In this assignment, you are provided with working code that does the following: 1. You input a sentence (containing no more than 50 characters). 2. The program will read the sentence and put it into an array of characters. 3. Then, it creates one thread for each character in the sentence. 4. The goal of the program is to capitalize each letter that has an odd index. The given program actually does this, but lacks the synchronization of the threads, so the output is not correct. You will need to provide the synchronization using mutex locks. Specifically, you are to (1) declare the mutex lock, (2) initialize the mutex lock, (3) lock and unlock the mutex lock at an appropriate location that results in the code working as expected, and (4) destroy the mutex lock. Be sure to place the mutex locks so that your program works correctly every time. Do not remove code or functions – you are to add the synchronization pieces only. When compiling using the GNU C compiler, be sure to include the –lpthread flag option. .c file down below PLEASE DO THIS IN C PROGRAMMING #include #include #include #define SIZE 50 char sentence[2000]; int ind = 0; char convertUppercase(char lower) { //Converts lowercase un uppercase if ((lower >96) && (lower <123)) { return (lower -32); } else { return lower; } } void printChar() { //prints the converted sentence printf("The new sentence is [%d]: \t%c\n", ind, sentence[ind]); ind++; } void *convertMessage(void *ptr) { // Function that each threads initiates its execution if (ind %2) { sentence[ind] = convertUppercase(sentence[ind]); } printChar(); return0; } int main() { int i; char buffer[SIZE]; char*p; pthread_t ts[SIZE]; // define up to 50 threads printf("Please enter a phrase (less than 50 characters): "); if (fgets(buffer, sizeof(buffer), stdin) !=NULL) { if ((p =strchr(buffer, '\n')) !=NULL) { *p ='\0'; } } strcpy(sentence, buffer); // copy string to char array printf("The original sentence is: \t%s\n", sentence); // create one thread for each character on the input word for (i =0; i
In this assignment, you are provided with working code that does the following: 1. You input a sentence (containing no more than 50 characters). 2. The program will read the sentence and put it into an array of characters. 3. Then, it creates one thread for each character in the sentence. 4. The goal of the program is to capitalize each letter that has an odd index. The given program actually does this, but lacks the synchronization of the threads, so the output is not correct. You will need to provide the synchronization using mutex locks. Specifically, you are to (1) declare the mutex lock, (2) initialize the mutex lock, (3) lock and unlock the mutex lock at an appropriate location that results in the code working as expected, and (4) destroy the mutex lock. Be sure to place the mutex locks so that your program works correctly every time. Do not remove code or functions – you are to add the synchronization pieces only. When compiling using the GNU C compiler, be sure to include the –lpthread flag option. .c file down below PLEASE DO THIS IN C PROGRAMMING #include #include #include #define SIZE 50 char sentence[2000]; int ind = 0; char convertUppercase(char lower) { //Converts lowercase un uppercase if ((lower >96) && (lower <123)) { return (lower -32); } else { return lower; } } void printChar() { //prints the converted sentence printf("The new sentence is [%d]: \t%c\n", ind, sentence[ind]); ind++; } void *convertMessage(void *ptr) { // Function that each threads initiates its execution if (ind %2) { sentence[ind] = convertUppercase(sentence[ind]); } printChar(); return0; } int main() { int i; char buffer[SIZE]; char*p; pthread_t ts[SIZE]; // define up to 50 threads printf("Please enter a phrase (less than 50 characters): "); if (fgets(buffer, sizeof(buffer), stdin) !=NULL) { if ((p =strchr(buffer, '\n')) !=NULL) { *p ='\0'; } } strcpy(sentence, buffer); // copy string to char array printf("The original sentence is: \t%s\n", sentence); // create one thread for each character on the input word for (i =0; i
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In this assignment, you are provided with working code that does the following:
1. You input a sentence (containing no more than 50 characters).
2. The program will read the sentence and put it into an array of characters.
3. Then, it creates one thread for each character in the sentence.
4. The goal of the program is to capitalize each letter that has an odd index.
The given program actually does this, but lacks the synchronization of the threads, so
the output is not correct. You will need to provide the synchronization using mutex
locks. Specifically, you are to (1) declare the mutex lock, (2) initialize the mutex lock, (3)
lock and unlock the mutex lock at an appropriate location that results in the code
working as expected, and (4) destroy the mutex lock. Be sure to place the mutex locks
so that your program works correctly every time. Do not remove code or functions – you
are to add the synchronization pieces only.
