In this exercise, you will design various classes and write a program to computerize the billing system of a hospital. a. Design the class doctorType, inherited from the class personType, with an additional data member to store a doctor’s speciality. Add appropriate constructors and member func- tions to initialize, access, and manipulate the data members. b. Design the class billType with data members to store a patient’s ID and a patient’s hospital charges, such as pharmacy charges for medicine, doctor’s fee, and room charges. Add appropriate construc- tors and member functions to initialize, access, and manipulate the data members. c. Design the class patientType, inherited from the class personType, with additional data members to store a patient’s ID, age, date of birth, attending physician’s name, the date when the patient was admitted in the hospital, and the date when the patient was discharged from the hospital. (Use the class dateType to store the date of birth, admit date, discharge date, and the class doctorType to store the attending physician’s name.) Add appropriate constructors and member functions to initialize, access, and manipulate the data members. d. Write a program to test your classes. Class personType is pictured b
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In this exercise, you will design various classes and write a program to computerize the billing system of a hospital.
a. Design the class doctorType, inherited from the class personType, with an additional data member to store a doctor’s speciality. Add appropriate constructors and member func- tions to initialize, access, and manipulate the data members.
b. Design the class billType with data members to store a patient’s ID and a patient’s hospital charges, such as pharmacy charges for medicine, doctor’s fee, and room charges. Add appropriate construc- tors and member functions to initialize, access, and manipulate the data members.
c. Design the class patientType, inherited from the class personType, with additional data members to store a patient’s ID, age, date of birth, attending physician’s name, the date when the patient was admitted in the hospital, and the date when the patient was discharged from the hospital. (Use the class dateType to store the date of birth, admit date, discharge date, and the class doctorType to store the attending physician’s name.) Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
d. Write a program to test your classes.
Class personType is pictured below
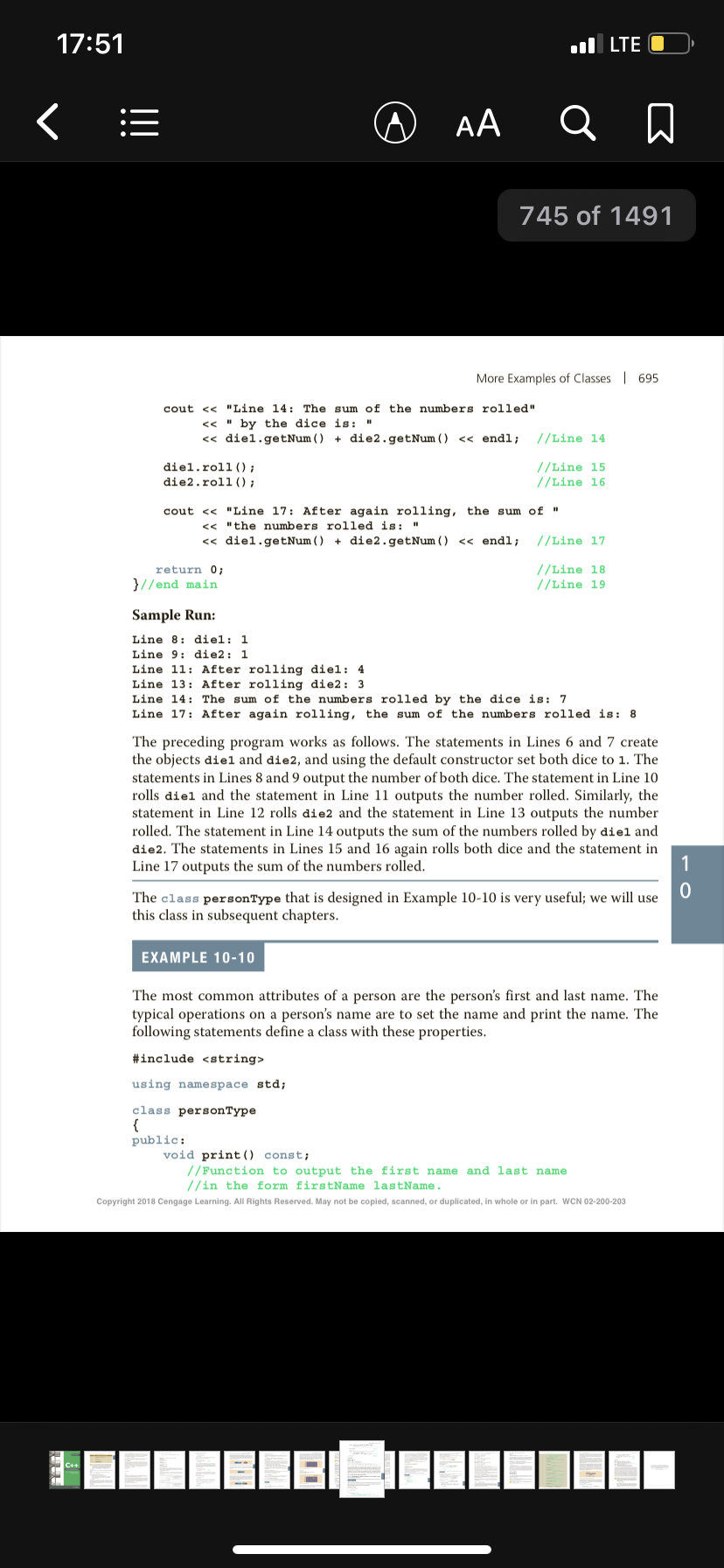
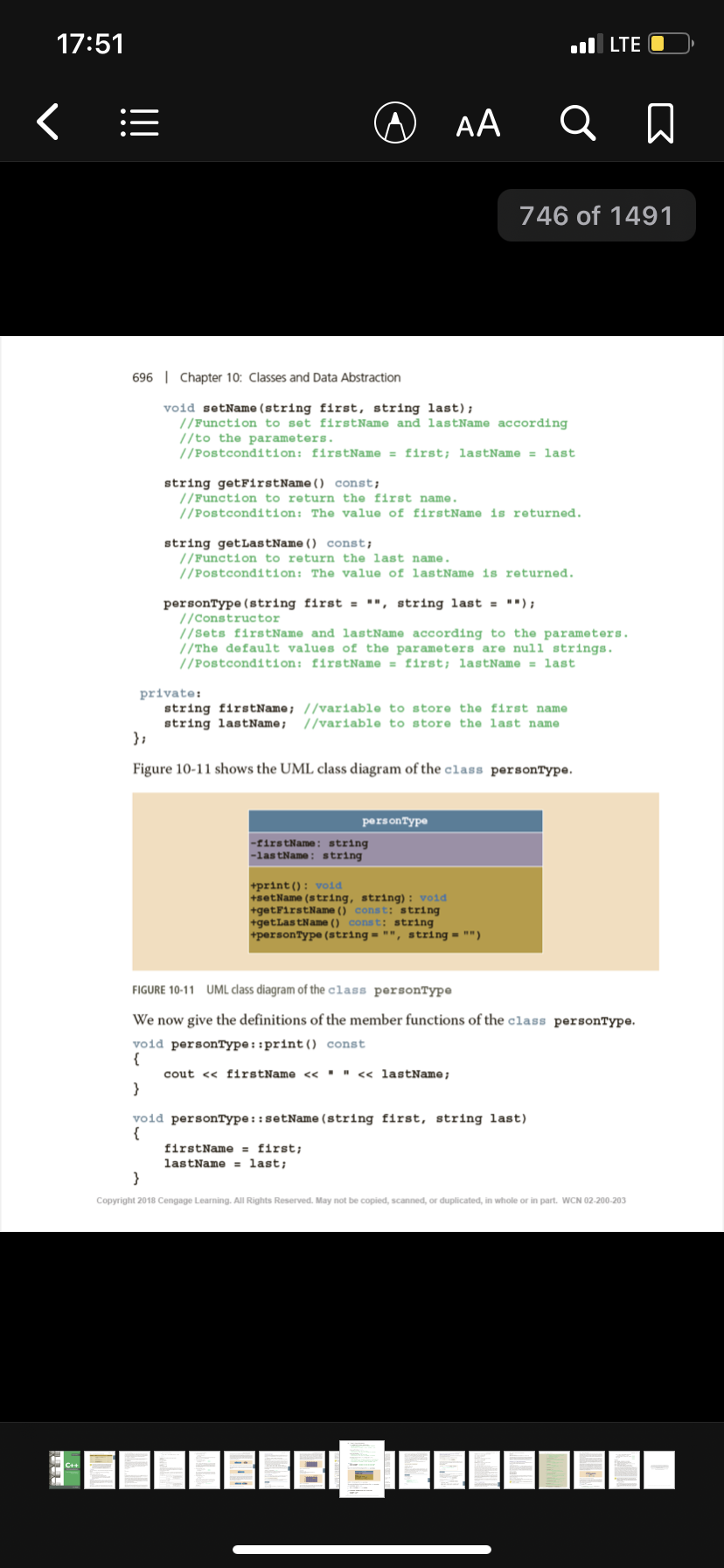

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

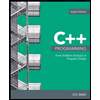
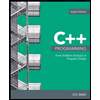