check balance – if selected, prompt the user to input the pin, check the pin and then, if correct,
call the get_balance method and report the balance.
All printed output should be done in main(), not the methods.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- PYTHON PROGRAMMING CODE: class Dog:def __init__(self, name, breed, age):self.name = nameself.breed = breedself.age = agedef sit(self):print(self.name.title() + " is now sitting")def roll(self):print(self.name.title() + " rolled over")def bark(self, times):print(self.name, 'says woof ', times, ' times.')dog1 = Dog("bantay", 'aspin', 4)print(dog1.name)print(dog1.breed)print(dog1.age)arrow_forwardJava Programming Please do not change anything in Student class or Course class class John_Smith extends Student{ public John_Smith() { setFirstName("John"); setLastName("Smith"); setEmail("jsmith@jaguar.tamu.edu"); setGender("Male"); setPhoneNumber("(200)00-0000"); setJNumber("J0101459"); } class MyCourse extends Course { public MyCourse { class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String…arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // instance of patient record with each of the 4 parameters taking in a value HeartRates heartRate = new HeartRates("James", "Kill", 1988, 2021); heartRate.DisplayPatientRecord(); // call the method to display The Patient Record } CLASS HeartRATES------------------- class HeartRates { //class attributes private private string _First_Name; private string _Last_Name; private int _Birth_Year; private int _Current_Year; // Constructor which receives private parameters to initialize variables public HeartRates(string First_Name, string Last_Name, int Birth_Year, int Current_Year) { _First_Name = First_Name;…arrow_forward
- 9arrow_forwardJava A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forwardExamine how wireless networks affect developing countries with low GDP per capita. In certain locations, physical connections like local area networks (LANs) may not exist. I'm curious as to the pros and cons of this method.arrow_forward
- Don't use AI.arrow_forwardUse Java Programming Language Create a Loan Account Hierarchy consisting of the following classes: LoanAccount, CarLoan, PrimaryMortgage , UnsecuredLoan, and Address. Each class should be in it's own .java file. The LoanAccount class consists of the following properties: principal- the original amount of the loan. annualInterestRate - the annual interest rate for the loan. It is not static as each loan can have it's own interest rate. months - the number of months in the term of the loan, i.e. the length of the loan. and the following methods: a constructor that takes the three properties as parameters. calculateMonthlyPayment() - takes no parameters and calculates the monthly payment using the same formula as Assignment 1. getters for the three property variables. toString() - displays the information about the principle, annualInterestRate, and months as shown in the example output below. The CarLoan class which is a subclass of the LoanAccount class and consists of the…arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forward
- C# Solve this error using System; namespace RahmanA3P1BasePlusCEmployee { public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; // gross weekly sales private decimal commissionRate; // commission percentage private decimal baseSalary; // base salary per week // six-parameter constructor public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { // implicit call to object constructor occurs here FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; // validates gross sales CommissionRate = commissionRate; // validates commission rate BaseSalary = baseSalary; // validates base…arrow_forwardPortfolio Instructions: You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. When the financial advisor makes a purchase of securities on behalf of a client, a single transaction can include multiple shares of stock or multiple bonds. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double)…arrow_forwardPLEASE help with the following C#.NET using the following class make a driver class which outputs the patient health record Write a driver class (app) that prompts for the person’s data input, instantiates an object of class HeartRates and displays the patient’s information from that object by calling the DisplayPatientRecord, method. essentially make a main method which asks the user to manually enter the data using System;namespace A1Question2{public class HealthProfile{ // attibutes which holds the following valueprivate String _FirstName;private String _LastName;private int _BirthYear;private double _Height;private double _Weigth;private int _CurrentYear;public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int currentYear){_FirstName = firstName;_LastName = lastName;_BirthYear = birthYear;_Height = height;_Weigth = wt;_CurrentYear = currentYear;}public string firstName { get; set; }public string lastName { get; set; }public int birthYear…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
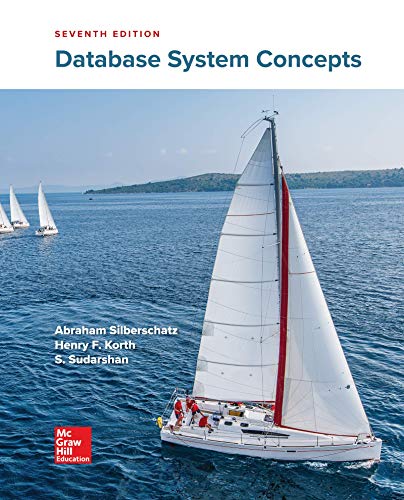
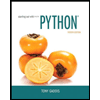
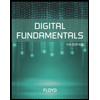
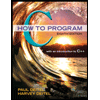
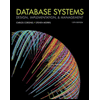
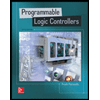