When compiling using the GNU C compiler, be sure to include the –lpthread flag
option.
1. You input a sentence (containing no more than 50 characters).
2. The program will read the sentence and put it into an array of characters.
3. Then, it creates one thread for each character in the sentence.
4. The goal of the program is to capitalize each letter that has an odd index.
The given program actually does this, but lacks the synchronization of the threads, so
the output is not correct. You will need to provide the synchronization using mutex
locks. Specifically, you are to (1) declare the mutex lock, (2) initialize the mutex lock, (3)
lock and unlock the mutex lock at an appropriate location that results in the code
working as expected, and (4) destroy the mutex lock. Be sure to place the mutex locks
so that your program works correctly every time. Do not remove code or functions – you
are to add the synchronization pieces only.
When compiling using the GNU C compiler, be sure to include the –lpthread flag
option.
.c file down below PLEASE DO THIS IN C PROGRAMMING
#include <stdio.h>
#include <string.h>
#include <pthread.h>
#define SIZE 50
char sentence[2000];
int ind = 0;
char convertUppercase(char lower)
{
//Converts lowercase un uppercase
if ((lower >96) && (lower <123))
{
return (lower -32);
}
else
{
return lower;
}
}
void printChar()
{
//prints the converted sentence
printf("The new sentence is [%d]: \t%c\n", ind, sentence[ind]);
ind++;
}
void *convertMessage(void *ptr)
{
// Function that each threads initiates its execution
if (ind %2)
{
sentence[ind] = convertUppercase(sentence[ind]);
}
printChar();
return0;
}
int main()
{
int i;
char buffer[SIZE];
char*p;
pthread_t ts[SIZE]; // define up to 50 threads
printf("Please enter a phrase (less than 50 characters): ");
if (fgets(buffer, sizeof(buffer), stdin) !=NULL)
{
if ((p =strchr(buffer, '\n')) !=NULL)
{
*p ='\0';
}
}
strcpy(sentence, buffer); // copy string to char array
printf("The original sentence is: \t%s\n", sentence);
// create one thread for each character on the input word
for (i =0; i <strlen(buffer) +1; ++i)
{
pthread_create(&ts[i], NULL, convertMessage, NULL);
}
// we wait until all threads finish execution
for (i =0; i <strlen(buffer); i++)
{
pthread_join(ts[i], NULL);
}
printf("\n");
return0;
}
![The problem is that the output should look something like:
$ . /a.out
Please enter a phrase (less than 50 characters): when all else
fails, read the instructions
The original sentence is: when all else fails, read the instructions
The new sentence is [0]:
The new sentence is [1]:
The new sentence is [2]:
The new sentence is [3]:
The new sentence is [4]:
The new sentence is [5]:
The new sentence is [6]:
The new sentence is [7]:
The new sentence is [8]:
The new sentence is [9]:
The new sentence is [10]: 1
The new sentence is [11]: S
The new sentence is [12]: e
The new sentence is [13]:
The new sentence is [14]: f
The new sentence is [15]: A
The new sentence is [16]: i
The new sentence is [17]: L
H
e
N
A
1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F02a699f1-d60c-495e-b821-a86bb0a104cb%2F8e87eba0-52c0-4f94-a8b2-f7fcf7c9e640%2Fdh21qtt_processed.png&w=3840&q=75)
