Please use java Write a multithreaded program (using pthread in Linux) that calculates various statistics values for a list of numbers. This program will be passed a series of numbers on the command line and will then create two separate worker threads. One thread will determine the sum of the numbers, the second will determine the product of the numbers of the input. Alternatively, you may create an array of numbers in your program instead of getting input from users. This method may be simpler to implement. We focus our effort on thread creation, not programming capabilities. The variables representing the sum and average values will be stored globally. The worker threads will set these values, and the parent thread will output the values once the workers have exited. For example, suppose your program is passed the integers 22 7 9 62 8 11 3 The program will report The sum value is 122 The average is 17.429
Please use java
Write a multithreaded program (using pthread in Linux) that calculates
various statistics values for a list of numbers. This program will be passed a series of numbers on the command line and will then create two separate worker threads. One thread will determine the sum of the numbers, the second will determine the product of the numbers of the input.
Alternatively, you may create an array of numbers in your program instead of getting input from users. This method may be simpler to implement. We focus our effort on thread creation, not
The variables representing the sum and average values will be stored globally. The worker
threads will set these values, and the parent thread will output the values once the workers
have exited.
For example, suppose your program is passed the integers 22 7 9 62 8 11 3
The program will report
The sum value is 122
The average is 17.429

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

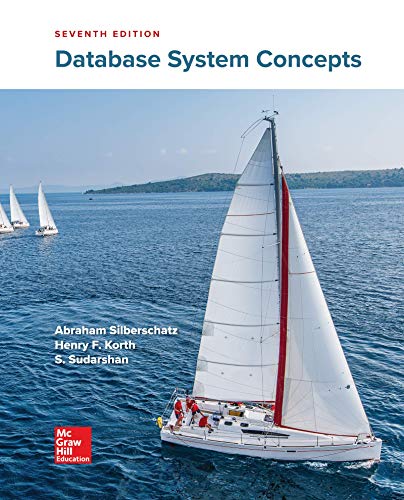
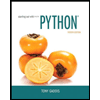
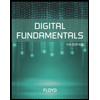
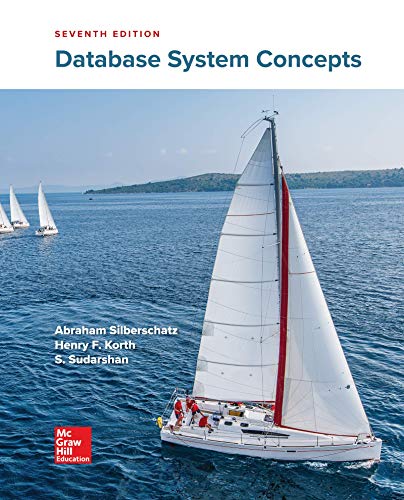
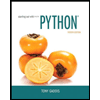
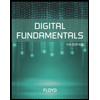
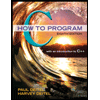
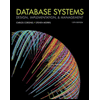
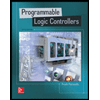