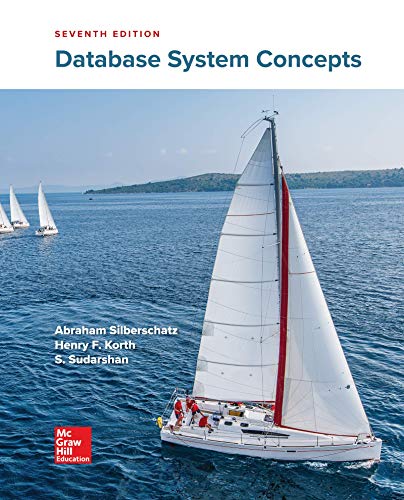
Concept explainers
Write a program that takes in an integer in the range 11-100 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Additionally, output the distance between the starting and ending numbers (ex: 93 - 88 = 5) Note: End with a newline.
Ex: If the input is:
93
the output is:
93 92 91 90 89 88 5
Ex: If the input is:
11
the output is:
11 0
Ex: If the input is:
9
or any value not between 11 and 100 (inclusive), the output is:
Input must be 11-100
For coding simplicity, follow each output number by a space, even the last one.
Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, …, 99), as that approach would be cumbersome for larger ranges.
Hint: To practice incremental development, start by writing code that just outputs the countdown until matching digits and submit to get most of the points. Then, update your code to output the distance.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Write a program that takes in an integer in the range 11-100 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Ex: If the input is: 93 the output is: 93 92 91 90 89 88 Ex: If the input is: 11 the output is: 11 Ex: If the input is: 9 or any value not between 11 and 100 (inclusive), the output is: Input must be 11-100 Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, ..., 99), as that approach would be cumbersome for larger ranges.arrow_forwardStatistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the average (using integer division) and max. A negative integer ends the input and is not included in the statistics. Ex: When the input is: 15 20 0 5 -1 the output is: 10 20 Assume that at least one non-negative integer is input.arrow_forwardDesign a program that computes pay for employees. Allow a user to continuously input employees’ names until an appropriate sentinel value is entered. Also input each employee’s hourly wage and hours worked. Compute each employee’s gross pay (hours times rate), withholding tax percentage (based on Table 6-4), withholding tax amount, and net pay (gross pay minus withholding tax). Display all the results for each employee. After the last employee has been entered, display the sum of all the hours worked, the total gross payroll, the total withholding for all employees, and the total net payroll. You will need to submit a flowchart and pseudocode for this assignment.arrow_forward
- Mad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Write a program that takes a string and an integer as input, and outputs a sentence using the input values as shown in the example below. The program repeats until the input string is quit and disregards the integer input that follows. Ex: If the input is: apples 5 shoes 2 quit 0 the output is: Eating 5 apples a day keeps the doctor away. Eating 2 shoes a day keeps the doctor away.arrow_forwardFor this question, you will write a program that prompts the user to enter an integer value between 1 and 1000 (exclusive). Your program should then print out a sequence of numbers between the given value and 1 (inclusive) following the pattern below:For a given number ai, If ai is even, then ai+1 = floor(ai / 2) If ai is odd, then ai+1 = 3ai + 1 Your program should stop printing numbers once the sequence reaches the value of 1. If the user enters a number that is not between 1 and 1000 (exclusive), print "Invalid input." and prompt the user for a number again. This sequence is referred to as the Collatz sequence. The Collatz Conjecture states that this sequence will eventually reach the value of 1 for any starting number, a fact that has not yet been proven by modern mathematics! Note: floor(x) means that x should be rounded down to the nearest integer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
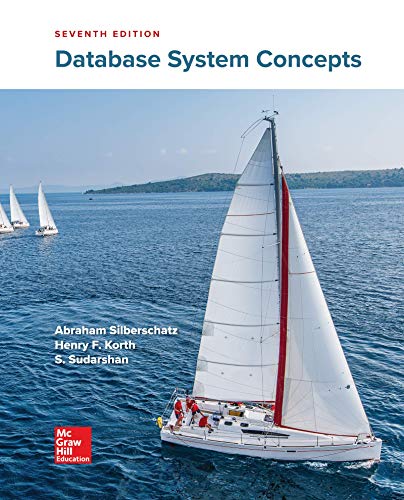
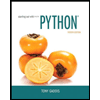
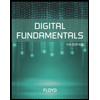
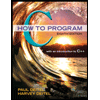
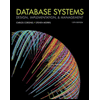
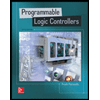