Transcribed Image Text:The problem is that the output should look something like:
$ . /a.out
Please enter a phrase (less than 50 characters): when all else
fails, read the instructions
The original sentence is: when all else fails, read the instructions
The new sentence is [0]:
The new sentence is [1]:
The new sentence is [2]:
The new sentence is [3]:
The new sentence is [4]:
The new sentence is [5]:
The new sentence is [6]:
The new sentence is [7]:
The new sentence is [8]:
The new sentence is [9]:
The new sentence is [10]: 1
The new sentence is [11]: S
The new sentence is [12]: e
The new sentence is [13]:
The new sentence is [14]: f
The new sentence is [15]: A
The new sentence is [16]: i
The new sentence is [17]: L
H
e
N
A
1
![The new sentence is [18] :
The new sentence is [19]:
The new sentence is [20]:
The new sentence is [21]:
The new sentence is [22]:
The new sentence is [23]:
The new sentence is [24]:
The new sentence is [25]:
The new sentence is [26]:
The new sentence is [27]:
The new sentence is [28]:
The new sentence is [29]:
The new sentence is [30]:
The new sentence is [31]:
The new sentence is [32]:
The new sentence is [33]:
The new sentence is [34]:
The new sentence is [35]:
The new sentence is [36]:
The new sentence is [37]:
The new sentence is [38]:
The new sentence is [39]:
The new sentence is [40] :
The new sentence is [41]:
The new sentence is [42]:
R
e
A
d
H
i
N
T
T
n
S](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F02a699f1-d60c-495e-b821-a86bb0a104cb%2F8e87eba0-52c0-4f94-a8b2-f7fcf7c9e640%2Foeyb4o_processed.png&w=3840&q=75)
Transcribed Image Text:The new sentence is [18] :
The new sentence is [19]:
The new sentence is [20]:
The new sentence is [21]:
The new sentence is [22]:
The new sentence is [23]:
The new sentence is [24]:
The new sentence is [25]:
The new sentence is [26]:
The new sentence is [27]:
The new sentence is [28]:
The new sentence is [29]:
The new sentence is [30]:
The new sentence is [31]:
The new sentence is [32]:
The new sentence is [33]:
The new sentence is [34]:
The new sentence is [35]:
The new sentence is [36]:
The new sentence is [37]:
The new sentence is [38]:
The new sentence is [39]:
The new sentence is [40] :
The new sentence is [41]:
The new sentence is [42]:
R
e
A
d
H
i
N
T
T
n
S
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
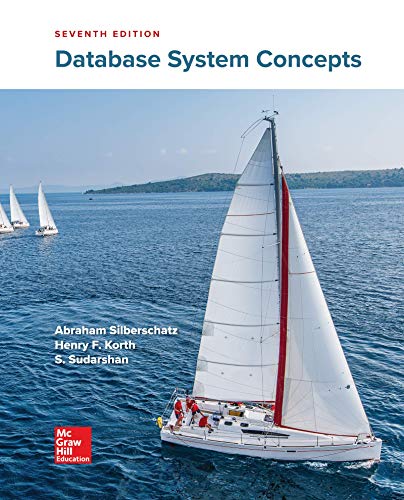
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
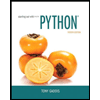
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
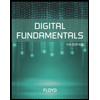
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
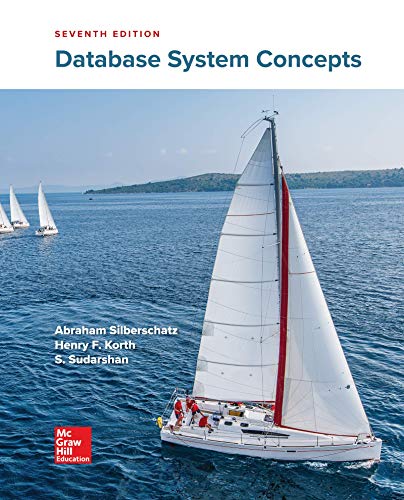
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
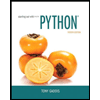
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
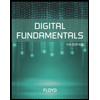
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
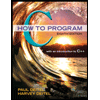
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
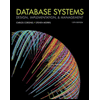
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
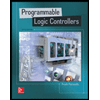
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education